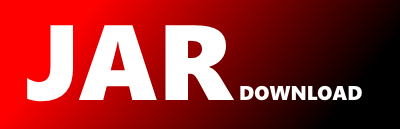
org.kuali.common.util.encrypt.openssl.OpenSSLEncryptedContext Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.encrypt.openssl;
import static com.google.common.collect.Lists.newArrayList;
import static org.kuali.common.util.base.Precondition.checkNotNull;
import java.util.List;
import com.google.common.collect.ImmutableList;
public final class OpenSSLEncryptedContext {
private final ImmutableList salt;
private final ImmutableList key;
private final ImmutableList initVector;
private OpenSSLEncryptedContext(Builder builder) {
this.salt = ImmutableList.copyOf(builder.salt);
this.key = ImmutableList.copyOf(builder.key);
this.initVector = ImmutableList.copyOf(builder.initVector);
}
public static Builder builder() {
return new Builder();
}
public static class Builder implements org.apache.commons.lang3.builder.Builder {
private List salt = newArrayList();
private List key = newArrayList();
private List initVector = newArrayList();
public Builder withSalt(List salt) {
this.salt = salt;
return this;
}
public Builder withKey(List key) {
this.key = key;
return this;
}
public Builder withInitVector(List initVector) {
this.initVector = initVector;
return this;
}
@Override
public OpenSSLEncryptedContext build() {
return validate(new OpenSSLEncryptedContext(this));
}
private static OpenSSLEncryptedContext validate(OpenSSLEncryptedContext instance) {
checkNotNull(instance.salt, "salt");
checkNotNull(instance.key, "key");
checkNotNull(instance.initVector, "initVector");
return instance;
}
}
public List getSalt() {
return salt;
}
public List getKey() {
return key;
}
public List getInitVector() {
return initVector;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy