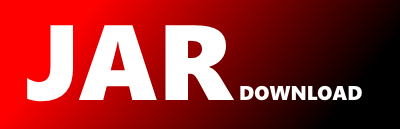
org.kurento.client.WebRtcEndpoint Maven / Gradle / Ivy
Show all versions of kurento-client Show documentation
/**
* This file is generated with Kurento-maven-plugin.
* Please don't edit.
*/
package org.kurento.client;
/**
*
* Control interface for Kurento WebRTC endpoint.
*
* This endpoint is one side of a peer-to-peer WebRTC communication, being the
* other peer a WebRTC capable browser -using the RTCPeerConnection API-, a
* native WebRTC app or even another Kurento Media Server.
*
*
* In order to establish a WebRTC communication, peers engage in an SDP
* negotiation process, where one of the peers (the offerer) sends an offer,
* while the other peer (the offeree) responds with an answer. This endpoint can
* function in both situations
*
*
* -
* As offerer: The negotiation process is initiated by the media server
*
* -
* KMS generates the SDP offer through the
*
generateOffer
method. This offer must then be sent
* to the remote peer (the offeree) through the signaling channel, for
* processing.
*
* -
* The remote peer processes the offer, and generates an
* answer. The answer is sent back to the media server.
*
* -
* Upon receiving the answer, the endpoint must invoke the
*
processAnswer
method.
*
*
*
* -
* As offeree: The negotiation process is initiated by the remote peer
*
* -
* The remote peer, acting as offerer, generates an SDP offer and
* sends it to the WebRTC endpoint in Kurento.
*
* -
* The endpoint will process the offer invoking the
*
processOffer
method. The result of this method will be a
* string, containing an SDP answer.
*
* -
* The SDP answer must be sent back to the offerer, so it can be
* processed.
*
*
*
*
*
* SDPs are sent without ICE candidates, following the Trickle ICE optimization.
* Once the SDP negotiation is completed, both peers proceed with the ICE
* discovery process, intended to set up a bidirectional media connection. During
* this process, each peer
*
*
* -
* Discovers ICE candidates for itself, containing pairs of IPs and ports.
*
* -
* ICE candidates are sent via the signaling channel as they are discovered, to
* the remote peer for probing.
*
* -
* ICE connectivity checks are run as soon as the new candidate description,
* from the remote peer, is available.
*
*
*
* Once a suitable pair of candidates (one for each peer) is discovered, the
* media session can start. The harvesting process in Kurento, begins with the
* invocation of the gatherCandidates
method. Since the whole
* Trickle ICE purpose is to speed-up connectivity, candidates are generated
* asynchronously. Therefore, in order to capture the candidates, the user must
* subscribe to the event IceCandidateFound
. It is important that
* the event listener is bound before invoking gatherCandidates
,
* otherwise a suitable candidate might be lost, and connection might not be
* established.
*
*
* It's important to keep in mind that WebRTC connection is an asynchronous
* process, when designing interactions between different MediaElements. For
* example, it would be pointless to start recording before media is flowing. In
* order to be notified of state changes, the application can subscribe to events
* generated by the WebRtcEndpoint. Following is a full list of events generated
* by WebRtcEndpoint:
*
*
* -
*
IceComponentStateChange
: This event informs only about changes
* in the ICE connection state. Possible values are:
*
* DISCONNECTED
: No activity scheduled
* GATHERING
: Gathering local candidates
* CONNECTING
: Establishing connectivity
* CONNECTED
: At least one working candidate pair
* -
*
READY
: ICE concluded, candidate pair selection is now final
*
* -
*
FAILED
: Connectivity checks have been completed, but media
* connection was not established
*
*
* The transitions between states are covered in RFC5245. It could be said that
* it's network-only, as it only takes into account the state of the network
* connection, ignoring other higher level stuff, like DTLS handshake, RTCP
* flow, etc. This implies that, while the component state is
* CONNECTED
, there might be no media flowing between the peers.
* This makes this event useful only to receive low-level information about the
* connection between peers. Even more, while other events might leave a
* graceful period of time before firing, this event fires immediately after
* the state change is detected.
*
* -
*
IceCandidateFound
: Raised when a new candidate is discovered.
* ICE candidates must be sent to the remote peer of the connection. Failing to
* do so for some or all of the candidates might render the connection
* unusable.
*
* -
*
IceGatheringDone
: Raised when the ICE harvesting process is
* completed. This means that all candidates have already been discovered.
*
* -
*
NewCandidatePairSelected
: Raised when a new ICE candidate pair
* gets selected. The pair contains both local and remote candidates being used
* for a component. This event can be raised during a media session, if a new
* pair of candidates with higher priority in the link are found.
*
* DataChannelOpen
: Raised when a data channel is open.
* DataChannelClose
: Raised when a data channel is closed.
*
*
* Registering to any of above events requires the application to provide a
* callback function. Each event provides different information, so it is
* recommended to consult the signature of the event listeners.
*
*
* Flow control and congestion management is one of the most important features
* of WebRTC. WebRTC connections start with the lowest bandwidth configured and
* slowly ramps up to the maximum available bandwidth, or to the higher limit of
* the exploration range in case no bandwidth limitation is detected. Notice that
* WebRtcEndpoints in Kurento are designed in a way that multiple WebRTC
* connections fed by the same stream share quality. When a new connection is
* added, as it requires to start with low bandwidth, it will cause the rest of
* connections to experience a transient period of degraded quality, until it
* stabilizes its bitrate. This doesn't apply when transcoding is involved.
* Transcoders will adjust their output bitrate based in bandwidth requirements,
* but it won't affect the original stream. If an incoming WebRTC stream needs to
* be transcoded, for whatever reason, all WebRtcEndpoints fed from transcoder
* output will share a separate quality than the ones connected directly to the
* original stream.
*
*
* The default bandwidth range of the endpoint is
* [100 kbps, 500 kbps], but it can be changed separately for
* input/output directions and for audio/video streams.
*
*
*
* Check the extended documentation of these parameters in
* {@link module:core/abstracts.SdpEndpoint SdpEndpoint}, {@link module:core/abstracts.BaseRtpEndpoint BaseRtpEndpoint}, and
* {@link module:core/complexTypes.RembParams RembParams}.
*
*
*
* -
* Input bandwidth: Configuration value used to inform remote peers about the
* bitrate that can be pushed into this endpoint.
*
* -
* {get,set}MinVideoRecvBandwidth: Minimum bitrate
* requested on the received video stream.
*
* -
* {get,set}Max{Audio,Video}RecvBandwidth: Maximum bitrate
* expected for the received stream.
*
*
*
* -
* Output bandwidth: Configuration values used to control bitrate of the output
* video stream sent to remote peers. It is important to keep in mind that
* pushed bitrate depends on network and remote peer capabilities. Remote peers
* can also announce bandwidth limitation in their SDPs (through the
*
b={modifier}:{value}
tag). Kurento will always enforce bitrate
* limitations specified by the remote peer over internal configurations.
*
* -
* {get,set}MinVideoSendBandwidth: Minimum video bitrate
* sent to remote peer.
*
* -
* {get,set}MaxVideoSendBandwidth: Maximum video bitrate
* sent to remote peer.
*
* -
* RembParams.rembOnConnect: Initial local REMB bandwidth
* estimation that gets propagated when a new endpoint is connected.
*
*
*
*
*
*
* All bandwidth control parameters must be changed before the SDP negotiation
* takes place, and can't be changed afterwards.
*
*
*
* DataChannels allow other media elements that make use of the DataPad, to send
* arbitrary data. For instance, if there is a filter that publishes event
* information, it'll be sent to the remote peer through the channel. There is no
* API available for programmers to make use of this feature in the
* WebRtcElement. DataChannels can be configured to provide the following:
*
*
* -
* Reliable or partially reliable delivery of sent messages
*
* -
* In-order or out-of-order delivery of sent messages
*
*
*
* Unreliable, out-of-order delivery is equivalent to raw UDP semantics. The
* message may make it, or it may not, and order is not important. However, the
* channel can be configured to be partially reliable by specifying the
* maximum number of retransmissions or setting a time limit for retransmissions:
* the WebRTC stack will handle the acknowledgments and timeouts.
*
*
* The possibility to create DataChannels in a WebRtcEndpoint must be explicitly
* enabled when creating the endpoint, as this feature is disabled by default. If
* this is the case, they can be created invoking the createDataChannel method.
* The arguments for this method, all of them optional, provide the necessary
* configuration:
*
*
* -
*
label
: assigns a label to the DataChannel. This can help
* identify each possible channel separately.
*
* -
*
ordered
: specifies if the DataChannel guarantees order, which
* is the default mode. If maxPacketLifetime and maxRetransmits have not been
* set, this enables reliable mode.
*
* -
*
maxPacketLifeTime
: The time window in milliseconds, during
* which transmissions and retransmissions may take place in unreliable mode.
* This forces unreliable mode, even if ordered
has been
* activated.
*
* -
*
maxRetransmits
: maximum number of retransmissions that are
* attempted in unreliable mode. This forces unreliable mode, even if
* ordered
has been activated.
*
* -
*
Protocol
: Name of the subprotocol used for data communication.
*
*
*
*
**/
@org.kurento.client.internal.RemoteClass
public interface WebRtcEndpoint extends BaseRtpEndpoint {
/**
*
* Get External (public) IP address of the media server.
*
* If you know what will be the external or public IP address of the media server
* (e.g. because your deployment has an static IP), you can specify it here.
* Doing so has the advantage of not needing to configure STUN/TURN for the media
* server.
*
*
* STUN/TURN are needed only when the media server sits behind a NAT and needs to
* find out its own external IP address. However, if you set a static external IP
* address with this parameter, then there is no need for the STUN/TURN
* auto-discovery.
*
*
* The effect of this parameter is that ALL local ICE candidates that are
* gathered (for WebRTC) will contain the provided external IP address instead of
* the local one.
*
*
* externalAddress
is an IPv4 or IPv6 address.
*
* Examples:
*
* externalAddress=10.70.35.2
* externalAddress=2001:0db8:85a3:0000:0000:8a2e:0370:7334
*
*
*
**/
String getExternalAddress();
/**
*
* Get External (public) IP address of the media server.
*
* If you know what will be the external or public IP address of the media server
* (e.g. because your deployment has an static IP), you can specify it here.
* Doing so has the advantage of not needing to configure STUN/TURN for the media
* server.
*
*
* STUN/TURN are needed only when the media server sits behind a NAT and needs to
* find out its own external IP address. However, if you set a static external IP
* address with this parameter, then there is no need for the STUN/TURN
* auto-discovery.
*
*
* The effect of this parameter is that ALL local ICE candidates that are
* gathered (for WebRTC) will contain the provided external IP address instead of
* the local one.
*
*
* externalAddress
is an IPv4 or IPv6 address.
*
* Examples:
*
* externalAddress=10.70.35.2
* externalAddress=2001:0db8:85a3:0000:0000:8a2e:0370:7334
*
*
*
**/
void getExternalAddress(Continuation cont);
/**
*
* Get External (public) IP address of the media server.
*
* If you know what will be the external or public IP address of the media server
* (e.g. because your deployment has an static IP), you can specify it here.
* Doing so has the advantage of not needing to configure STUN/TURN for the media
* server.
*
*
* STUN/TURN are needed only when the media server sits behind a NAT and needs to
* find out its own external IP address. However, if you set a static external IP
* address with this parameter, then there is no need for the STUN/TURN
* auto-discovery.
*
*
* The effect of this parameter is that ALL local ICE candidates that are
* gathered (for WebRTC) will contain the provided external IP address instead of
* the local one.
*
*
* externalAddress
is an IPv4 or IPv6 address.
*
* Examples:
*
* externalAddress=10.70.35.2
* externalAddress=2001:0db8:85a3:0000:0000:8a2e:0370:7334
*
*
*
**/
TFuture getExternalAddress(Transaction tx);
/**
*
* Set External (public) IP address of the media server.
*
* If you know what will be the external or public IP address of the media server
* (e.g. because your deployment has an static IP), you can specify it here.
* Doing so has the advantage of not needing to configure STUN/TURN for the media
* server.
*
*
* STUN/TURN are needed only when the media server sits behind a NAT and needs to
* find out its own external IP address. However, if you set a static external IP
* address with this parameter, then there is no need for the STUN/TURN
* auto-discovery.
*
*
* The effect of this parameter is that ALL local ICE candidates that are
* gathered (for WebRTC) will contain the provided external IP address instead of
* the local one.
*
*
* externalAddress
is an IPv4 or IPv6 address.
*
* Examples:
*
* externalAddress=10.70.35.2
* externalAddress=2001:0db8:85a3:0000:0000:8a2e:0370:7334
*
*
*
**/
void setExternalAddress(@org.kurento.client.internal.server.Param("externalAddress") String externalAddress);
/**
*
* Set External (public) IP address of the media server.
*
* If you know what will be the external or public IP address of the media server
* (e.g. because your deployment has an static IP), you can specify it here.
* Doing so has the advantage of not needing to configure STUN/TURN for the media
* server.
*
*
* STUN/TURN are needed only when the media server sits behind a NAT and needs to
* find out its own external IP address. However, if you set a static external IP
* address with this parameter, then there is no need for the STUN/TURN
* auto-discovery.
*
*
* The effect of this parameter is that ALL local ICE candidates that are
* gathered (for WebRTC) will contain the provided external IP address instead of
* the local one.
*
*
* externalAddress
is an IPv4 or IPv6 address.
*
* Examples:
*
* externalAddress=10.70.35.2
* externalAddress=2001:0db8:85a3:0000:0000:8a2e:0370:7334
*
*
*
**/
void setExternalAddress(@org.kurento.client.internal.server.Param("externalAddress") String externalAddress, Continuation cont);
/**
*
* Set External (public) IP address of the media server.
*
* If you know what will be the external or public IP address of the media server
* (e.g. because your deployment has an static IP), you can specify it here.
* Doing so has the advantage of not needing to configure STUN/TURN for the media
* server.
*
*
* STUN/TURN are needed only when the media server sits behind a NAT and needs to
* find out its own external IP address. However, if you set a static external IP
* address with this parameter, then there is no need for the STUN/TURN
* auto-discovery.
*
*
* The effect of this parameter is that ALL local ICE candidates that are
* gathered (for WebRTC) will contain the provided external IP address instead of
* the local one.
*
*
* externalAddress
is an IPv4 or IPv6 address.
*
* Examples:
*
* externalAddress=10.70.35.2
* externalAddress=2001:0db8:85a3:0000:0000:8a2e:0370:7334
*
*
*
**/
void setExternalAddress(@org.kurento.client.internal.server.Param("externalAddress") String externalAddress, Transaction tx);
/**
*
* Get Local network interfaces used for ICE gathering.
*
* If you know which network interfaces should be used to perform ICE (for WebRTC
* connectivity), you can define them here. Doing so has several advantages:
*
*
* -
* The WebRTC ICE gathering process will be much quicker. Normally, it needs to
* gather local candidates for all of the network interfaces, but this step can
* be made faster if you limit it to only the interface that you know will
* work.
*
* -
* It will ensure that the media server always decides to use the correct
* network interface. With WebRTC ICE gathering it's possible that, under some
* circumstances (in systems with virtual network interfaces such as
*
docker0
) the ICE process ends up choosing the wrong local IP.
*
*
*
* networkInterfaces
is a comma-separated list of network interface
* names.
*
* Examples:
*
* networkInterfaces=eth0
* networkInterfaces=eth0,enp0s25
*
*
*
**/
String getNetworkInterfaces();
/**
*
* Get Local network interfaces used for ICE gathering.
*
* If you know which network interfaces should be used to perform ICE (for WebRTC
* connectivity), you can define them here. Doing so has several advantages:
*
*
* -
* The WebRTC ICE gathering process will be much quicker. Normally, it needs to
* gather local candidates for all of the network interfaces, but this step can
* be made faster if you limit it to only the interface that you know will
* work.
*
* -
* It will ensure that the media server always decides to use the correct
* network interface. With WebRTC ICE gathering it's possible that, under some
* circumstances (in systems with virtual network interfaces such as
*
docker0
) the ICE process ends up choosing the wrong local IP.
*
*
*
* networkInterfaces
is a comma-separated list of network interface
* names.
*
* Examples:
*
* networkInterfaces=eth0
* networkInterfaces=eth0,enp0s25
*
*
*
**/
void getNetworkInterfaces(Continuation cont);
/**
*
* Get Local network interfaces used for ICE gathering.
*
* If you know which network interfaces should be used to perform ICE (for WebRTC
* connectivity), you can define them here. Doing so has several advantages:
*
*
* -
* The WebRTC ICE gathering process will be much quicker. Normally, it needs to
* gather local candidates for all of the network interfaces, but this step can
* be made faster if you limit it to only the interface that you know will
* work.
*
* -
* It will ensure that the media server always decides to use the correct
* network interface. With WebRTC ICE gathering it's possible that, under some
* circumstances (in systems with virtual network interfaces such as
*
docker0
) the ICE process ends up choosing the wrong local IP.
*
*
*
* networkInterfaces
is a comma-separated list of network interface
* names.
*
* Examples:
*
* networkInterfaces=eth0
* networkInterfaces=eth0,enp0s25
*
*
*
**/
TFuture getNetworkInterfaces(Transaction tx);
/**
*
* Set Local network interfaces used for ICE gathering.
*
* If you know which network interfaces should be used to perform ICE (for WebRTC
* connectivity), you can define them here. Doing so has several advantages:
*
*
* -
* The WebRTC ICE gathering process will be much quicker. Normally, it needs to
* gather local candidates for all of the network interfaces, but this step can
* be made faster if you limit it to only the interface that you know will
* work.
*
* -
* It will ensure that the media server always decides to use the correct
* network interface. With WebRTC ICE gathering it's possible that, under some
* circumstances (in systems with virtual network interfaces such as
*
docker0
) the ICE process ends up choosing the wrong local IP.
*
*
*
* networkInterfaces
is a comma-separated list of network interface
* names.
*
* Examples:
*
* networkInterfaces=eth0
* networkInterfaces=eth0,enp0s25
*
*
*
**/
void setNetworkInterfaces(@org.kurento.client.internal.server.Param("networkInterfaces") String networkInterfaces);
/**
*
* Set Local network interfaces used for ICE gathering.
*
* If you know which network interfaces should be used to perform ICE (for WebRTC
* connectivity), you can define them here. Doing so has several advantages:
*
*
* -
* The WebRTC ICE gathering process will be much quicker. Normally, it needs to
* gather local candidates for all of the network interfaces, but this step can
* be made faster if you limit it to only the interface that you know will
* work.
*
* -
* It will ensure that the media server always decides to use the correct
* network interface. With WebRTC ICE gathering it's possible that, under some
* circumstances (in systems with virtual network interfaces such as
*
docker0
) the ICE process ends up choosing the wrong local IP.
*
*
*
* networkInterfaces
is a comma-separated list of network interface
* names.
*
* Examples:
*
* networkInterfaces=eth0
* networkInterfaces=eth0,enp0s25
*
*
*
**/
void setNetworkInterfaces(@org.kurento.client.internal.server.Param("networkInterfaces") String networkInterfaces, Continuation cont);
/**
*
* Set Local network interfaces used for ICE gathering.
*
* If you know which network interfaces should be used to perform ICE (for WebRTC
* connectivity), you can define them here. Doing so has several advantages:
*
*
* -
* The WebRTC ICE gathering process will be much quicker. Normally, it needs to
* gather local candidates for all of the network interfaces, but this step can
* be made faster if you limit it to only the interface that you know will
* work.
*
* -
* It will ensure that the media server always decides to use the correct
* network interface. With WebRTC ICE gathering it's possible that, under some
* circumstances (in systems with virtual network interfaces such as
*
docker0
) the ICE process ends up choosing the wrong local IP.
*
*
*
* networkInterfaces
is a comma-separated list of network interface
* names.
*
* Examples:
*
* networkInterfaces=eth0
* networkInterfaces=eth0,enp0s25
*
*
*
**/
void setNetworkInterfaces(@org.kurento.client.internal.server.Param("networkInterfaces") String networkInterfaces, Transaction tx);
/**
*
* Get STUN server IP address.
* The ICE process uses STUN to punch holes through NAT firewalls.
*
* stunServerAddress
MUST be an IP address; domain names are NOT
* supported.
*
*
* You need to use a well-working STUN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
).
*
*
*
**/
String getStunServerAddress();
/**
*
* Get STUN server IP address.
* The ICE process uses STUN to punch holes through NAT firewalls.
*
* stunServerAddress
MUST be an IP address; domain names are NOT
* supported.
*
*
* You need to use a well-working STUN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
).
*
*
*
**/
void getStunServerAddress(Continuation cont);
/**
*
* Get STUN server IP address.
* The ICE process uses STUN to punch holes through NAT firewalls.
*
* stunServerAddress
MUST be an IP address; domain names are NOT
* supported.
*
*
* You need to use a well-working STUN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
).
*
*
*
**/
TFuture getStunServerAddress(Transaction tx);
/**
*
* Set STUN server IP address.
* The ICE process uses STUN to punch holes through NAT firewalls.
*
* stunServerAddress
MUST be an IP address; domain names are NOT
* supported.
*
*
* You need to use a well-working STUN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
).
*
*
*
**/
void setStunServerAddress(@org.kurento.client.internal.server.Param("stunServerAddress") String stunServerAddress);
/**
*
* Set STUN server IP address.
* The ICE process uses STUN to punch holes through NAT firewalls.
*
* stunServerAddress
MUST be an IP address; domain names are NOT
* supported.
*
*
* You need to use a well-working STUN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
).
*
*
*
**/
void setStunServerAddress(@org.kurento.client.internal.server.Param("stunServerAddress") String stunServerAddress, Continuation cont);
/**
*
* Set STUN server IP address.
* The ICE process uses STUN to punch holes through NAT firewalls.
*
* stunServerAddress
MUST be an IP address; domain names are NOT
* supported.
*
*
* You need to use a well-working STUN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
).
*
*
*
**/
void setStunServerAddress(@org.kurento.client.internal.server.Param("stunServerAddress") String stunServerAddress, Transaction tx);
/**
*
* Get Port of the STUN server
*
**/
int getStunServerPort();
/**
*
* Get Port of the STUN server
*
**/
void getStunServerPort(Continuation cont);
/**
*
* Get Port of the STUN server
*
**/
TFuture getStunServerPort(Transaction tx);
/**
*
* Set Port of the STUN server
*
**/
void setStunServerPort(@org.kurento.client.internal.server.Param("stunServerPort") int stunServerPort);
/**
*
* Set Port of the STUN server
*
**/
void setStunServerPort(@org.kurento.client.internal.server.Param("stunServerPort") int stunServerPort, Continuation cont);
/**
*
* Set Port of the STUN server
*
**/
void setStunServerPort(@org.kurento.client.internal.server.Param("stunServerPort") int stunServerPort, Transaction tx);
/**
*
* Get TURN server URL.
*
* When STUN is not enough to open connections through some NAT firewalls, using
* TURN is the remaining alternative.
*
*
* Note that TURN is a superset of STUN, so you don't need to configure STUN if
* you are using TURN.
*
* The provided URL should follow one of these formats:
*
* user:password@ipaddress:port
* -
*
user:password@ipaddress:port?transport=[udp|tcp|tls]
*
*
*
* ipaddress
MUST be an IP address; domain names are NOT supported.
* transport
is OPTIONAL. Possible values: udp, tcp, tls. Default: udp.
*
*
* You need to use a well-working TURN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
) AND one Relay Candidate (type relay
).
*
*
*
**/
String getTurnUrl();
/**
*
* Get TURN server URL.
*
* When STUN is not enough to open connections through some NAT firewalls, using
* TURN is the remaining alternative.
*
*
* Note that TURN is a superset of STUN, so you don't need to configure STUN if
* you are using TURN.
*
* The provided URL should follow one of these formats:
*
* user:password@ipaddress:port
* -
*
user:password@ipaddress:port?transport=[udp|tcp|tls]
*
*
*
* ipaddress
MUST be an IP address; domain names are NOT supported.
* transport
is OPTIONAL. Possible values: udp, tcp, tls. Default: udp.
*
*
* You need to use a well-working TURN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
) AND one Relay Candidate (type relay
).
*
*
*
**/
void getTurnUrl(Continuation cont);
/**
*
* Get TURN server URL.
*
* When STUN is not enough to open connections through some NAT firewalls, using
* TURN is the remaining alternative.
*
*
* Note that TURN is a superset of STUN, so you don't need to configure STUN if
* you are using TURN.
*
* The provided URL should follow one of these formats:
*
* user:password@ipaddress:port
* -
*
user:password@ipaddress:port?transport=[udp|tcp|tls]
*
*
*
* ipaddress
MUST be an IP address; domain names are NOT supported.
* transport
is OPTIONAL. Possible values: udp, tcp, tls. Default: udp.
*
*
* You need to use a well-working TURN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
) AND one Relay Candidate (type relay
).
*
*
*
**/
TFuture getTurnUrl(Transaction tx);
/**
*
* Set TURN server URL.
*
* When STUN is not enough to open connections through some NAT firewalls, using
* TURN is the remaining alternative.
*
*
* Note that TURN is a superset of STUN, so you don't need to configure STUN if
* you are using TURN.
*
* The provided URL should follow one of these formats:
*
* user:password@ipaddress:port
* -
*
user:password@ipaddress:port?transport=[udp|tcp|tls]
*
*
*
* ipaddress
MUST be an IP address; domain names are NOT supported.
* transport
is OPTIONAL. Possible values: udp, tcp, tls. Default: udp.
*
*
* You need to use a well-working TURN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
) AND one Relay Candidate (type relay
).
*
*
*
**/
void setTurnUrl(@org.kurento.client.internal.server.Param("turnUrl") String turnUrl);
/**
*
* Set TURN server URL.
*
* When STUN is not enough to open connections through some NAT firewalls, using
* TURN is the remaining alternative.
*
*
* Note that TURN is a superset of STUN, so you don't need to configure STUN if
* you are using TURN.
*
* The provided URL should follow one of these formats:
*
* user:password@ipaddress:port
* -
*
user:password@ipaddress:port?transport=[udp|tcp|tls]
*
*
*
* ipaddress
MUST be an IP address; domain names are NOT supported.
* transport
is OPTIONAL. Possible values: udp, tcp, tls. Default: udp.
*
*
* You need to use a well-working TURN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
) AND one Relay Candidate (type relay
).
*
*
*
**/
void setTurnUrl(@org.kurento.client.internal.server.Param("turnUrl") String turnUrl, Continuation cont);
/**
*
* Set TURN server URL.
*
* When STUN is not enough to open connections through some NAT firewalls, using
* TURN is the remaining alternative.
*
*
* Note that TURN is a superset of STUN, so you don't need to configure STUN if
* you are using TURN.
*
* The provided URL should follow one of these formats:
*
* user:password@ipaddress:port
* -
*
user:password@ipaddress:port?transport=[udp|tcp|tls]
*
*
*
* ipaddress
MUST be an IP address; domain names are NOT supported.
* transport
is OPTIONAL. Possible values: udp, tcp, tls. Default: udp.
*
*
* You need to use a well-working TURN server. Use this to check if it works:
* https://webrtc.github.io/samples/src/content/peerconnection/trickle-ice/
* From that check, you should get at least one Server-Reflexive Candidate (type
* srflx
) AND one Relay Candidate (type relay
).
*
*
*
**/
void setTurnUrl(@org.kurento.client.internal.server.Param("turnUrl") String turnUrl, Transaction tx);
/**
*
* Get the ICE candidate pair (local and remote candidates) used by the ice library for each stream.
*
**/
java.util.List getICECandidatePairs();
/**
*
* Get the ICE candidate pair (local and remote candidates) used by the ice library for each stream.
*
**/
void getICECandidatePairs(Continuation> cont);
/**
*
* Get the ICE candidate pair (local and remote candidates) used by the ice library for each stream.
*
**/
TFuture> getICECandidatePairs(Transaction tx);
/**
*
* Get the ICE connection state for all the connections.
*
**/
java.util.List getIceConnectionState();
/**
*
* Get the ICE connection state for all the connections.
*
**/
void getIceConnectionState(Continuation> cont);
/**
*
* Get the ICE connection state for all the connections.
*
**/
TFuture> getIceConnectionState(Transaction tx);
/**
*
* Start the gathering of ICE candidates.
*
* It must be called after SdpEndpoint::generateOffer
or
* SdpEndpoint::processOffer
for Trickle ICE. If
* invoked before generating or processing an SDP offer, the candidates gathered
* will be added to the SDP processed.
*
*
*
**/
void gatherCandidates();
/**
*
* Asynchronous version of gatherCandidates:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see WebRtcEndpoint#gatherCandidates
*
**/
void gatherCandidates(Continuation cont);
/**
*
* Start the gathering of ICE candidates.
*
* It must be called after SdpEndpoint::generateOffer
or
* SdpEndpoint::processOffer
for Trickle ICE. If
* invoked before generating or processing an SDP offer, the candidates gathered
* will be added to the SDP processed.
*
*
*
**/
void gatherCandidates(Transaction tx);
/**
*
* Process an ICE candidate sent by the remote peer of the connection.
*
* @param candidate
* Remote ICE candidate
*
**/
void addIceCandidate(@org.kurento.client.internal.server.Param("candidate") org.kurento.client.IceCandidate candidate);
/**
*
* Asynchronous version of addIceCandidate:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see WebRtcEndpoint#addIceCandidate
*
* @param candidate
* Remote ICE candidate
*
**/
void addIceCandidate(@org.kurento.client.internal.server.Param("candidate") org.kurento.client.IceCandidate candidate, Continuation cont);
/**
*
* Process an ICE candidate sent by the remote peer of the connection.
*
* @param candidate
* Remote ICE candidate
*
**/
void addIceCandidate(Transaction tx, @org.kurento.client.internal.server.Param("candidate") org.kurento.client.IceCandidate candidate);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
* @param maxPacketLifeTime
* The time window (in milliseconds) during which transmissions and retransmissions may take place in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
* @param maxRetransmits
* maximum number of retransmissions that are attempted in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
* @param protocol
* Name of the subprotocol used for data communication
*
**/
void createDataChannel(@org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered, @org.kurento.client.internal.server.Param("maxPacketLifeTime") int maxPacketLifeTime, @org.kurento.client.internal.server.Param("maxRetransmits") int maxRetransmits, @org.kurento.client.internal.server.Param("protocol") String protocol);
/**
*
* Asynchronous version of createDataChannel:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see WebRtcEndpoint#createDataChannel
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
* @param maxPacketLifeTime
* The time window (in milliseconds) during which transmissions and retransmissions may take place in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
* @param maxRetransmits
* maximum number of retransmissions that are attempted in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
* @param protocol
* Name of the subprotocol used for data communication
*
**/
void createDataChannel(@org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered, @org.kurento.client.internal.server.Param("maxPacketLifeTime") int maxPacketLifeTime, @org.kurento.client.internal.server.Param("maxRetransmits") int maxRetransmits, @org.kurento.client.internal.server.Param("protocol") String protocol, Continuation cont);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
* @param maxPacketLifeTime
* The time window (in milliseconds) during which transmissions and retransmissions may take place in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
* @param maxRetransmits
* maximum number of retransmissions that are attempted in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
* @param protocol
* Name of the subprotocol used for data communication
*
**/
void createDataChannel(Transaction tx, @org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered, @org.kurento.client.internal.server.Param("maxPacketLifeTime") int maxPacketLifeTime, @org.kurento.client.internal.server.Param("maxRetransmits") int maxRetransmits, @org.kurento.client.internal.server.Param("protocol") String protocol);
/**
*
* Closes an open data channel
*
* @param channelId
* The channel identifier
*
**/
void closeDataChannel(@org.kurento.client.internal.server.Param("channelId") int channelId);
/**
*
* Asynchronous version of closeDataChannel:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see WebRtcEndpoint#closeDataChannel
*
* @param channelId
* The channel identifier
*
**/
void closeDataChannel(@org.kurento.client.internal.server.Param("channelId") int channelId, Continuation cont);
/**
*
* Closes an open data channel
*
* @param channelId
* The channel identifier
*
**/
void closeDataChannel(Transaction tx, @org.kurento.client.internal.server.Param("channelId") int channelId);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
**/
void createDataChannel();
/**
*
* Asynchronous version of createDataChannel:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see WebRtcEndpoint#createDataChannel
*
**/
void createDataChannel(Continuation cont);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
**/
void createDataChannel(Transaction tx);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
* @param label
* Channel's label
*
**/
void createDataChannel(@org.kurento.client.internal.server.Param("label") String label);
/**
*
* Asynchronous version of createDataChannel:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see WebRtcEndpoint#createDataChannel
*
* @param label
* Channel's label
*
**/
void createDataChannel(@org.kurento.client.internal.server.Param("label") String label, Continuation cont);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
* @param label
* Channel's label
*
**/
void createDataChannel(Transaction tx, @org.kurento.client.internal.server.Param("label") String label);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
*
**/
void createDataChannel(@org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered);
/**
*
* Asynchronous version of createDataChannel:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see WebRtcEndpoint#createDataChannel
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
*
**/
void createDataChannel(@org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered, Continuation cont);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
*
**/
void createDataChannel(Transaction tx, @org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
* @param maxPacketLifeTime
* The time window (in milliseconds) during which transmissions and retransmissions may take place in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
*
**/
void createDataChannel(@org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered, @org.kurento.client.internal.server.Param("maxPacketLifeTime") int maxPacketLifeTime);
/**
*
* Asynchronous version of createDataChannel:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see WebRtcEndpoint#createDataChannel
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
* @param maxPacketLifeTime
* The time window (in milliseconds) during which transmissions and retransmissions may take place in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
*
**/
void createDataChannel(@org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered, @org.kurento.client.internal.server.Param("maxPacketLifeTime") int maxPacketLifeTime, Continuation cont);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
* @param maxPacketLifeTime
* The time window (in milliseconds) during which transmissions and retransmissions may take place in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
*
**/
void createDataChannel(Transaction tx, @org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered, @org.kurento.client.internal.server.Param("maxPacketLifeTime") int maxPacketLifeTime);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
* @param maxPacketLifeTime
* The time window (in milliseconds) during which transmissions and retransmissions may take place in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
* @param maxRetransmits
* maximum number of retransmissions that are attempted in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
*
**/
void createDataChannel(@org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered, @org.kurento.client.internal.server.Param("maxPacketLifeTime") int maxPacketLifeTime, @org.kurento.client.internal.server.Param("maxRetransmits") int maxRetransmits);
/**
*
* Asynchronous version of createDataChannel:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see WebRtcEndpoint#createDataChannel
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
* @param maxPacketLifeTime
* The time window (in milliseconds) during which transmissions and retransmissions may take place in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
* @param maxRetransmits
* maximum number of retransmissions that are attempted in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
*
**/
void createDataChannel(@org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered, @org.kurento.client.internal.server.Param("maxPacketLifeTime") int maxPacketLifeTime, @org.kurento.client.internal.server.Param("maxRetransmits") int maxRetransmits, Continuation cont);
/**
*
* Create a new data channel, if data channels are supported.
*
* Being supported means that the WebRtcEndpoint has been created with data
* channel support, the client also supports data channels, and they have been
* negotiated in the SDP exchange. Otherwise, the method throws an exception,
* indicating that the operation is not possible.
*
*
* Data channels can work in either unreliable mode (analogous to User Datagram
* Protocol or UDP) or reliable mode (analogous to Transmission Control Protocol
* or TCP). The two modes have a simple distinction:
*
*
* -
* Reliable mode guarantees the transmission of messages and also the order in
* which they are delivered. This takes extra overhead, thus potentially making
* this mode slower.
*
* -
* Unreliable mode does not guarantee every message will get to the other side
* nor what order they get there. This removes the overhead, allowing this mode
* to work much faster.
*
*
* If data channels are not supported, this method throws an exception.
*
*
* @param label
* Channel's label
* @param ordered
* If the data channel should guarantee order or not. If true, and maxPacketLifeTime and maxRetransmits have not been provided, reliable mode is activated.
* @param maxPacketLifeTime
* The time window (in milliseconds) during which transmissions and retransmissions may take place in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
* @param maxRetransmits
* maximum number of retransmissions that are attempted in unreliable mode.
* Note that this forces unreliable mode, even if ordered
has been activated.
*
*
**/
void createDataChannel(Transaction tx, @org.kurento.client.internal.server.Param("label") String label, @org.kurento.client.internal.server.Param("ordered") boolean ordered, @org.kurento.client.internal.server.Param("maxPacketLifeTime") int maxPacketLifeTime, @org.kurento.client.internal.server.Param("maxRetransmits") int maxRetransmits);
/**
* Add a {@link EventListener} for event {@link OnIceCandidateEvent}. Synchronous call.
*
* @param listener Listener to be called on OnIceCandidateEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceCandidateEvent.class)
ListenerSubscription addOnIceCandidateListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link OnIceCandidateEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on OnIceCandidateEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceCandidateEvent.class)
void addOnIceCandidateListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link OnIceCandidateEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceCandidateEvent.class)
void removeOnIceCandidateListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link OnIceCandidateEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceCandidateEvent.class)
void removeOnIceCandidateListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link IceCandidateFoundEvent}. Synchronous call.
*
* @param listener Listener to be called on IceCandidateFoundEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(IceCandidateFoundEvent.class)
ListenerSubscription addIceCandidateFoundListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link IceCandidateFoundEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on IceCandidateFoundEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(IceCandidateFoundEvent.class)
void addIceCandidateFoundListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link IceCandidateFoundEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(IceCandidateFoundEvent.class)
void removeIceCandidateFoundListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link IceCandidateFoundEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(IceCandidateFoundEvent.class)
void removeIceCandidateFoundListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link OnIceGatheringDoneEvent}. Synchronous call.
*
* @param listener Listener to be called on OnIceGatheringDoneEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceGatheringDoneEvent.class)
ListenerSubscription addOnIceGatheringDoneListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link OnIceGatheringDoneEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on OnIceGatheringDoneEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceGatheringDoneEvent.class)
void addOnIceGatheringDoneListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link OnIceGatheringDoneEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceGatheringDoneEvent.class)
void removeOnIceGatheringDoneListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link OnIceGatheringDoneEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceGatheringDoneEvent.class)
void removeOnIceGatheringDoneListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link IceGatheringDoneEvent}. Synchronous call.
*
* @param listener Listener to be called on IceGatheringDoneEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(IceGatheringDoneEvent.class)
ListenerSubscription addIceGatheringDoneListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link IceGatheringDoneEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on IceGatheringDoneEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(IceGatheringDoneEvent.class)
void addIceGatheringDoneListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link IceGatheringDoneEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(IceGatheringDoneEvent.class)
void removeIceGatheringDoneListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link IceGatheringDoneEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(IceGatheringDoneEvent.class)
void removeIceGatheringDoneListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link OnIceComponentStateChangedEvent}. Synchronous call.
*
* @param listener Listener to be called on OnIceComponentStateChangedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceComponentStateChangedEvent.class)
ListenerSubscription addOnIceComponentStateChangedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link OnIceComponentStateChangedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on OnIceComponentStateChangedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceComponentStateChangedEvent.class)
void addOnIceComponentStateChangedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link OnIceComponentStateChangedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceComponentStateChangedEvent.class)
void removeOnIceComponentStateChangedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link OnIceComponentStateChangedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(OnIceComponentStateChangedEvent.class)
void removeOnIceComponentStateChangedListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link IceComponentStateChangeEvent}. Synchronous call.
*
* @param listener Listener to be called on IceComponentStateChangeEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(IceComponentStateChangeEvent.class)
ListenerSubscription addIceComponentStateChangeListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link IceComponentStateChangeEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on IceComponentStateChangeEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(IceComponentStateChangeEvent.class)
void addIceComponentStateChangeListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link IceComponentStateChangeEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(IceComponentStateChangeEvent.class)
void removeIceComponentStateChangeListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link IceComponentStateChangeEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(IceComponentStateChangeEvent.class)
void removeIceComponentStateChangeListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link OnDataChannelOpenedEvent}. Synchronous call.
*
* @param listener Listener to be called on OnDataChannelOpenedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(OnDataChannelOpenedEvent.class)
ListenerSubscription addOnDataChannelOpenedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link OnDataChannelOpenedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on OnDataChannelOpenedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(OnDataChannelOpenedEvent.class)
void addOnDataChannelOpenedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link OnDataChannelOpenedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(OnDataChannelOpenedEvent.class)
void removeOnDataChannelOpenedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link OnDataChannelOpenedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(OnDataChannelOpenedEvent.class)
void removeOnDataChannelOpenedListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link DataChannelOpenEvent}. Synchronous call.
*
* @param listener Listener to be called on DataChannelOpenEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(DataChannelOpenEvent.class)
ListenerSubscription addDataChannelOpenListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link DataChannelOpenEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on DataChannelOpenEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(DataChannelOpenEvent.class)
void addDataChannelOpenListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link DataChannelOpenEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(DataChannelOpenEvent.class)
void removeDataChannelOpenListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link DataChannelOpenEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(DataChannelOpenEvent.class)
void removeDataChannelOpenListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link OnDataChannelClosedEvent}. Synchronous call.
*
* @param listener Listener to be called on OnDataChannelClosedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(OnDataChannelClosedEvent.class)
ListenerSubscription addOnDataChannelClosedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link OnDataChannelClosedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on OnDataChannelClosedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(OnDataChannelClosedEvent.class)
void addOnDataChannelClosedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link OnDataChannelClosedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(OnDataChannelClosedEvent.class)
void removeOnDataChannelClosedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link OnDataChannelClosedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(OnDataChannelClosedEvent.class)
void removeOnDataChannelClosedListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link DataChannelCloseEvent}. Synchronous call.
*
* @param listener Listener to be called on DataChannelCloseEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(DataChannelCloseEvent.class)
ListenerSubscription addDataChannelCloseListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link DataChannelCloseEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on DataChannelCloseEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(DataChannelCloseEvent.class)
void addDataChannelCloseListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link DataChannelCloseEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(DataChannelCloseEvent.class)
void removeDataChannelCloseListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link DataChannelCloseEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(DataChannelCloseEvent.class)
void removeDataChannelCloseListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link NewCandidatePairSelectedEvent}. Synchronous call.
*
* @param listener Listener to be called on NewCandidatePairSelectedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(NewCandidatePairSelectedEvent.class)
ListenerSubscription addNewCandidatePairSelectedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link NewCandidatePairSelectedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on NewCandidatePairSelectedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(NewCandidatePairSelectedEvent.class)
void addNewCandidatePairSelectedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link NewCandidatePairSelectedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(NewCandidatePairSelectedEvent.class)
void removeNewCandidatePairSelectedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link NewCandidatePairSelectedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(NewCandidatePairSelectedEvent.class)
void removeNewCandidatePairSelectedListener(ListenerSubscription listenerSubscription, Continuation cont);
public class Builder extends AbstractBuilder {
/**
*
* Creates a Builder for WebRtcEndpoint
*
**/
public Builder(org.kurento.client.MediaPipeline mediaPipeline){
super(WebRtcEndpoint.class,mediaPipeline);
props.add("mediaPipeline",mediaPipeline);
}
public Builder withProperties(Properties properties) {
return (Builder)super.withProperties(properties);
}
public Builder with(String name, Object value) {
return (Builder)super.with(name, value);
}
/**
*
* Single direction, receive-only endpoint
*
**/
public Builder recvonly(){
props.add("recvonly",Boolean.TRUE);
return this;
}
/**
*
* Single direction, send-only endpoint
*
**/
public Builder sendonly(){
props.add("sendonly",Boolean.TRUE);
return this;
}
/**
*
* Activate data channels support
*
**/
public Builder useDataChannels(){
props.add("useDataChannels",Boolean.TRUE);
return this;
}
/**
*
* Sets a value for certificateKeyType in Builder for WebRtcEndpoint.
*
* @param certificateKeyType
* Define the type of the certificate used in dtls
*
**/
public Builder withCertificateKeyType(org.kurento.client.CertificateKeyType certificateKeyType){
props.add("certificateKeyType",certificateKeyType);
return this;
}
}
}