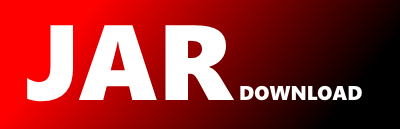
org.kuali.common.util.log.LoggerContext Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.log;
import static com.google.common.base.Preconditions.checkNotNull;
import java.util.List;
import org.slf4j.Logger;
import com.google.common.collect.ImmutableList;
public final class LoggerContext {
private final LoggerLevel level;
private final String msg;
private final ImmutableList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy