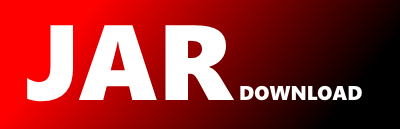
org.kuali.common.util.tree.Trees Maven / Gradle / Ivy
/**
* Copyright 2010-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.common.util.tree;
import static com.google.common.collect.Lists.newArrayList;
import java.util.List;
import org.apache.commons.lang3.StringUtils;
import com.google.common.base.Function;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Lists;
public class Trees {
public static List> getLeaves(List> nodes) {
List> leaves = newArrayList();
for (Node node : nodes) {
leaves.addAll(getLeaves(node));
}
return leaves;
}
public static List> getLeaves(Node root) {
List> leaves = newArrayList();
List> nodes = breadthFirst(root);
for (Node node : nodes) {
if (node.isLeaf()) {
leaves.add(node);
}
}
return leaves;
}
public static List breadthFirstElements(Node node) {
return Lists.transform(breadthFirst(node), new NodeElementFunction());
}
public static List> breadthFirst(List> nodes) {
List> list = newArrayList();
for (Node node : nodes) {
list.addAll(breadthFirst(node));
}
return list;
}
public static List> breadthFirst(Node node) {
NodeTraverser nt = NodeTraverser.create();
Iterable> itr = nt.breadthFirstTraversal(node);
return newArrayList(itr);
}
public static List> postOrder(Node node) {
NodeTraverser nt = NodeTraverser.create();
Iterable> itr = nt.postOrderTraversal(node);
return newArrayList(itr);
}
public static List> preOrder(Node node) {
NodeTraverser nt = NodeTraverser.create();
Iterable> itr = nt.preOrderTraversal(node);
return newArrayList(itr);
}
public static String html(String title, Node node) {
Function, String> converter = NodeStringFunction.create();
return html(title, ImmutableList.of(node), converter);
}
public static String html(String title, Node node, Function, String> converter) {
return html(title, ImmutableList.of(node), converter);
}
public static String html(String title, List> nodes) {
Function, String> converter = NodeStringFunction.create();
return html(title, nodes, converter);
}
public static String html(String title, List> nodes, Function, String> converter) {
StringBuilder sb = new StringBuilder();
sb.append("\n");
sb.append(" " + title + " \n");
sb.append(" \n");
sb.append(" \n");
for (Node node : nodes) {
sb.append(html(node, 3, converter));
}
sb.append(" \n");
sb.append(" \n");
sb.append("
\n");
return sb.toString();
}
public static String html(Node node) {
Function, String> converter = NodeStringFunction.create();
return html(node, 0, converter);
}
public static String html(Node node, Function, String> converter) {
return html(node, 0, converter);
}
public static String html(Node node, int indent, Function, String> converter) {
StringBuilder sb = new StringBuilder();
String prefix = StringUtils.repeat(" ", indent);
sb.append(prefix + "\n");
sb.append(prefix + " \n");
sb.append(prefix + " " + converter.apply(node) + " \n");
List> children = node.getChildren();
if (!children.isEmpty()) {
sb.append(prefix + " \n");
for (Node child : children) {
sb.append(html(child, indent + 3, converter));
}
sb.append(prefix + " \n");
}
sb.append(prefix + " \n");
sb.append(prefix + "
\n");
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy