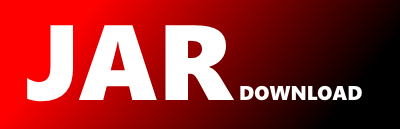
org.odmg.DSet Maven / Gradle / Ivy
Show all versions of db-ojb Show documentation
package org.odmg;
/**
* The ODMG Set collection interface.
* A DSet
object is an unordered collection that does not support
* multiple elements with the same value. An implementation typically is very
* efficient at determining whether the collection contains a particular value.
*
* All of the operations defined by the JavaSoft Set
* interface are supported by an ODMG implementation of DSet
,
* the exception UnsupportedOperationException
is not thrown when a
* call is made to any of the Set
methods.
* @author David Jordan (as Java Editor of the Object Data Management Group)
* @version ODMG 3.0
*/
// * @see java.lang.UnsupportedOperationException
public interface DSet extends DCollection, java.util.Set
{
/**
* Create a new DSet
object that is the set union of this
* DSet
object and the set referenced by otherSet
.
* @param otherSet The other set to be used in the union operation.
* @return A newly created DSet
instance that contains the union of the two sets.
*/
public DSet union(DSet otherSet);
/**
* Create a new DSet
object that is the set intersection of this
* DSet
object and the set referenced by otherSet
.
* @param otherSet The other set to be used in the intersection operation.
* @return A newly created DSet
instance that contains the
* intersection of the two sets.
*/
public DSet intersection(DSet otherSet);
/**
* Create a new DSet
object that contains the elements of this
* collection minus the elements in otherSet
.
* @param otherSet A set containing elements that should not be in the result set.
* @return A newly created DSet
instance that contains the elements
* of this set minus those elements in otherSet
.
*/
public DSet difference(DSet otherSet);
/**
* Determine whether this set is a subset of the set referenced by otherSet
.
* @param otherSet Another set.
* @return True if this set is a subset of the set referenced by otherSet
,
* otherwise false.
*/
public boolean subsetOf(DSet otherSet);
/**
* Determine whether this set is a proper subset of the set referenced by
* otherSet
.
* @param otherSet Another set.
* @return True if this set is a proper subset of the set referenced by
* otherSet
, otherwise false.
*/
public boolean properSubsetOf(DSet otherSet);
/**
* Determine whether this set is a superset of the set referenced by otherSet
.
* @param otherSet Another set.
* @return True if this set is a superset of the set referenced by otherSet
,
* otherwise false.
*/
public boolean supersetOf(DSet otherSet);
/**
* Determine whether this set is a proper superset of the set referenced by
* otherSet
.
* @param otherSet Another set.
* @return True if this set is a proper superset of the set referenced by
* otherSet
, otherwise false.
*/
public boolean properSupersetOf(DSet otherSet);
}