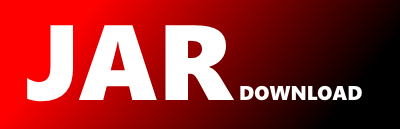
org.kuali.common.util.execute.impl.ShowEnvExec Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.execute.impl;
import static java.lang.String.format;
import static org.apache.commons.lang3.StringUtils.isBlank;
import static org.kuali.common.util.base.Precondition.checkNotBlank;
import static org.kuali.common.util.base.Precondition.checkNotNull;
import static org.kuali.common.util.log.Loggers.newLogger;
import java.nio.charset.Charset;
import java.util.List;
import java.util.Locale;
import org.kuali.common.util.execute.Executable;
import org.kuali.common.util.file.CanonicalFile;
import org.slf4j.Logger;
import com.google.common.collect.ImmutableList;
public final class ShowEnvExec implements Executable {
private static final Logger logger = newLogger();
private static final Object[] EMPTY_OBJECT_ARRAY = {};
public ShowEnvExec() {
this(false);
}
public ShowEnvExec(boolean skip) {
this.skip = skip;
}
private final boolean skip;
@Override
public void execute() {
if (skip) {
return;
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy