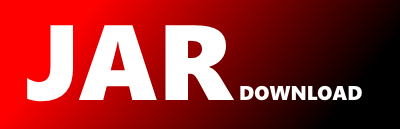
org.kuali.common.util.base.string.SplitterFunction Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.base.string;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.collect.Sets.newHashSet;
import static org.kuali.common.util.base.Precondition.checkNotBlank;
import static org.kuali.common.util.base.Precondition.checkNotNull;
import java.util.List;
import java.util.Set;
import com.google.common.base.Function;
import com.google.common.base.Splitter;
import com.google.common.collect.ImmutableSet;
/**
*
* Convert a set of delimited strings into an immutable set of individual elements.
*
*
* A set containing these two strings:
*
*
* java.class.path
* java.class.version
*
*
* Converts to a set containing these four strings:
*
*
* java
* java.class
* java.class.path
* java.class.version
*
*/
public final class SplitterFunction implements Function, Set> {
public SplitterFunction() {
this(".");
}
public SplitterFunction(String separator) {
this.separator = checkNotNull(separator, "separator");
this.splitter = Splitter.on(separator);
}
private final Splitter splitter;
private final String separator;
/**
*
* Convert a set of delimited strings into an immutable set of individual elements.
*
*
* A set containing these two strings:
*
*
* java.class.path
* java.class.version
*
*
* Converts to a set containing these four strings:
*
*
* java
* java.class
* java.class.path
* java.class.version
*
*
* @throws IllegalArgumentException
* If splitting the individual strings into elements produces blank tokens
* @throws NullPointerException
* If strings is null or contains null elements
*/
@Override
public Set apply(Set strings) {
checkNotNull(strings, "strings");
Set set = newHashSet();
for (String string : strings) {
set.addAll(apply(string));
}
return ImmutableSet.copyOf(set);
}
/**
* Convert a delimited string into a set of individual elements.
*
*
* java.class.path -> java
* java.class
* java.class.path
*
*
* @throws IllegalArgumentException
* If splitting the string into elements produces blank tokens
*/
protected Set apply(String string) {
checkNotNull(string, "string");
// Split the key into tokens
List tokens = splitter.splitToList(string);
// Setup some storage for the strings we are creating
Set strings = newHashSet();
// Allocate a string builder
StringBuilder sb = new StringBuilder();
// Iterate over the tokens to create unique string elements
for (int i = 0; i < tokens.size(); i++) {
// append the separator unless this is the first loop iteration
sb = (i != 0) ? sb.append(separator) : sb;
// Extract the token, checking to make sure it isn't blank
String token = checkNotBlank(tokens.get(i), "token");
// Append the current token to create a new element
String element = sb.append(token).toString();
// Make sure it was actually added
// TODO Don't think checkArgument() here is necessary since checkNotBlank() prevents token from being the empty string
checkArgument(strings.add(element), "%s is a duplicate token -> [%s]", element, string);
}
// Return what we've got
return strings;
}
public String getSeparator() {
return separator;
}
public Splitter getSplitter() {
return splitter;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy