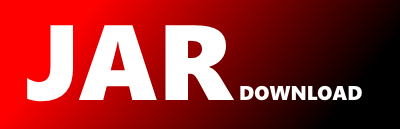
org.kuali.common.util.enc.DefaultEncryptionService Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.enc;
import java.util.List;
import java.util.Properties;
import java.util.SortedSet;
import org.jasypt.util.text.TextEncryptor;
import org.kuali.common.util.Assert;
import org.kuali.common.util.PropertyUtils;
import com.google.common.collect.Sets;
/**
* @deprecated
*/
@Deprecated
public final class DefaultEncryptionService implements EncryptionService {
private final TextEncryptor encryptor;
public DefaultEncryptionService(TextEncryptor encryptor) {
Assert.noNulls(encryptor);
this.encryptor = encryptor;
}
@Override
public String encrypt(String text) {
if (EncUtils.isEncrypted(text)) {
return text; // It's already encrypted, just return it
}
Assert.notEncrypted(text);
String encryptedText = encryptor.encrypt(text);
return EncUtils.wrap(encryptedText);
}
@Override
public String decrypt(String text) {
if (!EncUtils.isEncrypted(text)) {
return text; // It's not encrypted, just return it
}
Assert.encrypted(text);
String unwrapped = EncUtils.unwrap(text);
return encryptor.decrypt(unwrapped);
}
/**
* Decrypt any encrypted property values.
*/
@Override
public void decrypt(Properties properties) {
List keys = PropertyUtils.getEncryptedKeys(properties);
for (String key : keys) {
String encrypted = properties.getProperty(key);
String decrypted = decrypt(encrypted);
properties.setProperty(key, decrypted);
}
}
/**
* Encrypt any property values that are not already encrypted.
*/
@Override
public void encrypt(Properties properties) {
SortedSet allKeys = Sets.newTreeSet(properties.stringPropertyNames());
SortedSet encKeys = Sets.newTreeSet(PropertyUtils.getEncryptedKeys(properties));
SortedSet keys = Sets.newTreeSet(Sets.difference(allKeys, encKeys));
for (String key : keys) {
String plaintext = properties.getProperty(key);
String encrypted = encrypt(plaintext);
properties.setProperty(key, encrypted);
}
}
public TextEncryptor getEncryptor() {
return encryptor;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy