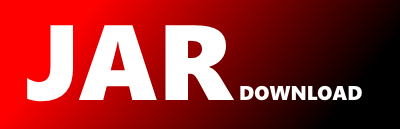
org.apache.torque.mojo.BaseMojo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-impex-plugin Show documentation
Show all versions of maven-impex-plugin Show documentation
Maven plugin for converting database agnostic XML files into platform specific SQL files and for examining proprietary databases via JDBC to generate database agnostic XML files
/**
* Copyright 2004-2012 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.torque.mojo;
import org.apache.maven.execution.MavenSession;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.project.MavenProject;
import org.apache.maven.settings.Settings;
import org.kuali.maven.mojo.MavenLogger;
/**
* Mojo essentials. Contains the "skip" logic that is the de facto standard for
* maven plugins. Contains a number of maven related properties that are common
* to most mojos. Also sets up logging so that if libraries called by a mojo
* issue log statements to Jakarta Commons Logging or Log4j, those log messages
* are shown in maven's output
*/
public abstract class BaseMojo extends AbstractMojo {
public static final String FS = System.getProperty("file.separator");
public static final String SKIP_PACKAGING_TYPE = "pom";
/**
* When true, redirect logging from Log4j and Jakarta Commons Logging to the
* Maven logging system
*
* @parameter expression="${startMavenLogger}" default-value="true"
*/
private boolean startMavenLogger;
/**
* When true
, skip the execution of this mojo
*
* @parameter default-value="false"
*/
private boolean skip;
/**
* Setting this parameter to true
will force the execution of
* this mojo, even if it would get skipped usually.
*
* @parameter expression="${forceMojoExecution}" default-value="false"
* @required
*/
private boolean forceMojoExecution;
/**
* The encoding to use when reading/writing files. If not specified this
* defaults to the platform specific encoding of whatever machine the build
* is running on.
*
* @parameter expression="${encoding}"
* default-value="${project.build.sourceEncoding}"
*/
private String encoding;
/**
* The Maven project this plugin runs in.
*
* @parameter expression="${project}"
* @required
* @readonly
*/
private MavenProject project;
/**
* @parameter expression="${settings}"
* @required
* @since 1.0
* @readonly
*/
private Settings settings;
/**
* @parameter default-value="${session}"
* @required
* @readonly
*/
private MavenSession mavenSession;
protected void beforeExecution() throws MojoExecutionException, MojoFailureException {
}
protected void afterExecution() throws MojoExecutionException, MojoFailureException {
}
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
beforeExecution();
if (isStartMavenLogger()) {
MavenLogger.startPluginLog(this);
}
if (skipMojo()) {
return;
}
executeMojo();
if (isStartMavenLogger()) {
MavenLogger.endPluginLog(this);
}
afterExecution();
}
protected abstract void executeMojo() throws MojoExecutionException, MojoFailureException;
/**
*
* Determine if the mojo execution should get skipped.
*
* This is the case if:
*
* - {@link #skip} is
true
* - if the mojo gets executed on a project with packaging type 'pom' and
* {@link #forceMojoExecution} is
false
*
*
* @return true
if the mojo execution should be skipped.
*/
protected boolean skipMojo() {
if (skip) {
getLog().info("Skipping execution");
return true;
}
if (!forceMojoExecution && project != null && SKIP_PACKAGING_TYPE.equals(project.getPackaging())) {
getLog().info("Skipping execution for project with packaging type '" + SKIP_PACKAGING_TYPE + "'");
return true;
}
return false;
}
/**
* Returns the maven project.
*
* @return The maven project where this plugin runs in.
*/
public MavenProject getProject() {
return project;
}
public String getEncoding() {
return encoding;
}
public void setEncoding(String encoding) {
this.encoding = encoding;
}
public boolean isSkip() {
return skip;
}
public void setSkip(boolean skip) {
this.skip = skip;
}
public boolean isForceMojoExecution() {
return forceMojoExecution;
}
public void setForceMojoExecution(boolean forceMojoExecution) {
this.forceMojoExecution = forceMojoExecution;
}
public Settings getSettings() {
return settings;
}
public void setSettings(Settings settings) {
this.settings = settings;
}
public MavenSession getMavenSession() {
return mavenSession;
}
public void setMavenSession(MavenSession mavenSession) {
this.mavenSession = mavenSession;
}
public void setProject(MavenProject project) {
this.project = project;
}
public boolean isStartMavenLogger() {
return startMavenLogger;
}
public void setStartMavenLogger(boolean startMavenLogger) {
this.startMavenLogger = startMavenLogger;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy