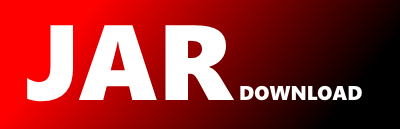
org.kuali.common.util.ObjectUtils Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util;
import static com.google.common.base.Preconditions.checkState;
import static org.kuali.common.util.base.Precondition.checkNotNull;
import java.util.Objects;
import org.kuali.common.util.equality.Equatable;
public class ObjectUtils {
/**
*
* This method returns true
if the toString()
methods on both objects return matching strings AND both objects are the exact same runtime type.
*
*
*
* Returns true
immediately if instance==other
(ie they are the same object).
*
*
*
* Returns false
immediately if other==null
or is a different runtime type than instance
.
*
*
*
* If neither one is null
, and both are the exact same runtime type, then compare the toString()
methods
*
*
* @param instance
* The object other
is being compared to.
* @param other
* The object being examined for equality with instance
.
*
* @throws NullPointerException
* If instance is null
or instance.toString()
returns null
*/
public static boolean equalsByToString(Object instance, Object other) {
checkNotNull(instance, "instance");
// They are the same object
if (instance == other) {
return true;
}
if (notEqual(instance, other)) {
return false; // Don't bother comparing the toString() methods
} else {
return instance.toString().equals(other.toString());
}
}
/**
*
* Return true if instance
is definitely not equal to other
.
*
*
*
* If other
is null OR a different runtime type than instance
, return true
*
*
* @param instance
* The object other
is being compared to.
* @param other
* The object being examined for equality with instance
.
*
* @throws NullPointerException
* If instance is null
*/
public static boolean notEqual(Object instance, Object other) {
checkNotNull(instance, "instance");
if (other == null) {
return true;
} else {
return instance.getClass() != other.getClass();
}
}
public static boolean equal(T instance, Object other) {
checkNotNull(instance, "instance");
if (instance == other) {
return true;
} else if (notEqual(instance, other)) {
return false;
} else {
return equal(instance, (Equatable) other);
}
}
private static boolean equal(Equatable instance, Equatable other) {
Object[] values1 = instance.getEqualityValues();
Object[] values2 = other.getEqualityValues();
checkState(values1.length == values2.length, "value arrays must be the same. values1.length=%s values2.length=%s", values1.length, values2.length);
for (int i = 0; i < values1.length; i++) {
if (!Objects.equals(values1[i], values2[i])) {
return false;
}
}
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy