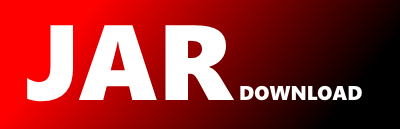
org.kuali.common.util.spring.env.BasicEnvironmentService Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.spring.env;
import java.io.File;
import java.util.Properties;
import org.kuali.common.util.Assert;
import org.kuali.common.util.ModeUtils;
import org.kuali.common.util.spring.env.model.EnvironmentServiceContext;
/**
*
* By default, an exception is thrown if a value cannot be located (unless a default value has been supplied).
*
*
*
* By default, an exception is thrown if any placeholders cannot be resolved in any string values.
*
*
*
* By default, string values are resolved before being returned
*
*
*
* By default, environment variables are automatically checked if a normal property value cannot be found.
*
* For example, given the key db.vendor
the service will also automatically check env.DB_VENDOR
*
*/
public final class BasicEnvironmentService implements EnvironmentService {
private final EnvironmentServiceContext context;
/**
* Uses system properties / environment variables to resolve values
*/
public BasicEnvironmentService() {
this(new EnvironmentServiceContext.Builder().build());
}
/**
* Uses properties
to resolve values
*/
public BasicEnvironmentService(Properties properties) {
this(new EnvironmentServiceContext.Builder().env(properties).build());
}
/**
* Uses context
to resolve values
*/
public BasicEnvironmentService(EnvironmentServiceContext context) {
Assert.noNulls(context);
this.context = context;
}
@Override
public boolean containsProperty(String key) {
Assert.noBlanks(key);
return context.getEnv().containsProperty(key);
}
@Override
public T getProperty(EnvContext context) {
// If context is null, we have issues
Assert.noNulls(context);
// Extract a value from Spring's Environment abstraction
T springValue = getSpringValue(context.getKey(), context.getType());
// If that value is null, use whatever default value they gave us (this might also be null)
T returnValue = (springValue == null) ? context.getDefaultValue() : springValue;
// If we could not locate a value, we may need to error out
if (returnValue == null) {
ModeUtils.validate(this.context.getMissingPropertyMode(), getMissingPropertyMessage(context.getKey()));
}
// Return the value we've located
return returnValue;
}
@Override
public T getProperty(String key, Class type, T provided) {
return getProperty(EnvContext.newCtx(key, type, provided));
}
@Override
public T getProperty(String key, Class type) {
return getProperty(EnvContext.newCtx(key, type, null));
}
protected String getMissingPropertyMessage(String key) {
if (context.isCheckEnvironmentVariables()) {
String envKey = EnvUtils.getEnvironmentVariableKey(key);
return "No value for [" + key + "] or [" + envKey + "]";
} else {
return "No value for [" + key + "]";
}
}
protected T getSpringValue(String key, Class type) {
T value = context.getEnv().getProperty(key, type);
if (value == null && context.isCheckEnvironmentVariables()) {
String envKey = EnvUtils.getEnvironmentVariableKey(key);
return context.getEnv().getProperty(envKey, type);
} else {
return value;
}
}
protected Class getSpringValueAsClass(String key, Class type) {
Class value = context.getEnv().getPropertyAsClass(key, type);
if (value == null && context.isCheckEnvironmentVariables()) {
String envKey = EnvUtils.getEnvironmentVariableKey(key);
return context.getEnv().getPropertyAsClass(envKey, type);
} else {
return value;
}
}
@Override
public Class getClass(String key, Class type) {
return getClass(key, type, null);
}
@Override
public Class getClass(String key, Class type, Class defaultValue) {
Class springValue = getSpringValueAsClass(key, type);
Class returnValue = (springValue == null) ? defaultValue : springValue;
// If we could not locate a value, we may need to error out
if (returnValue == null) {
ModeUtils.validate(context.getMissingPropertyMode(), getMissingPropertyMessage(key));
}
// Return what we've got
return returnValue;
}
@Override
public String getString(String key) {
return getString(key, null);
}
@Override
public String getString(String key, String defaultValue) {
String string = getProperty(EnvContext.newString(key, defaultValue));
if (context.isResolveStrings()) {
return context.getEnv().resolveRequiredPlaceholders(string);
} else {
return string;
}
}
@Override
public Boolean getBoolean(String key) {
return getBoolean(key, null);
}
@Override
public Boolean getBoolean(String key, Boolean defaultValue) {
return getProperty(EnvContext.newBoolean(key, defaultValue));
}
@Override
public File getFile(String key) {
return getFile(key, null);
}
@Override
public File getFile(String key, File defaultValue) {
return getProperty(EnvContext.newFile(key, defaultValue));
}
@Override
public Integer getInteger(String key, Integer defaultValue) {
return getProperty(EnvContext.newInteger(key, defaultValue));
}
@Override
public Integer getInteger(String key) {
return getInteger(key, null);
}
public EnvironmentServiceContext getContext() {
return context;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy