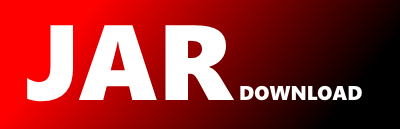
org.kuali.common.util.spring.service.SpringContext Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.spring.service;
import java.util.List;
import java.util.Map;
import org.kuali.common.util.CollectionUtils;
public class SpringContext {
public SpringContext(Map beans, Class> annotatedClass) {
this(beans, CollectionUtils.asList(annotatedClass), (String) null);
}
public SpringContext(Map beans, Class> annotatedClass, String activeProfile) {
this(beans, CollectionUtils.asList(annotatedClass), null, CollectionUtils.toEmptyList(activeProfile));
}
public SpringContext(Map beans, List> annotatedClasses, String activeProfile) {
this(beans, annotatedClasses, null, CollectionUtils.toEmptyList(activeProfile));
}
public SpringContext(PropertySourceContext propertySourceContext) {
this((Class>) null, propertySourceContext);
}
public SpringContext() {
this((Class>) null);
}
public SpringContext(Class> annotatedClass) {
this(CollectionUtils.asList(annotatedClass));
}
public SpringContext(Class> annotatedClass, PropertySourceContext propertySourceContext) {
this(CollectionUtils.asList(annotatedClass), propertySourceContext);
}
public SpringContext(List> annotatedClasses) {
this(annotatedClasses, null);
}
public SpringContext(List> annotatedClasses, PropertySourceContext propertySourceContext) {
this(null, annotatedClasses, propertySourceContext);
}
public SpringContext(Map contextBeans, List> annotatedClasses, PropertySourceContext propertySourceContext) {
this(contextBeans, annotatedClasses, propertySourceContext, null);
}
public SpringContext(Map contextBeans, List> annotatedClasses, PropertySourceContext propertySourceContext, List activeProfiles) {
this.contextBeans = contextBeans;
this.annotatedClasses = annotatedClasses;
this.propertySourceContext = propertySourceContext;
this.activeProfiles = activeProfiles;
}
String id;
String displayName;
List locations;
List> annotatedClasses;
Map contextBeans;
PropertySourceContext propertySourceContext;
List activeProfiles;
List defaultProfiles;
@Deprecated
List beanNames;
@Deprecated
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy