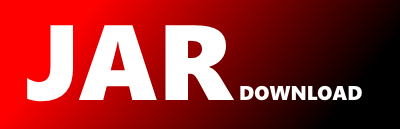
org.kuali.rice.test.ClearDatabaseLifecycle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rice-it-internal-tools Show documentation
Show all versions of rice-it-internal-tools Show documentation
This module contains rice's internal integration testing tools. This module is internal to rice and should not used outside of the rice project. It should only be depended on by rice integration test code. This module can depend on any part of rice.
/**
* Copyright 2005-2014 The Kuali Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ecl2.php
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.kuali.rice.test;
import org.apache.commons.lang.StringUtils;
import org.apache.commons.lang.time.DurationFormatUtils;
import org.apache.commons.lang.time.StopWatch;
import org.apache.log4j.Logger;
import org.junit.Assert;
import org.kuali.rice.core.api.config.property.ConfigContext;
import org.kuali.rice.core.api.lifecycle.BaseLifecycle;
import org.springframework.dao.DataAccessException;
import org.springframework.jdbc.core.ConnectionCallback;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.jdbc.core.StatementCallback;
import org.springframework.transaction.PlatformTransactionManager;
import org.springframework.transaction.TransactionStatus;
import org.springframework.transaction.support.TransactionCallback;
import org.springframework.transaction.support.TransactionTemplate;
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Lifecycle class to clean up the database for use in testing.
* This lifecycle will not be run (even if it is listed in the lifecycles list)
* if the 'use.use.clearDatabaseLifecycle' configuration property is defined, and is
* not 'true'. If the property is omitted the lifecycle runs as normal.
*
* @author Kuali Rice Team ([email protected])
* @since 0.9
*
*/
public class ClearDatabaseLifecycle extends BaseLifecycle {
protected static final Logger LOG = Logger.getLogger(ClearDatabaseLifecycle.class);
private List tablesToClear = new ArrayList();
private List tablesNotToClear = new ArrayList();
public ClearDatabaseLifecycle() {
addStandardTables();
}
public ClearDatabaseLifecycle(List tablesToClear, List tablesNotToClear) {
this.tablesToClear = tablesToClear;
this.tablesNotToClear = tablesNotToClear;
addStandardTables();
}
protected void addStandardTables() {
tablesNotToClear.add("BIN.*");
tablesNotToClear.add(".*_S");
}
public static final String TEST_TABLE_NAME = "EN_UNITTEST_T";
public void start() throws Exception {
String useClearDatabaseLifecycle = ConfigContext.getCurrentContextConfig().getProperty("use.clearDatabaseLifecycle");
if (useClearDatabaseLifecycle != null && !Boolean.valueOf(useClearDatabaseLifecycle)) {
LOG.debug("Skipping ClearDatabaseLifecycle due to property: use.clearDatabaseLifecycle=" + useClearDatabaseLifecycle);
return;
}
final DataSource dataSource = TestHarnessServiceLocator.getDataSource();
clearTables(TestHarnessServiceLocator.getJtaTransactionManager(), dataSource);
super.start();
}
protected Boolean isTestTableInSchema(final Connection connection) throws SQLException {
Assert.assertNotNull("Connection could not be located.", connection);
ResultSet resultSet = null;
try {
resultSet = connection.getMetaData().getTables(null, connection.getMetaData().getUserName().toUpperCase(), TEST_TABLE_NAME, null);
return new Boolean(resultSet.next());
} finally {
if (resultSet != null) {
resultSet.close();
}
}
}
protected void verifyTestEnvironment(final DataSource dataSource) {
new JdbcTemplate(dataSource).execute(new ConnectionCallback
© 2015 - 2024 Weber Informatics LLC | Privacy Policy