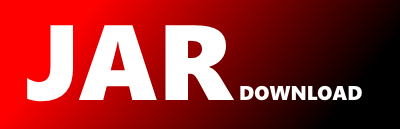
org.kuali.common.util.encrypt.provider.FileEncryptionContextProvider Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.encrypt.provider;
import static com.google.common.base.Optional.absent;
import static com.google.common.base.Optional.fromNullable;
import static com.google.common.base.Preconditions.checkState;
import static java.lang.String.format;
import static org.apache.commons.lang3.StringUtils.isBlank;
import static org.apache.commons.lang3.StringUtils.trimToNull;
import static org.kuali.common.util.base.Exceptions.illegalState;
import static org.kuali.common.util.base.Precondition.checkNotBlank;
import static org.kuali.common.util.encrypt.EncryptionStrength.DEFAULT_ENCRYPTION_STRENGTH;
import static org.kuali.common.util.encrypt.provider.DefaultEncryptionContextProviderChain.toEnvKey;
import static org.kuali.common.util.log.Loggers.newLogger;
import java.io.File;
import java.io.IOException;
import java.util.List;
import org.apache.commons.io.FileUtils;
import org.kuali.common.util.encrypt.EncryptionContext;
import org.kuali.common.util.encrypt.EncryptionStrength;
import org.kuali.common.util.file.CanonicalFile;
import org.slf4j.Logger;
import com.google.common.base.Optional;
import com.google.common.collect.ImmutableList;
public final class FileEncryptionContextProvider implements EncryptionContextProvider {
private static final Logger logger = newLogger();
private static final String ENC_PASSWORD_FILE_SYS_KEY = "enc.password.file";
private static final String ENC_PASSWORD_FILE_ENV_KEY = toEnvKey(ENC_PASSWORD_FILE_SYS_KEY);
private static final File DEFAULT_ENC_PASSWORD_FILE = new CanonicalFile(System.getProperty("user.home") + "/.enc/password");
@Override
public Optional getEncryptionContext() {
File file = getEncPasswordFile();
if (!file.exists()) {
logger.debug(format("[%s] does not exist", file));
return absent();
}
List lines = readLines(file);
String password = getPassword(lines);
EncryptionStrength strength = getStrength(lines);
logger.debug(format("[%s, %s] encryption password located", file, strength));
return Optional.of(new EncryptionContext(password, strength));
}
protected EncryptionStrength getStrength(List lines) {
if (lines.size() < 2) {
return DEFAULT_ENCRYPTION_STRENGTH;
}
String value = lines.get(1);
if (isBlank(value)) {
return DEFAULT_ENCRYPTION_STRENGTH;
}
return EncryptionStrength.valueOf(value.toUpperCase().trim());
}
protected String getPassword(List lines) {
checkState(lines.size() > 0, "[%s] must contain at least one line");
String password = trimToNull(lines.get(0));
checkNotBlank(password, "password");
return password;
}
protected static List readLines(File file) {
try {
return ImmutableList.copyOf(FileUtils.readLines(file));
} catch (IOException e) {
throw illegalState(e);
}
}
protected static File getEncPasswordFile() {
Optional sys = getOptional(System.getProperty(ENC_PASSWORD_FILE_SYS_KEY));
if (sys.isPresent()) {
File file = new CanonicalFile(sys.get());
logger.debug(format("encryption system property: %s=[%s]", ENC_PASSWORD_FILE_SYS_KEY, file.getPath()));
return file;
}
Optional env = getOptional(System.getenv(ENC_PASSWORD_FILE_ENV_KEY));
if (env.isPresent()) {
File file = new CanonicalFile(env.get());
logger.debug(format("encryption environment variable: %s=[%s]", ENC_PASSWORD_FILE_ENV_KEY, file.getPath()));
return file;
}
return DEFAULT_ENC_PASSWORD_FILE;
}
protected static Optional getOptional(String value) {
return fromNullable(trimToNull(value));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy