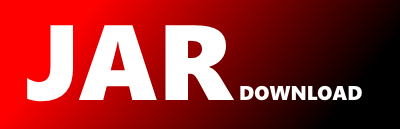
org.kuali.common.util.CollectionUtils Maven / Gradle / Ivy
/**
* Copyright 2010-2012 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util;
import java.io.File;
import java.io.IOException;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import org.apache.commons.io.IOUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.util.Assert;
public class CollectionUtils {
/**
* Prefix the strings passed in with their position in the list (left padded with zero's). The padding is the number of digits in the
* size of the list. A list with 100 elements will return strings prefixed with 000, 001, etc.
*/
public static final List getSequencedStrings(List strings, int initialSequenceNumber) {
List sequencedStrings = new ArrayList();
int size = strings.size();
int length = new Integer(size).toString().length();
String prefix = StringUtils.repeat("0", length);
for (String string : strings) {
String sequence = StringUtils.right(prefix + (initialSequenceNumber++), length);
String sequencedString = sequence + "-" + string;
sequencedStrings.add(sequencedString);
}
return sequencedStrings;
}
/**
* Prefix the strings passed in with their position in the list (left padded with zero's). The padding is the number of digits in the
* size of the list. A list with 100 elements will return strings prefixed with 000, 001, etc.
*/
public static final List getSequencedStrings(List strings) {
return getSequencedStrings(strings, 0);
}
/**
* Return a new List
containing the unique set of strings from strings
*/
public static final List getUniqueStrings(List strings) {
List unique = new ArrayList();
for (String string : strings) {
if (!unique.contains(string)) {
unique.add(string);
}
}
return unique;
}
public static final List getUniqueFiles(List files) {
List strings = new ArrayList();
for (File file : files) {
strings.add(LocationUtils.getCanonicalPath(file));
}
List uniqueStrings = getUniqueStrings(strings);
List uniqueFiles = new ArrayList();
for (String uniqueString : uniqueStrings) {
uniqueFiles.add(new File(uniqueString));
}
return uniqueFiles;
}
public static final List getLines(String s) {
if (s == null) {
return Collections. emptyList();
}
try {
return IOUtils.readLines(new StringReader(s));
} catch (IOException e) {
throw new IllegalStateException(e);
}
}
/**
* Return a new list containing the unique set of strings contained in both lists
*/
public static final List combineStringsUniquely(List list1, List list2) {
List newList = getUniqueStrings(list1);
for (String element : list2) {
if (!newList.contains(element)) {
newList.add(element);
}
}
return newList;
}
protected static final T getNewInstance(Class c) {
try {
return c.newInstance();
} catch (IllegalAccessException e) {
throw new IllegalArgumentException(e);
} catch (InstantiationException e) {
throw new IllegalArgumentException(e);
}
}
/**
* Create a new list containing new instances of c
*/
public static final List getNewList(Class c, int size) {
List list = new ArrayList();
for (int i = 0; i < size; i++) {
T element = getNewInstance(c);
list.add(element);
}
return list;
}
/**
* Return a list containing only the elements where the corresponding index in the includes
list is true
.
* includes
and list
must be the same size.
*/
public static final List getList(List includes, List list) {
Assert.isTrue(includes.size() == list.size());
List included = new ArrayList();
for (int i = 0; i < includes.size(); i++) {
if (includes.get(i)) {
included.add(list.get(i));
}
}
return included;
}
/**
* Return a combined list where required
is always the first element in the list
*/
public static final List combine(T required, List optional) {
Assert.notNull(required);
if (optional == null) {
return Collections.singletonList(required);
} else {
List combined = new ArrayList();
// Always insert required as the first element in the list
combined.add(required);
// Add the other elements
for (T element : optional) {
combined.add(element);
}
return combined;
}
}
/**
* If o==null
return an empty list otherwise return a singleton list.
*/
public static final List toEmptyList(T o) {
if (o == null) {
return Collections. emptyList();
} else {
return Collections.singletonList(o);
}
}
/**
* If list==null
return an empty list otherwise return list
*/
public static final List toEmptyList(List list) {
if (list == null) {
return Collections. emptyList();
} else {
return list;
}
}
public static final List toNullIfEmpty(List list) {
if (isEmpty(list)) {
return null;
} else {
return list;
}
}
public static final Collection toNullIfEmpty(Collection c) {
if (isEmpty(c)) {
return null;
} else {
return c;
}
}
public static final List getPreFilledList(int size, T value) {
if (value == null || size < 1) {
return Collections. emptyList();
} else {
List list = new ArrayList(size);
for (int i = 0; i < size; i++) {
list.add(value);
}
return list;
}
}
public static final String getSpaceSeparatedString(List> list) {
list = toEmptyList(list);
StringBuilder sb = new StringBuilder();
for (int i = 0; i < list.size(); i++) {
if (i != 0) {
sb.append(" ");
}
sb.append(list.get(i).toString());
}
return sb.toString();
}
public static final Object[] toObjectArray(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy