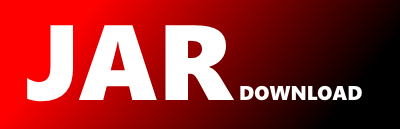
org.kurento.client.ServerManager Maven / Gradle / Ivy
Show all versions of kurento-client Show documentation
/**
* This file is generated with Kurento-maven-plugin.
* Please don't edit.
*/
package org.kurento.client;
/**
*
* This is a standalone object for managing the MediaServer
*
**/
@org.kurento.client.internal.RemoteClass
public interface ServerManager extends MediaObject {
/**
*
* Get Server information, version, modules, factories, etc
*
**/
org.kurento.client.ServerInfo getInfo();
/**
*
* Get Server information, version, modules, factories, etc
*
**/
void getInfo(Continuation cont);
/**
*
* Get Server information, version, modules, factories, etc
*
**/
TFuture getInfo(Transaction tx);
/**
*
* Get All the pipelines available in the server
*
**/
java.util.List getPipelines();
/**
*
* Get All the pipelines available in the server
*
**/
void getPipelines(Continuation> cont);
/**
*
* Get All the pipelines available in the server
*
**/
TFuture> getPipelines(Transaction tx);
/**
*
* Get All active sessions in the server
*
**/
java.util.List getSessions();
/**
*
* Get All active sessions in the server
*
**/
void getSessions(Continuation> cont);
/**
*
* Get All active sessions in the server
*
**/
TFuture> getSessions(Transaction tx);
/**
*
* Get Metadata stored in the server
*
**/
String getMetadata();
/**
*
* Get Metadata stored in the server
*
**/
void getMetadata(Continuation cont);
/**
*
* Get Metadata stored in the server
*
**/
TFuture getMetadata(Transaction tx);
/**
*
* Returns the kmd associated to a module
*
* @param moduleName
* Name of the module to get its kmd file
* @return The kmd file. *
**/
String getKmd(@org.kurento.client.internal.server.Param("moduleName") String moduleName);
/**
*
* Asynchronous version of getKmd:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see ServerManager#getKmd
*
* @param moduleName
* Name of the module to get its kmd file
*
**/
void getKmd(@org.kurento.client.internal.server.Param("moduleName") String moduleName, Continuation cont);
/**
*
* Returns the kmd associated to a module
*
* @param moduleName
* Name of the module to get its kmd file
* @return The kmd file. *
**/
TFuture getKmd(Transaction tx, @org.kurento.client.internal.server.Param("moduleName") String moduleName);
/**
*
* Number of CPU cores that the media server can use.
*
* Linux processes can be configured to use only a subset of the cores that are
* available in the system, via the process affinity settings
* (sched_setaffinity(2)). With this method it is possible to
* know the number of cores that the media server can use in the machine where it
* is running.
*
*
* For example, it's possible to limit the core affinity inside a Docker
* container by running with a command such as
* docker run --cpuset-cpus='0,1'.
*
*
* Note that the return value represents the number of
* logical processing units available, i.e. CPU cores including
* Hyper-Threading.
*
*
* @return Number of CPU cores available for the media server. *
**/
int getCpuCount();
/**
*
* Asynchronous version of getCpuCount:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see ServerManager#getCpuCount
*
**/
void getCpuCount(Continuation cont);
/**
*
* Number of CPU cores that the media server can use.
*
* Linux processes can be configured to use only a subset of the cores that are
* available in the system, via the process affinity settings
* (sched_setaffinity(2)). With this method it is possible to
* know the number of cores that the media server can use in the machine where it
* is running.
*
*
* For example, it's possible to limit the core affinity inside a Docker
* container by running with a command such as
* docker run --cpuset-cpus='0,1'.
*
*
* Note that the return value represents the number of
* logical processing units available, i.e. CPU cores including
* Hyper-Threading.
*
*
* @return Number of CPU cores available for the media server. *
**/
TFuture getCpuCount(Transaction tx);
/**
*
* Average CPU usage of the server.
*
* This method measures the average CPU usage of the media server during the
* requested interval. Normally you will want to choose an interval between 1000
* and 10000 ms.
*
*
* The returned value represents the global system CPU usage of the media server,
* as an average across all processing units (CPU cores).
*
*
*
* @param interval
* Time to measure the average CPU usage, in milliseconds.
* @return CPU usage %. *
**/
float getUsedCpu(@org.kurento.client.internal.server.Param("interval") int interval);
/**
*
* Asynchronous version of getUsedCpu:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see ServerManager#getUsedCpu
*
* @param interval
* Time to measure the average CPU usage, in milliseconds.
*
**/
void getUsedCpu(@org.kurento.client.internal.server.Param("interval") int interval, Continuation cont);
/**
*
* Average CPU usage of the server.
*
* This method measures the average CPU usage of the media server during the
* requested interval. Normally you will want to choose an interval between 1000
* and 10000 ms.
*
*
* The returned value represents the global system CPU usage of the media server,
* as an average across all processing units (CPU cores).
*
*
*
* @param interval
* Time to measure the average CPU usage, in milliseconds.
* @return CPU usage %. *
**/
TFuture getUsedCpu(Transaction tx, @org.kurento.client.internal.server.Param("interval") int interval);
/**
*
* Returns the amount of memory that the server is using, in KiB
* @return Used memory, in KiB. *
**/
long getUsedMemory();
/**
*
* Asynchronous version of getUsedMemory:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see ServerManager#getUsedMemory
*
**/
void getUsedMemory(Continuation cont);
/**
*
* Returns the amount of memory that the server is using, in KiB
* @return Used memory, in KiB. *
**/
TFuture getUsedMemory(Transaction tx);
/**
* Add a {@link EventListener} for event {@link ObjectCreatedEvent}. Synchronous call.
*
* @param listener Listener to be called on ObjectCreatedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(ObjectCreatedEvent.class)
ListenerSubscription addObjectCreatedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link ObjectCreatedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on ObjectCreatedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(ObjectCreatedEvent.class)
void addObjectCreatedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link ObjectCreatedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(ObjectCreatedEvent.class)
void removeObjectCreatedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link ObjectCreatedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(ObjectCreatedEvent.class)
void removeObjectCreatedListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link ObjectDestroyedEvent}. Synchronous call.
*
* @param listener Listener to be called on ObjectDestroyedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(ObjectDestroyedEvent.class)
ListenerSubscription addObjectDestroyedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link ObjectDestroyedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on ObjectDestroyedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(ObjectDestroyedEvent.class)
void addObjectDestroyedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link ObjectDestroyedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(ObjectDestroyedEvent.class)
void removeObjectDestroyedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link ObjectDestroyedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(ObjectDestroyedEvent.class)
void removeObjectDestroyedListener(ListenerSubscription listenerSubscription, Continuation cont);
}