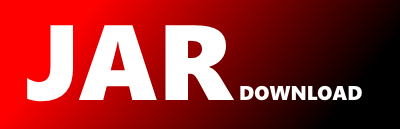
org.kuali.student.admin.ui.mojo.AdminUiLookupViewBeanWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-kscontractdoc-plugin Show documentation
Show all versions of maven-kscontractdoc-plugin Show documentation
Generates documentation for Kuali Student service contracts
The newest version!
/*
* Copyright 2009 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.osedu.org/licenses/ECL-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.student.admin.ui.mojo;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Stack;
import org.kuali.student.contract.model.Lookup;
import org.kuali.student.contract.model.MessageStructure;
import org.kuali.student.contract.model.Service;
import org.kuali.student.contract.model.ServiceContractModel;
import org.kuali.student.contract.model.ServiceMethod;
import org.kuali.student.contract.model.XmlType;
import org.kuali.student.contract.model.impl.ServiceContractModelQDoxLoader;
import org.kuali.student.contract.model.util.ModelFinder;
import org.kuali.student.contract.writer.XmlWriter;
import org.kuali.student.contract.writer.service.GetterSetterNameCalculator;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
*
* @author nwright
*/
public class AdminUiLookupViewBeanWriter {
private static final Logger log = LoggerFactory.getLogger(AdminUiLookupViewBeanWriter.class);
private ServiceContractModel model;
private ModelFinder finder;
private String directory;
private String rootPackage;
private String servKey;
private Service service;
private XmlType xmlType;
private List methods;
private XmlWriter out;
String fileName;
String fullDirectoryPath;
public AdminUiLookupViewBeanWriter(ServiceContractModel model,
String directory,
String rootPackage,
String servKey,
XmlType xmlType,
List methods) {
this.model = model;
this.finder = new ModelFinder(model);
this.directory = directory;
this.rootPackage = rootPackage;
this.servKey = servKey;
service = finder.findService(servKey);
this.xmlType = xmlType;
this.methods = methods;
}
/**
* Write out the entire file
*
* @param out
*/
public void write() {
initXmlWriter();
writeBoilerPlate();
writeBean();
}
private void writeBean() {
String infoClass = GetterSetterNameCalculator.calcInitUpper(xmlType.getName());
String serviceClass = GetterSetterNameCalculator.calcInitUpper(service.getName());
String serviceVar = GetterSetterNameCalculator.calcInitLower(service.getName());
String serviceContract = service.getImplProject() + "." + service.getName();
String lookupable = AdminUiLookupableWriter.calcPackage(servKey, rootPackage, xmlType) + "."
+ AdminUiLookupableWriter.calcClassName(servKey, xmlType);
String viewId = "KS-" + infoClass + "-AdminLookupView";
out.println("");
out.incrementIndent();
out.indentPrintln(" ");
out.indentPrintln("");
out.indentPrintln("");
out.indentPrintln("");
out.indentPrintln("");
out.indentPrintln("");
out.indentPrintln("");
out.incrementIndent();
out.indentPrintln("");
out.incrementIndent();
out.indentPrintln(" ");
this.writeFieldsToSearchOn (xmlType, new Stack(), "");
out.indentPrintln(" ");
out.decrementIndent();
out.indentPrintln("
");
out.decrementIndent();
out.indentPrintln(" ");
out.indentPrintln("");
out.indentPrintln(" ");
for (MessageStructure ms : this.getFieldsToShowOnLookup()) {
String fieldName = GetterSetterNameCalculator.calcInitLower(ms.getShortName());
out.indentPrint(" ");
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln(" ");
} else if (AdminUiInquiryViewBeanWriter.shouldDoLookup (ms.getLookup())) {
out.println(">");
Lookup lookup = ms.getLookup();
XmlType msType = finder.findXmlType(lookup.getXmlTypeName());
MessageStructure pk = this.getPrimaryKey(ms.getLookup().getXmlTypeName());
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln(" ");
out.indentPrintln("
");
} else {
out.println(" />");
}
}
out.indentPrintln(" ");
out.indentPrintln("");
out.decrementIndent();
out.indentPrintln("");
out.indentPrintln("");
out.decrementIndent();
out.indentPrintln("");
}
private MessageStructure getPrimaryKey(String xmlTypeName) {
for (MessageStructure ms : finder.findMessageStructures(xmlTypeName)) {
if (ms.isPrimaryKey()) {
return ms;
}
}
throw new NullPointerException ("could not find primary key for " + xmlTypeName);
}
private void writeFieldsToSearchOn(XmlType type, Stack parents, String prefix) {
// avoid recursion
if (parents.contains(type)) {
return;
}
parents.push(type);
for (MessageStructure ms : finder.findMessageStructures(type.getName())) {
String fieldName = GetterSetterNameCalculator.calcInitLower(ms.getShortName());
if (!prefix.isEmpty()) {
fieldName = prefix + "." + fieldName;
}
String fieldNameCamel = GetterSetterNameCalculator.dot2Camel(fieldName);
if (ms.getShortName().equalsIgnoreCase("versionInd")) {
out.indentPrintln("");
continue;
}
if (ms.getType().equalsIgnoreCase("AttributeInfoList")) {
out.indentPrintln("");
continue;
}
if (ms.getShortName ().equalsIgnoreCase("name")) {
out.indentPrintln("");
continue;
}
if (ms.getShortName ().equalsIgnoreCase("descr")) {
out.indentPrintln("");
continue;
}
if (ms.getType().endsWith("List")) {
out.indentPrintln("");
continue;
}
XmlType fieldType = finder.findXmlType(ms.getType());
if (fieldType.getPrimitive().equalsIgnoreCase(XmlType.COMPLEX)) {
// complex sub-types such as rich text
this.writeFieldsToSearchOn(fieldType, parents, fieldName);
continue;
}
if (!ms.getType().equalsIgnoreCase("String")) {
out.indentPrintln("");
continue;
}
out.indentPrint(" ");
continue;
}
out.println(">");
out.incrementIndent();
XmlType msType = finder.findXmlType(ms.getLookup().getXmlTypeName());
if (msType == null) {
throw new NullPointerException ("Processing lookup for: " + type.getName ()
+ ms.getName()
+ " lookup=" + ms.getLookup().getXmlTypeName());
}
out.indentPrintln("");
out.incrementIndent();
out.indentPrintln(" ");
out.decrementIndent();
out.indentPrintln(" ");
out.decrementIndent();
out.indentPrintln("");
}
parents.pop();
}
private List getFieldsToSearchOn() {
List list = new ArrayList();
for (MessageStructure ms : finder.findMessageStructures(xmlType.getName())) {
// lists of values cannot be displayed within a list of values
if (ms.getType().endsWith("List")) {
continue;
}
if (ms.getType().endsWith("List")) {
continue;
}
XmlType msType = finder.findXmlType(ms.getType());
// just show fields on the main object for now don't dive down into complex fields
if (msType.getPrimitive().equalsIgnoreCase(XmlType.COMPLEX)) {
continue;
}
}
return list;
}
private List getFieldsToShowOnLookup() {
List list = new ArrayList();
for (MessageStructure ms : finder.findMessageStructures(xmlType.getName())) {
// lists of values cannot be displayed within a list of values
if (ms.getType().endsWith("List")) {
continue;
}
XmlType msType = finder.findXmlType(ms.getType());
// just show fields on the main object for now don't dive down into complex fields
if (msType.getPrimitive().equalsIgnoreCase(XmlType.COMPLEX)) {
continue;
}
list.add(ms);
}
return list;
}
private void initXmlWriter() {
String infoClass = GetterSetterNameCalculator.calcInitUpper(xmlType.getName());
String serviceClass = GetterSetterNameCalculator.calcInitUpper(service.getName());
String serviceVar = GetterSetterNameCalculator.calcInitLower(service.getName());
String serviceContract = service.getImplProject() + "." + service.getName();
fullDirectoryPath = AdminUiLookupableWriter.calcPackage(servKey, rootPackage, xmlType).replace('.', '/');
fileName = infoClass + "AdminLookupView.xml";
File dir = new File(this.directory);
if (!dir.exists()) {
if (!dir.mkdirs()) {
throw new IllegalStateException("Could not create directory "
+ this.directory);
}
}
String dirStr = this.directory + "/" + "resources" + "/" + fullDirectoryPath;
File dirFile = new File(dirStr);
if (!dirFile.exists()) {
if (!dirFile.mkdirs()) {
throw new IllegalStateException(
"Could not create directory " + dirStr);
}
}
try {
PrintStream out = new PrintStream(new FileOutputStream(
dirStr + "/"
+ fileName, false));
log.info("AdminUILookupViewBeanWriter: writing " + dirStr + "/" + fileName);
this.out = new XmlWriter(out, 0);
} catch (FileNotFoundException ex) {
throw new IllegalStateException(ex);
}
}
private void writeBoilerPlate() {
out.println("");
out.println("");
out.println(" ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy