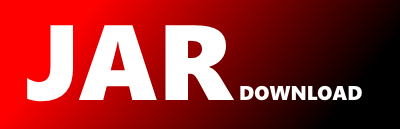
org.kuali.common.util.ListUtils Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util;
import static org.kuali.common.util.base.Precondition.checkNotNull;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import org.apache.commons.lang3.StringUtils;
import com.google.common.base.Optional;
import com.google.common.collect.ImmutableList;
public class ListUtils {
public static boolean equalElements(List list1, List list2) {
checkNotNull(list1, "list1");
checkNotNull(list2, "list2");
if (list1.size() != list2.size()) {
return false;
}
for (int i = 0; i < list1.size(); i++) {
if (!list1.get(i).equals(list2.get(i))) {
return false;
}
}
return true;
}
public static List prefix(String prefix, List list) {
return prefix(prefix, Optional. absent(), list);
}
public static List prefix(String prefix, String separator, List list) {
return prefix(prefix, Optional.of(separator), list);
}
private static List prefix(String prefix, Optional separator, List list) {
Assert.noBlanks(prefix);
Assert.noNulls(list, separator);
Assert.noBlanks(separator);
List newList = newArrayList();
String separatorValue = separator.isPresent() ? separator.get() : "";
for (String element : list) {
String value = prefix + separatorValue + element;
newList.add(value);
}
return ImmutableList.copyOf(newList);
}
public static List newArrayList() {
return newArrayList(new ArrayList());
}
public static List newArrayList(T element) {
Assert.noNulls(element);
List list = newArrayList();
list.add(element);
return list;
}
public static List newArrayList(List list) {
return newArrayList(list, false);
}
public static List newImmutableArrayList(List list) {
return newArrayList(list, true);
}
public static List newArrayList(List list, boolean immutable) {
Assert.noNulls(list);
if (immutable) {
return Collections.unmodifiableList(new ArrayList(list));
} else {
return new ArrayList(list);
}
}
/**
* This method guarantees 4 things:
*
* 1 - The list
is not null.
* 2 - The list
is not empty. (size() > 0)
* 3 - The list
does not contain null
.
* 4 - Every element in the list
is the exact same runtime type.
*/
public static void assertUniformRuntimeType(List> list) {
Assert.noNulls(list);
Assert.isTrue(list.size() > 0, "list is empty");
Assert.isFalse(list.contains(null), "list contains null");
Class> previous = list.get(0).getClass();
for (int i = 1; i < list.size(); i++) {
Class> current = list.get(i).getClass();
Assert.isTrue(current == previous, "non-uniform runtime types at index " + i);
previous = current;
}
}
public static boolean equals(List one, List two) {
return equals(one, two, false);
}
public static boolean equalsIgnoreCase(List one, List two) {
return equals(one, two, true);
}
protected static boolean equals(List one, List two, boolean ignoreCase) {
// Nulls not allowed
Assert.noNulls(one, two);
// If the sizes are different they are not equal
if (one.size() != two.size()) {
return false;
}
// The sizes are the same, just pick one
int size = one.size();
// Iterate over both lists comparing each value for equality
for (int i = 0; i < size; i++) {
if (!equal(one.get(i), two.get(i), ignoreCase)) {
return false;
}
}
// All values in both lists match
return true;
}
protected static boolean equal(String one, String two, boolean ignoreCase) {
if (ignoreCase) {
return StringUtils.equalsIgnoreCase(one, two);
} else {
return StringUtils.equals(one, two);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy