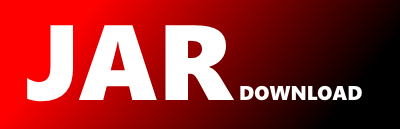
org.kuali.common.util.spring.env.SysEnvPropertySource Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.spring.env;
import static com.google.common.base.Preconditions.checkNotNull;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import org.kuali.common.util.PropertyUtils;
import org.kuali.common.util.log.LoggerUtils;
import org.slf4j.Logger;
import org.springframework.core.env.MapPropertySource;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Maps;
/**
*
* When {@code getProperty(name)} is called, {@code name} is checked. If no value is found, {@code name} is then converted into several aliases representing a few different ways it
* might be represented as an environment variable. All of the aliases are then also checked.
*
*
*
* foo.barBaz -> env.FOO_BAR_BAZ
* FOO_BAR_BAZ
* env.foo_bar_baz
* foo_bar_baz
*
*/
public class SysEnvPropertySource extends MapPropertySource {
private static final Map> ALIAS_CACHE = Maps.newConcurrentMap();
private static final String GLOBAL_PROPERTIES_PROPERTY_SOURCE_NAME = "systemPropertiesAndEnvironmentVariables";
private static final Logger logger = LoggerUtils.make();
public SysEnvPropertySource() {
this(GLOBAL_PROPERTIES_PROPERTY_SOURCE_NAME, PropertyUtils.getGlobalProperties());
}
public SysEnvPropertySource(String name, Properties source) {
this(name, convert(source));
}
public SysEnvPropertySource(String name, Map source) {
super(name, source);
}
/**
* {@inheritDoc}
*/
@Override
public Object getProperty(String name) {
checkNotNull(name, "'name' cannot be null");
Object value = super.getProperty(name);
if (value != null) {
return value;
} else {
Set aliases = getAliases(name);
return getProperty(aliases, name);
}
}
protected Object getProperty(Set aliases, String original) {
for (String alias : aliases) {
Object value = super.getProperty(alias);
if (value != null) {
logger.debug(String.format("PropertySource [%s] does not contain '%s', but found equivalent '%s'", this.getName(), original, alias));
return value;
}
}
return null;
}
/**
*
* foo.barBaz -> env.FOO_BAR_BAZ
* FOO_BAR_BAZ
* env.foo_bar_baz
* foo_bar_baz
*
*/
protected ImmutableSet getAliases(String name) {
ImmutableSet aliases = ALIAS_CACHE.get(name);
if (aliases == null) {
// foo.barBaz -> env.FOO_BAR_BAZ
String env1 = EnvUtils.getEnvironmentVariableKey(name);
// foo.barBaz -> FOO_BAR_BAZ
String env2 = EnvUtils.toUnderscore(name).toUpperCase();
// foo.barBaz -> env.foo_bar_baz
String env3 = env1.toLowerCase();
// foo.barBaz -> foo_bar_baz
String env4 = env2.toLowerCase();
aliases = ImmutableSet.of(env1, env2, env3, env4);
ALIAS_CACHE.put(name, aliases);
}
return aliases;
}
protected static Map convert(Properties properties) {
Map map = Maps.newHashMap();
for (String key : properties.stringPropertyNames()) {
map.put(key, properties.getProperty(key));
}
return map;
}
public void clearAliasCache() {
ALIAS_CACHE.clear();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy