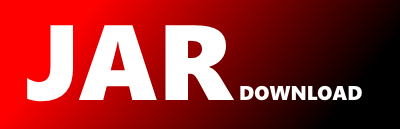
org.kuali.common.util.encrypt.provider.DefaultEncryptionContextProviderChain Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.encrypt.provider;
import static com.google.common.base.Optional.absent;
import static com.google.common.collect.Lists.newArrayList;
import java.util.List;
import org.kuali.common.util.encrypt.EncryptionContext;
import com.google.common.base.Optional;
import com.google.common.collect.ImmutableList;
public final class DefaultEncryptionContextProviderChain implements EncryptionContextProvider {
private static final String ENC_PASSWORD_KEY = "enc.password";
private static final String ENC_STRENGTH_KEY = "enc.strength";
// Need this key for backwards compatibility. "enc.password" contains a different password for some existing Maven processes
private static final String ENC_MAVEN_ALTERNATE_PASSWORD_KEY = "enc.pwd";
public DefaultEncryptionContextProviderChain() {
List providers = newArrayList();
providers.add(new SystemPropertiesEncryptionContextProvider(ENC_PASSWORD_KEY, ENC_STRENGTH_KEY));
providers.add(new EnvironmentVariableEncryptionContextProvider(toEnvKey(ENC_PASSWORD_KEY), toEnvKey(ENC_STRENGTH_KEY)));
providers.add(new FileEncryptionContextProvider());
providers.add(new MavenEncryptionContextProvider(ENC_MAVEN_ALTERNATE_PASSWORD_KEY, ENC_STRENGTH_KEY));
providers.add(new MavenEncryptionContextProvider(ENC_PASSWORD_KEY, ENC_STRENGTH_KEY));
this.providers = ImmutableList.copyOf(providers);
}
public DefaultEncryptionContextProviderChain(EncryptionContextProvider... providers) {
this(ImmutableList.copyOf(providers));
}
public DefaultEncryptionContextProviderChain(List providers) {
this.providers = ImmutableList.copyOf(providers);
}
private final List providers;
public List getProviders() {
return providers;
}
@Override
public Optional getEncryptionContext() {
for (EncryptionContextProvider provider : providers) {
Optional context = provider.getEncryptionContext();
if (context.isPresent()) {
return context;
}
}
return absent();
}
protected static String toEnvKey(String key) {
return key.replace('.', '_').toUpperCase();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy