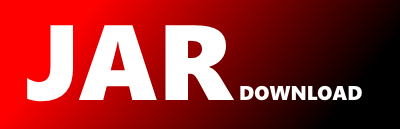
org.kuali.common.util.encrypt.provider.AbstractEncryptionContextProvider Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.encrypt.provider;
import static com.google.common.base.Optional.absent;
import static com.google.common.base.Optional.fromNullable;
import static java.lang.String.format;
import static org.apache.commons.lang3.StringUtils.trimToNull;
import static org.kuali.common.util.base.Precondition.checkNotBlank;
import static org.kuali.common.util.encrypt.EncryptionStrength.DEFAULT_ENCRYPTION_STRENGTH;
import static org.kuali.common.util.log.Loggers.newLogger;
import org.kuali.common.util.encrypt.EncryptionContext;
import org.kuali.common.util.encrypt.EncryptionStrength;
import org.slf4j.Logger;
import com.google.common.base.Optional;
public abstract class AbstractEncryptionContextProvider implements EncryptionContextProvider {
private static final Logger logger = newLogger();
public AbstractEncryptionContextProvider(String passwordKey, String strengthKey) {
this.passwordKey = checkNotBlank(passwordKey, "passwordKey");
this.strengthKey = checkNotBlank(strengthKey, "strengthKey");
}
private final String passwordKey;
private final String strengthKey;
@Override
public Optional getEncryptionContext() {
Optional password = getOptionalString(passwordKey);
if (!password.isPresent()) {
logger.debug(format("[%s, key=%s] encryption password not found", this.getClass().getSimpleName(), passwordKey));
return absent();
} else {
EncryptionStrength strength = getEncryptionStrength();
logger.debug(format("[%s, key=%s %s] encryption password located", this.getClass().getSimpleName(), passwordKey, strength));
return Optional.of(new EncryptionContext(password.get(), strength));
}
}
protected Optional getOptionalString(String key) {
return fromNullable(trimToNull(getValueFromSource(key)));
}
protected abstract String getValueFromSource(String key);
protected EncryptionStrength getEncryptionStrength() {
Optional optional = getOptionalString(strengthKey);
if (optional.isPresent()) {
return EncryptionStrength.valueOf(optional.get().toUpperCase());
} else {
return DEFAULT_ENCRYPTION_STRENGTH;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy