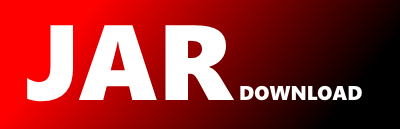
org.kuali.student.admin.ui.mojo.AdminUiInquiryViewBeanWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kscontractdoc-maven-plugin Show documentation
Show all versions of kscontractdoc-maven-plugin Show documentation
Generates documentation for Kuali Student service contracts
/**
* Copyright 2004-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.student.admin.ui.mojo;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintStream;
import java.util.List;
import java.util.Stack;
import org.kuali.student.contract.model.Lookup;
import org.kuali.student.contract.model.MessageStructure;
import org.kuali.student.contract.model.Service;
import org.kuali.student.contract.model.ServiceContractModel;
import org.kuali.student.contract.model.ServiceMethod;
import org.kuali.student.contract.model.XmlType;
import org.kuali.student.contract.model.util.ModelFinder;
import org.kuali.student.contract.writer.XmlWriter;
import org.kuali.student.contract.writer.service.GetterSetterNameCalculator;
/**
*
* @author nwright
*/
public class AdminUiInquiryViewBeanWriter {
private ServiceContractModel model;
private ModelFinder finder;
private String directory;
private String rootPackage;
private String servKey;
private Service service;
private XmlType xmlType;
private List methods;
private XmlWriter out;
String fileName;
String fullDirectoryPath;
public AdminUiInquiryViewBeanWriter(ServiceContractModel model,
String directory,
String rootPackage,
String servKey,
XmlType xmlType,
List methods) {
this.model = model;
this.finder = new ModelFinder(model);
this.directory = directory;
this.rootPackage = rootPackage;
this.servKey = servKey;
service = finder.findService(servKey);
this.xmlType = xmlType;
this.methods = methods;
}
/**
* Write out the entire file
*
* @param out
*/
public void write() {
initXmlWriter();
writeBoilerPlate();
writeBean();
}
private void writeBean() {
String infoClass = GetterSetterNameCalculator.calcInitUpper(xmlType.getName());
String serviceClass = GetterSetterNameCalculator.calcInitUpper(service.getName());
String serviceVar = GetterSetterNameCalculator.calcInitLower(service.getName());
String serviceContract = service.getImplProject() + "." + service.getName();
String inquirable = AdminUiInquirableWriter.calcPackage(servKey, rootPackage, xmlType) + "."
+ AdminUiInquirableWriter.calcClassName(servKey, xmlType);
out.println("");
out.incrementIndent();
out.indentPrintln(" ");
out.indentPrintln("");
out.indentPrintln("");
out.indentPrintln("");
out.indentPrintln("");
out.indentPrintln("");
out.indentPrintln("");
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln(" ");
this.writeFields(xmlType, new Stack(), "");
out.indentPrintln("
");
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln("
");
out.indentPrintln(" ");
out.decrementIndent();
out.indentPrintln(" ");
out.indentPrintln("");
out.decrementIndent();
out.indentPrintln("");
}
private void writeFields(XmlType type, Stack parents, String prefix) {
// avoid recursion
if (parents.contains(type)) {
return;
}
parents.push(type);
for (MessageStructure ms : finder.findMessageStructures(type.getName())) {
String fieldName = GetterSetterNameCalculator.calcInitLower(ms.getShortName());
if (!prefix.isEmpty()) {
fieldName = prefix + "." + fieldName;
}
if (ms.getType().equalsIgnoreCase("AttributeInfoList")) {
out.indentPrintln(" ");
continue;
}
if (ms.getType().endsWith("List")) {
out.indentPrintln(" ");
continue;
}
XmlType fieldType = finder.findXmlType(ms.getType());
if (fieldType.getPrimitive().equalsIgnoreCase(XmlType.COMPLEX)) {
// complex sub-types such as rich text
this.writeFields(fieldType, parents, fieldName);
continue;
}
out.indentPrint(" ");
continue;
}
// process lookup
out.println(">");
Lookup lookup = ms.getLookup();
XmlType msType = finder.findXmlType(lookup.getXmlTypeName());
MessageStructure pk = this.getPrimaryKey(ms.getLookup().getXmlTypeName());
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln(" ");
}
parents.pop();
}
public static boolean shouldDoLookup (Lookup lookup) {
if (lookup == null) {
return false;
}
// can't do lookups on things we don't have inquirables on yet
// if (lookup.getXmlTypeName().equals("OrgInfo")) {
// return true;
// }
if (lookup.getXmlTypeName().equals("Principal")) {
return false;
}
if (lookup.getXmlTypeName().equals("Agenda")) {
return false;
}
return true;
}
private MessageStructure getPrimaryKey(String xmlTypeName) {
for (MessageStructure ms : finder.findMessageStructures(xmlTypeName)) {
if (ms.isPrimaryKey()) {
return ms;
}
}
throw new NullPointerException ("could not find primary key for " + xmlTypeName);
}
private void initXmlWriter() {
String infoClass = GetterSetterNameCalculator.calcInitUpper(xmlType.getName());
String serviceClass = GetterSetterNameCalculator.calcInitUpper(service.getName());
String serviceVar = GetterSetterNameCalculator.calcInitLower(service.getName());
String serviceContract = service.getImplProject() + "." + service.getName();
fullDirectoryPath = AdminUiInquirableWriter.calcPackage(servKey, rootPackage, xmlType).replace('.', '/');
fileName = infoClass + "AdminInquiryView.xml";
File dir = new File(this.directory);
if (!dir.exists()) {
if (!dir.mkdirs()) {
throw new IllegalStateException("Could not create directory "
+ this.directory);
}
}
String dirStr = this.directory + "/" + "resources" + "/" + fullDirectoryPath;
File dirFile = new File(dirStr);
if (!dirFile.exists()) {
if (!dirFile.mkdirs()) {
throw new IllegalStateException(
"Could not create directory " + dirStr);
}
}
try {
PrintStream out = new PrintStream(new FileOutputStream(
dirStr + "/" + fileName, false));
this.out = new XmlWriter(out, 0);
} catch (FileNotFoundException ex) {
throw new IllegalStateException(ex);
}
}
private void writeBoilerPlate() {
out.println("");
out.println("");
out.println(" ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy