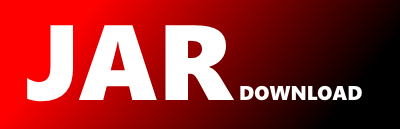
org.kurento.client.SdpEndpoint Maven / Gradle / Ivy
Show all versions of kurento-client Show documentation
/**
* This file is generated with Kurento-maven-plugin.
* Please don't edit.
*/
package org.kurento.client;
/**
*
* Interface implemented by Endpoints that require an SDP negotiation for the setup
* of a networked media session with remote peers.
* The API provides the following functionality:
*
* - Generate SDP offers.
* - Process SDP offers.
* - Configure SDP related params.
*
*
*
**/
@org.kurento.client.internal.RemoteClass
public interface SdpEndpoint extends SessionEndpoint {
/**
*
* Get Maximum bitrate expected for the received audio stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
int getMaxAudioRecvBandwidth();
/**
*
* Get Maximum bitrate expected for the received audio stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
void getMaxAudioRecvBandwidth(Continuation cont);
/**
*
* Get Maximum bitrate expected for the received audio stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
TFuture getMaxAudioRecvBandwidth(Transaction tx);
/**
*
* Set Maximum bitrate expected for the received audio stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
void setMaxAudioRecvBandwidth(@org.kurento.client.internal.server.Param("maxAudioRecvBandwidth") int maxAudioRecvBandwidth);
/**
*
* Set Maximum bitrate expected for the received audio stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
void setMaxAudioRecvBandwidth(@org.kurento.client.internal.server.Param("maxAudioRecvBandwidth") int maxAudioRecvBandwidth, Continuation cont);
/**
*
* Set Maximum bitrate expected for the received audio stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
void setMaxAudioRecvBandwidth(@org.kurento.client.internal.server.Param("maxAudioRecvBandwidth") int maxAudioRecvBandwidth, Transaction tx);
/**
*
* Get Maximum bitrate expected for the received video stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
int getMaxVideoRecvBandwidth();
/**
*
* Get Maximum bitrate expected for the received video stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
void getMaxVideoRecvBandwidth(Continuation cont);
/**
*
* Get Maximum bitrate expected for the received video stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
TFuture getMaxVideoRecvBandwidth(Transaction tx);
/**
*
* Set Maximum bitrate expected for the received video stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
void setMaxVideoRecvBandwidth(@org.kurento.client.internal.server.Param("maxVideoRecvBandwidth") int maxVideoRecvBandwidth);
/**
*
* Set Maximum bitrate expected for the received video stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
void setMaxVideoRecvBandwidth(@org.kurento.client.internal.server.Param("maxVideoRecvBandwidth") int maxVideoRecvBandwidth, Continuation cont);
/**
*
* Set Maximum bitrate expected for the received video stream.
*
* This is used to put a limit on the bitrate that the remote peer will send to
* this endpoint. The net effect of setting this parameter is that
* when Kurento generates an SDP Offer, an 'Application Specific' (AS)
* maximum bandwidth attribute will be added to the SDP media section:
* b=AS:{value}
.
*
* Note: This parameter has to be set before the SDP is generated.
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* - 0 = unconstrained.
*
*
*
**/
void setMaxVideoRecvBandwidth(@org.kurento.client.internal.server.Param("maxVideoRecvBandwidth") int maxVideoRecvBandwidth, Transaction tx);
/**
*
* Generates an SDP offer with media capabilities of the Endpoint.
* Throws:
*
* -
* SDP_END_POINT_ALREADY_NEGOTIATED If the endpoint is already negotiated.
*
* -
* SDP_END_POINT_GENERATE_OFFER_ERROR if the generated offer is empty. This is
* most likely due to an internal error.
*
*
*
* @return The SDP offer. *
**/
String generateOffer();
/**
*
* Asynchronous version of generateOffer:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see SdpEndpoint#generateOffer
*
**/
void generateOffer(Continuation cont);
/**
*
* Generates an SDP offer with media capabilities of the Endpoint.
* Throws:
*
* -
* SDP_END_POINT_ALREADY_NEGOTIATED If the endpoint is already negotiated.
*
* -
* SDP_END_POINT_GENERATE_OFFER_ERROR if the generated offer is empty. This is
* most likely due to an internal error.
*
*
*
* @return The SDP offer. *
**/
TFuture generateOffer(Transaction tx);
/**
*
* Processes SDP offer of the remote peer, and generates an SDP answer based on the endpoint's capabilities.
*
* If no matching capabilities are found, the SDP will contain no codecs.
*
* Throws:
*
* -
* SDP_PARSE_ERROR If the offer is empty or has errors.
*
* -
* SDP_END_POINT_ALREADY_NEGOTIATED If the endpoint is already negotiated.
*
* -
* SDP_END_POINT_PROCESS_OFFER_ERROR if the generated offer is empty. This is
* most likely due to an internal error.
*
*
*
*
* @param offer
* SessionSpec offer from the remote User Agent
* @return The chosen configuration from the ones stated in the SDP offer. *
**/
String processOffer(@org.kurento.client.internal.server.Param("offer") String offer);
/**
*
* Asynchronous version of processOffer:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see SdpEndpoint#processOffer
*
* @param offer
* SessionSpec offer from the remote User Agent
*
**/
void processOffer(@org.kurento.client.internal.server.Param("offer") String offer, Continuation cont);
/**
*
* Processes SDP offer of the remote peer, and generates an SDP answer based on the endpoint's capabilities.
*
* If no matching capabilities are found, the SDP will contain no codecs.
*
* Throws:
*
* -
* SDP_PARSE_ERROR If the offer is empty or has errors.
*
* -
* SDP_END_POINT_ALREADY_NEGOTIATED If the endpoint is already negotiated.
*
* -
* SDP_END_POINT_PROCESS_OFFER_ERROR if the generated offer is empty. This is
* most likely due to an internal error.
*
*
*
*
* @param offer
* SessionSpec offer from the remote User Agent
* @return The chosen configuration from the ones stated in the SDP offer. *
**/
TFuture processOffer(Transaction tx, @org.kurento.client.internal.server.Param("offer") String offer);
/**
*
* Generates an SDP offer with media capabilities of the Endpoint.
* Throws:
*
* -
* SDP_PARSE_ERROR If the offer is empty or has errors.
*
* -
* SDP_END_POINT_ALREADY_NEGOTIATED If the endpoint is already negotiated.
*
* -
* SDP_END_POINT_PROCESS_ANSWER_ERROR if the result of processing the answer is
* an empty string. This is most likely due to an internal error.
*
* -
* SDP_END_POINT_NOT_OFFER_GENERATED If the method is invoked before the
* generateOffer method.
*
*
*
*
* @param answer
* SessionSpec answer from the remote User Agent
* @return Updated SDP offer, based on the answer received. *
**/
String processAnswer(@org.kurento.client.internal.server.Param("answer") String answer);
/**
*
* Asynchronous version of processAnswer:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see SdpEndpoint#processAnswer
*
* @param answer
* SessionSpec answer from the remote User Agent
*
**/
void processAnswer(@org.kurento.client.internal.server.Param("answer") String answer, Continuation cont);
/**
*
* Generates an SDP offer with media capabilities of the Endpoint.
* Throws:
*
* -
* SDP_PARSE_ERROR If the offer is empty or has errors.
*
* -
* SDP_END_POINT_ALREADY_NEGOTIATED If the endpoint is already negotiated.
*
* -
* SDP_END_POINT_PROCESS_ANSWER_ERROR if the result of processing the answer is
* an empty string. This is most likely due to an internal error.
*
* -
* SDP_END_POINT_NOT_OFFER_GENERATED If the method is invoked before the
* generateOffer method.
*
*
*
*
* @param answer
* SessionSpec answer from the remote User Agent
* @return Updated SDP offer, based on the answer received. *
**/
TFuture processAnswer(Transaction tx, @org.kurento.client.internal.server.Param("answer") String answer);
/**
*
* Returns the local SDP.
*
* -
* No offer has been generated: returns null.
*
* -
* Offer has been generated: returns the SDP offer.
*
* -
* Offer has been generated and answer processed: returns the agreed SDP.
*
*
*
* @return The last agreed SessionSpec. *
**/
String getLocalSessionDescriptor();
/**
*
* Asynchronous version of getLocalSessionDescriptor:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see SdpEndpoint#getLocalSessionDescriptor
*
**/
void getLocalSessionDescriptor(Continuation cont);
/**
*
* Returns the local SDP.
*
* -
* No offer has been generated: returns null.
*
* -
* Offer has been generated: returns the SDP offer.
*
* -
* Offer has been generated and answer processed: returns the agreed SDP.
*
*
*
* @return The last agreed SessionSpec. *
**/
TFuture getLocalSessionDescriptor(Transaction tx);
/**
*
* This method returns the remote SDP.
* If the negotiation process is not complete, it will return NULL.
*
* @return The last agreed User Agent session description. *
**/
String getRemoteSessionDescriptor();
/**
*
* Asynchronous version of getRemoteSessionDescriptor:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see SdpEndpoint#getRemoteSessionDescriptor
*
**/
void getRemoteSessionDescriptor(Continuation cont);
/**
*
* This method returns the remote SDP.
* If the negotiation process is not complete, it will return NULL.
*
* @return The last agreed User Agent session description. *
**/
TFuture getRemoteSessionDescriptor(Transaction tx);
}