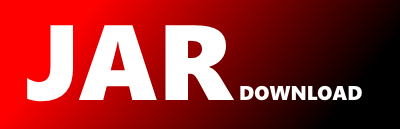
org.kuali.common.util.enc.EncryptionContext Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.enc;
import org.kuali.common.util.Assert;
import org.kuali.common.util.nullify.NullUtils;
import com.google.common.base.Optional;
/**
* @deprecated Use EncContext instead
*/
@Deprecated
public final class EncryptionContext {
public static final EncryptionContext DEFAULT = new EncryptionContext.Builder().build();
private final boolean enabled;
private final boolean passwordRequired;
private final boolean removePasswordSystemProperty;
private final Optional password;
private final Optional passwordKey;
private final EncStrength strength;
public static class Builder {
private boolean passwordRequired = false;
private boolean removePasswordSystemProperty = true;
private Optional password = Optional.absent();
private Optional passwordKey = Optional.absent();
private EncStrength strength = EncStrength.BASIC;
// For convenience only. enabled == password.isPresent()
private boolean enabled = false;
public Builder removePasswordSystemProperty(boolean removePasswordSystemProperty) {
this.removePasswordSystemProperty = removePasswordSystemProperty;
return this;
}
public Builder passwordRequired(boolean passwordRequired) {
this.passwordRequired = passwordRequired;
return this;
}
public Builder password(String password) {
this.password = NullUtils.toAbsent(password);
return this;
}
public Builder passwordKey(String passwordKey) {
this.passwordKey = NullUtils.toAbsent(passwordKey);
return this;
}
public Builder strength(EncStrength strength) {
this.strength = strength;
return this;
}
public EncryptionContext build() {
Assert.noNulls(password, passwordKey, strength, enabled);
this.enabled = password.isPresent();
if (passwordRequired) {
Assert.isTrue(password.isPresent(), "Encryption password is required");
}
if (password.isPresent()) {
Assert.noBlanks(password.get());
}
return new EncryptionContext(this);
}
}
private EncryptionContext(Builder builder) {
this.enabled = builder.enabled;
this.passwordKey = builder.passwordKey;
this.passwordRequired = builder.passwordRequired;
this.strength = builder.strength;
this.removePasswordSystemProperty = builder.removePasswordSystemProperty;
this.password = builder.password;
}
public boolean isEnabled() {
return enabled;
}
public Optional getPassword() {
return password;
}
public EncStrength getStrength() {
return strength;
}
public boolean isPasswordRequired() {
return passwordRequired;
}
public Optional getPasswordKey() {
return passwordKey;
}
public boolean isRemovePasswordSystemProperty() {
return removePasswordSystemProperty;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy