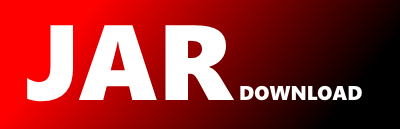
org.kuali.ole.OleDocStoreData Maven / Gradle / Ivy
/*
* Copyright 2011 The Kuali Foundation.
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.ole;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Created by IntelliJ IDEA.
* User: pvsubrah
* Date: 9/8/11
* Time: 10:41 AM
* To change this template use File | Settings | File Templates.
*/
public class OleDocStoreData {
private String category;
private List doctypes = new ArrayList();
private List formats = new ArrayList();
private Map> typeFormatMap = new HashMap>();
private Map formatLevelsMap = new HashMap();
private Map> typeFormatMapWithNodeCount = new HashMap>();
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
public Map> getTypeFormatMap() {
return typeFormatMap;
}
public List getDoctypes() {
return doctypes;
}
public void setDoctypes(List doctypes) {
this.doctypes = doctypes;
}
public void addDocType(String docType) {
if (!this.doctypes.contains(docType)) {
this.doctypes.add(docType);
}
}
public void addFormat(String docType, String format) {
List formatsList = null;
if (!this.typeFormatMap.containsKey(docType)) {
this.typeFormatMap.put(docType, new ArrayList());
}
formatsList = this.typeFormatMap.get(docType);
if (!formatsList.contains(format)) {
formatsList.add(format);
formats.add(format);
}
this.typeFormatMap.put(docType, formatsList);
}
public void addLevelInfoForFormat(String format, Integer levels) {
formatLevelsMap.put(format, levels);
}
public Map> getTypeFormatMapWithNodeCount() {
return typeFormatMapWithNodeCount;
}
public void setTypeFormatMapWithNodeCount(Map> typeFormatMapWithNodeCount) {
this.typeFormatMapWithNodeCount = typeFormatMapWithNodeCount;
}
public Map getFormatLevelsMap() {
return formatLevelsMap;
}
public void setFormatLevelsMap(Map formatLevelsMap) {
this.formatLevelsMap = formatLevelsMap;
}
public List getFormats() {
return formats;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy