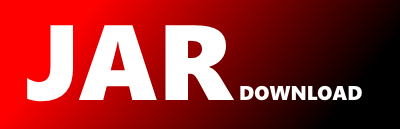
org.kurento.client.BaseRtpEndpoint Maven / Gradle / Ivy
Show all versions of kurento-client Show documentation
/**
* This file is generated with Kurento-maven-plugin.
* Please don't edit.
*/
package org.kurento.client;
/**
*
* Handles RTP communications.
*
* All endpoints that rely on the RTP protocol, like the
* RtpEndpoint or the WebRtcEndpoint, inherit
* from this class. The endpoint provides information about the connection state
* and the media state, which can be consulted at any time through the
* {@link #mediaState} and the {@link #connectionState} properties. It is
* also possible subscribe to events fired when these properties change.
*
*
* -
* ConnectionStateChangedEvent: This event is raised when the connection
* between two peers changes. It can have two values:
*
* - CONNECTED
* - DISCONNECTED
*
*
* -
* MediaStateChangedEvent: Based on RTCP packet flow, this event provides more
* reliable information about the state of media flow. Since RTCP packets are
* not flowing at a constant rate (minimizing a browser with an
* RTCPeerConnection might affect this interval, for instance), there is a
* guard period of about 5s. This traduces in a period where there might be no
* media flowing, but the event hasn't been fired yet. Nevertheless, this is
* the most reliable and useful way of knowing what the state of media exchange
* is. Possible values are:
*
* - CONNECTED: There is an RTCP packet flow between peers.
* -
* DISCONNECTED: No RTCP packets have been received, or at least 5s have
* passed since the last packet arrived.
*
*
*
*
*
* Part of the bandwidth control of the video component of the media session is
* done here:
*
*
* -
* Input bandwidth: Configuration value used to inform remote peers about the
* bitrate that can be pushed into this endpoint.
*
* -
* {get,set}MinVideoRecvBandwidth: Minimum bitrate
* requested on the received video stream.
*
* -
* {get,set}Max{Audio,Video}RecvBandwidth: Maximum bitrate
* expected for the received stream.
*
*
*
* -
* Output bandwidth: Configuration values used to control bitrate of the output
* video stream sent to remote peers. It is important to keep in mind that
* pushed bitrate depends on network and remote peer capabilities. Remote peers
* can also announce bandwidth limitation in their SDPs (through the
*
b={modifier}:{value}
tag). Kurento will always enforce bitrate
* limitations specified by the remote peer over internal configurations.
*
* -
* {get,set}MinVideoSendBandwidth: Minimum video bitrate
* sent to remote peer.
*
* -
* {get,set}MaxVideoSendBandwidth: Maximum video bitrate
* sent to remote peer.
*
* -
* RembParams.rembOnConnect: Initial local REMB bandwidth
* estimation that gets propagated when a new endpoint is connected.
*
*
*
*
*
*
* All bandwidth control parameters must be changed before the SDP negotiation
* takes place, and can't be changed afterwards.
*
*
*
*
**/
@org.kurento.client.internal.RemoteClass
public interface BaseRtpEndpoint extends SdpEndpoint {
/**
*
* Get Minimum bitrate requested on the received video stream.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
int getMinVideoRecvBandwidth();
/**
*
* Get Minimum bitrate requested on the received video stream.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
void getMinVideoRecvBandwidth(Continuation cont);
/**
*
* Get Minimum bitrate requested on the received video stream.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
TFuture getMinVideoRecvBandwidth(Transaction tx);
/**
*
* Set Minimum bitrate requested on the received video stream.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
void setMinVideoRecvBandwidth(@org.kurento.client.internal.server.Param("minVideoRecvBandwidth") int minVideoRecvBandwidth);
/**
*
* Set Minimum bitrate requested on the received video stream.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
void setMinVideoRecvBandwidth(@org.kurento.client.internal.server.Param("minVideoRecvBandwidth") int minVideoRecvBandwidth, Continuation cont);
/**
*
* Set Minimum bitrate requested on the received video stream.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
void setMinVideoRecvBandwidth(@org.kurento.client.internal.server.Param("minVideoRecvBandwidth") int minVideoRecvBandwidth, Transaction tx);
/**
*
* Get Minimum video bitrate sent to remote peer.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unconstrained: the video bitrate will drop as needed, even to the
* lowest possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
int getMinVideoSendBandwidth();
/**
*
* Get Minimum video bitrate sent to remote peer.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unconstrained: the video bitrate will drop as needed, even to the
* lowest possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
void getMinVideoSendBandwidth(Continuation cont);
/**
*
* Get Minimum video bitrate sent to remote peer.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unconstrained: the video bitrate will drop as needed, even to the
* lowest possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
TFuture getMinVideoSendBandwidth(Transaction tx);
/**
*
* Set Minimum video bitrate sent to remote peer.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unconstrained: the video bitrate will drop as needed, even to the
* lowest possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
void setMinVideoSendBandwidth(@org.kurento.client.internal.server.Param("minVideoSendBandwidth") int minVideoSendBandwidth);
/**
*
* Set Minimum video bitrate sent to remote peer.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unconstrained: the video bitrate will drop as needed, even to the
* lowest possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
void setMinVideoSendBandwidth(@org.kurento.client.internal.server.Param("minVideoSendBandwidth") int minVideoSendBandwidth, Continuation cont);
/**
*
* Set Minimum video bitrate sent to remote peer.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unconstrained: the video bitrate will drop as needed, even to the
* lowest possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
void setMinVideoSendBandwidth(@org.kurento.client.internal.server.Param("minVideoSendBandwidth") int minVideoSendBandwidth, Transaction tx);
/**
*
* Get Maximum video bitrate sent to remote peer.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than
* this parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this parameter to higher values such as 2000 (2
* mbps) or even 10000 (10 mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unconstrained: the video bitrate will grow until all the available
* network bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
int getMaxVideoSendBandwidth();
/**
*
* Get Maximum video bitrate sent to remote peer.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than
* this parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this parameter to higher values such as 2000 (2
* mbps) or even 10000 (10 mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unconstrained: the video bitrate will grow until all the available
* network bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
void getMaxVideoSendBandwidth(Continuation cont);
/**
*
* Get Maximum video bitrate sent to remote peer.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than
* this parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this parameter to higher values such as 2000 (2
* mbps) or even 10000 (10 mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unconstrained: the video bitrate will grow until all the available
* network bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
TFuture getMaxVideoSendBandwidth(Transaction tx);
/**
*
* Set Maximum video bitrate sent to remote peer.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than
* this parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this parameter to higher values such as 2000 (2
* mbps) or even 10000 (10 mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unconstrained: the video bitrate will grow until all the available
* network bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
void setMaxVideoSendBandwidth(@org.kurento.client.internal.server.Param("maxVideoSendBandwidth") int maxVideoSendBandwidth);
/**
*
* Set Maximum video bitrate sent to remote peer.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than
* this parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this parameter to higher values such as 2000 (2
* mbps) or even 10000 (10 mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unconstrained: the video bitrate will grow until all the available
* network bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
void setMaxVideoSendBandwidth(@org.kurento.client.internal.server.Param("maxVideoSendBandwidth") int maxVideoSendBandwidth, Continuation cont);
/**
*
* Set Maximum video bitrate sent to remote peer.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than
* this parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this parameter to higher values such as 2000 (2
* mbps) or even 10000 (10 mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unconstrained: the video bitrate will grow until all the available
* network bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
void setMaxVideoSendBandwidth(@org.kurento.client.internal.server.Param("maxVideoSendBandwidth") int maxVideoSendBandwidth, Transaction tx);
/**
*
* Get Media flow state.
*
* - CONNECTED: There is an RTCP flow.
* - DISCONNECTED: No RTCP packets have been received for at least 5 sec.
*
*
*
**/
org.kurento.client.MediaState getMediaState();
/**
*
* Get Media flow state.
*
* - CONNECTED: There is an RTCP flow.
* - DISCONNECTED: No RTCP packets have been received for at least 5 sec.
*
*
*
**/
void getMediaState(Continuation cont);
/**
*
* Get Media flow state.
*
* - CONNECTED: There is an RTCP flow.
* - DISCONNECTED: No RTCP packets have been received for at least 5 sec.
*
*
*
**/
TFuture getMediaState(Transaction tx);
/**
*
* Get Connection state.
*
* - CONNECTED
* - DISCONNECTED
*
*
*
**/
org.kurento.client.ConnectionState getConnectionState();
/**
*
* Get Connection state.
*
* - CONNECTED
* - DISCONNECTED
*
*
*
**/
void getConnectionState(Continuation cont);
/**
*
* Get Connection state.
*
* - CONNECTED
* - DISCONNECTED
*
*
*
**/
TFuture getConnectionState(Transaction tx);
/**
*
* Get Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
int getMtu();
/**
*
* Get Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
void getMtu(Continuation cont);
/**
*
* Get Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
TFuture getMtu(Transaction tx);
/**
*
* Set Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
void setMtu(@org.kurento.client.internal.server.Param("mtu") int mtu);
/**
*
* Set Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
void setMtu(@org.kurento.client.internal.server.Param("mtu") int mtu, Continuation cont);
/**
*
* Set Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
void setMtu(@org.kurento.client.internal.server.Param("mtu") int mtu, Transaction tx);
/**
*
* Get Advanced parameters to configure the congestion control algorithm.
*
**/
org.kurento.client.RembParams getRembParams();
/**
*
* Get Advanced parameters to configure the congestion control algorithm.
*
**/
void getRembParams(Continuation cont);
/**
*
* Get Advanced parameters to configure the congestion control algorithm.
*
**/
TFuture getRembParams(Transaction tx);
/**
*
* Set Advanced parameters to configure the congestion control algorithm.
*
**/
void setRembParams(@org.kurento.client.internal.server.Param("rembParams") org.kurento.client.RembParams rembParams);
/**
*
* Set Advanced parameters to configure the congestion control algorithm.
*
**/
void setRembParams(@org.kurento.client.internal.server.Param("rembParams") org.kurento.client.RembParams rembParams, Continuation cont);
/**
*
* Set Advanced parameters to configure the congestion control algorithm.
*
**/
void setRembParams(@org.kurento.client.internal.server.Param("rembParams") org.kurento.client.RembParams rembParams, Transaction tx);
/**
* Add a {@link EventListener} for event {@link MediaStateChangedEvent}. Synchronous call.
*
* @param listener Listener to be called on MediaStateChangedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(MediaStateChangedEvent.class)
ListenerSubscription addMediaStateChangedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link MediaStateChangedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on MediaStateChangedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(MediaStateChangedEvent.class)
void addMediaStateChangedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link MediaStateChangedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(MediaStateChangedEvent.class)
void removeMediaStateChangedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link MediaStateChangedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(MediaStateChangedEvent.class)
void removeMediaStateChangedListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link ConnectionStateChangedEvent}. Synchronous call.
*
* @param listener Listener to be called on ConnectionStateChangedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(ConnectionStateChangedEvent.class)
ListenerSubscription addConnectionStateChangedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link ConnectionStateChangedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on ConnectionStateChangedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(ConnectionStateChangedEvent.class)
void addConnectionStateChangedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link ConnectionStateChangedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(ConnectionStateChangedEvent.class)
void removeConnectionStateChangedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link ConnectionStateChangedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(ConnectionStateChangedEvent.class)
void removeConnectionStateChangedListener(ListenerSubscription listenerSubscription, Continuation cont);
}