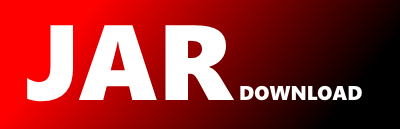
org.kuali.common.util.metainf.spring.RiceXmlConfig Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.metainf.spring;
import java.io.File;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.Map;
import com.google.common.base.Optional;
import com.google.common.collect.Lists;
import org.apache.commons.lang3.StringUtils;
import org.kuali.common.util.metainf.model.*;
import org.kuali.common.util.metainf.service.MetaInfUtils;
import org.kuali.common.util.nullify.NullUtils;
import org.kuali.common.util.project.ProjectUtils;
import org.kuali.common.util.project.model.Build;
import org.kuali.common.util.project.model.Project;
import org.kuali.common.util.project.spring.AutowiredProjectConfig;
import org.kuali.common.util.spring.SpringUtils;
import org.kuali.common.util.spring.env.EnvironmentService;
import org.kuali.common.util.spring.service.SpringServiceConfig;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Import;
import com.google.common.collect.Maps;
/**
* TODO Should not be a class called {@code RiceXmlConfig} down here in kuali-util. Create a rice-util and move this there? Main issue preventing this from living in the rice-xml
* module itself is that it gets tricky having software used very early in the build lifecycle reside in the same project that makes use of it.
*/
@Configuration
@Import({ AutowiredProjectConfig.class, MetaInfExecutableConfig.class, SpringServiceConfig.class })
public class RiceXmlConfig implements MetaInfContextsConfig {
private static final boolean DEFAULT_GENERATE_RELATIVE_PATHS = true;
private static final String RELATIVE_KEY = MetaInfUtils.PROPERTY_PREFIX + ".xml.relative";
private static final String PREFIX = "xml";
// This is used in the rice-xml module to help locate the correct .resources file containing the XML to ingest
public static final String INGEST_FILENAME = "ingest";
@Autowired
EnvironmentService env;
@Autowired
Project project;
@Autowired
Build build;
@Override
@Bean
public List metaInfContexts() {
List metaInfContexts = new ArrayList();
List includeStrings = Lists.newArrayList("/initial-xml/", "/upgrades/*/");
for (String includeString : includeStrings) {
for (MetaInfDataType type : getTypes()) {
List contexts = getMetaInfContexts(MetaInfGroup.OTHER, includeString, type);
metaInfContexts.addAll(contexts);
}
}
return metaInfContexts;
}
protected List getMetaInfContexts(MetaInfGroup group, String includeString, MetaInfDataType type) {
List metaInfContexts = Lists.newArrayList();
String includesKey = MetaInfConfigUtils.getIncludesKey(group, PREFIX);
String excludesKey = MetaInfConfigUtils.getExcludesKey(group, PREFIX);
File scanDir = build.getOutputDir();
String encoding = build.getEncoding();
Comparator comparator = getComparator();
boolean relativePaths = env.getBoolean(RELATIVE_KEY, DEFAULT_GENERATE_RELATIVE_PATHS);
List qualifiers = MetaInfUtils.getQualifiers(scanDir, project, Lists.newArrayList(includeString), Lists.newArrayList());
for (String qualifier : qualifiers) {
File outputFile = MetaInfUtils.getOutputFile(project, build, Optional.of(qualifier), Optional. absent(), Optional.of(type), INGEST_FILENAME);
Map defaultIncludes = getDefaultIncludes(project, qualifier, type);
Map defaultExcludes = getDefaultExcludes();
List includes = SpringUtils.getNoneSensitiveListFromCSV(env, includesKey, defaultIncludes.get(group));
List excludes = SpringUtils.getNoneSensitiveListFromCSV(env, excludesKey, defaultExcludes.get(group));
MetaInfContext context = new MetaInfContext.Builder(outputFile, encoding, scanDir).comparator(comparator).includes(includes).excludes(excludes).relativePaths(relativePaths).build();
metaInfContexts.add(context);
}
return metaInfContexts;
}
protected List getTypes() {
return Lists.newArrayList(MetaInfDataType.BOOTSTRAP, MetaInfDataType.DEMO, MetaInfDataType.TEST);
}
protected Comparator getComparator() {
return new MetaInfResourcePathComparator();
}
protected Map getDefaultIncludes(Project project, String qualifier, MetaInfDataType type) {
String resourcePath = ProjectUtils.getResourcePath(project.getGroupId(), project.getArtifactId());
Map map = Maps.newHashMap();
List paths = Lists.newArrayList(resourcePath, qualifier, type.name().toLowerCase(), "**/*.xml");
map.put(MetaInfGroup.OTHER, StringUtils.join(paths, "/"));
return map;
}
protected Map getDefaultExcludes() {
Map map = Maps.newHashMap();
map.put(MetaInfGroup.OTHER, NullUtils.NONE);
return map;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy