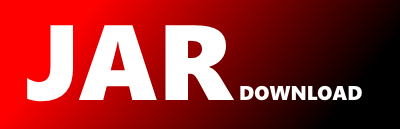
org.kurento.client.BaseRtpEndpoint Maven / Gradle / Ivy
Show all versions of kurento-client Show documentation
/**
* This file is generated with Kurento-maven-plugin.
* Please don't edit.
*/
package org.kurento.client;
/**
*
* Handles RTP communications.
*
* All endpoints that rely on the RTP protocol, like the
* RtpEndpoint or the WebRtcEndpoint, inherit
* from this class. The endpoint provides information about the connection state
* and the media state, which can be consulted at any time through the
* {@link #mediaState} and the {@link #connectionState} properties. It is
* also possible subscribe to events fired when these properties change.
*
*
* -
* ConnectionStateChangedEvent: This event is raised when the
* connection between two peers changes. It can have two values:
*
* - CONNECTED
* - DISCONNECTED
*
*
* -
* MediaStateChangedEvent: This event provides information
* about the state of the underlying RTP session.
*
* The standard definition of RTP (RFC 3550) describes a session as active whenever there is a maintained flow of
* RTCP control packets, regardless of whether there is actual media flowing
* through RTP data packets or not. The reasoning behind this is that, at any
* given moment, a participant of an RTP session might temporarily stop
* sending RTP data packets, but this wouldn't necessarily mean that the RTP
* session as a whole is finished; it maybe just means that the participant
* has some temporary issues but it will soon resume sending data. For this
* reason, that an RTP session has really finished is something that is
* considered only by the prolonged absence of RTCP control packets between
* participants.
*
*
* Since RTCP packets do not flow at a constant rate (for instance,
* minimizing a browser window with a WebRTC's
* RTCPeerConnection
object might affect the sending interval),
* it is not possible to immediately detect their absence and assume that the
* RTP session has finished. Instead, there is a guard period of
* approximately 5 seconds of missing RTCP packets before
* considering that the underlying RTP session is effectively finished, thus
* triggering a MediaStateChangedEvent = DISCONNECTED
event.
*
*
* In other words, there is always a period during which there might be no
* media flowing, but this event hasn't been fired yet. Nevertheless, this is
* the most reliable and useful way of knowing what is the long-term, steady
* state of RTP media exchange.
*
*
* The ConnectionStateChangedEvent
comes in contrast with more
* instantaneous events such as MediaElement's
* {@link #MediaFlowInStateChange} and
* {@link #MediaFlowOutStateChange}, which are triggered almost
* immediately after the RTP data packets stop flowing between RTP session
* participants. This makes the MediaFlow events a good way to
* know if participants are suffering from short-term intermittent
* connectivity issues, but they are not enough to know if the connectivity
* issues are just spurious network hiccups or are part of a more long-term
* disconnection problem.
*
*
* Possible values are:
*
*
* - CONNECTED: There is an RTCP packet flow between peers.
* -
* DISCONNECTED: Either no RTCP packets have been received yet, or the
* remote peer has ended the RTP session with a
BYE
message,
* or at least 5 seconds have elapsed since the last RTCP packet was
* received.
*
*
*
*
*
* Part of the bandwidth control for the video component of the media session is
* done here:
*
*
* -
* Input bandwidth: Values used to inform remote peers about the bitrate that
* can be sent to this endpoint.
*
* -
* MinVideoRecvBandwidth: Minimum input bitrate, requested
* from WebRTC senders with REMB (Default: 30 Kbps).
*
* -
* MaxAudioRecvBandwidth and
* MaxVideoRecvBandwidth: Maximum input bitrate, signaled
* in SDP Offers to WebRTC and RTP senders (Default: unlimited).
*
*
*
* -
* Output bandwidth: Values used to control bitrate of the video streams sent
* to remote peers. It is important to keep in mind that pushed bitrate depends
* on network and remote peer capabilities. Remote peers can also announce
* bandwidth limitation in their SDPs (through the
*
b={modifier}:{value}
attribute). Kurento will always enforce
* bitrate limitations specified by the remote peer over internal
* configurations.
*
* -
* MinVideoSendBandwidth: REMB override of minimum bitrate
* sent to WebRTC receivers (Default: 100 Kbps).
*
* -
* MaxVideoSendBandwidth: REMB override of maximum bitrate
* sent to WebRTC receivers (Default: 500 Kbps).
*
* -
* RembParams.rembOnConnect: Initial local REMB bandwidth
* estimation that gets propagated when a new endpoint is connected.
*
*
*
*
*
*
* All bandwidth control parameters must be changed before the SDP negotiation
* takes place, and can't be changed afterwards.
*
*
*
*
**/
@org.kurento.client.internal.RemoteClass
public interface BaseRtpEndpoint extends SdpEndpoint {
/**
*
* Get Minimum input bitrate, requested from WebRTC senders with REMB.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
int getMinVideoRecvBandwidth();
/**
*
* Get Minimum input bitrate, requested from WebRTC senders with REMB.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
void getMinVideoRecvBandwidth(Continuation cont);
/**
*
* Get Minimum input bitrate, requested from WebRTC senders with REMB.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
TFuture getMinVideoRecvBandwidth(Transaction tx);
/**
*
* Set Minimum input bitrate, requested from WebRTC senders with REMB.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
void setMinVideoRecvBandwidth(@org.kurento.client.internal.server.Param("minVideoRecvBandwidth") int minVideoRecvBandwidth);
/**
*
* Set Minimum input bitrate, requested from WebRTC senders with REMB.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
void setMinVideoRecvBandwidth(@org.kurento.client.internal.server.Param("minVideoRecvBandwidth") int minVideoRecvBandwidth, Continuation cont);
/**
*
* Set Minimum input bitrate, requested from WebRTC senders with REMB.
*
* This is used to set a minimum value of local REMB during bandwidth estimation,
* if supported by the implementing class. The REMB estimation will then be sent
* to remote peers, requesting them to send at least the indicated video bitrate.
* It follows that min values will only have effect in remote peers that support
* this congestion control mechanism, such as Chrome.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 0.
* -
* Note: The absolute minimum REMB value is 30 kbps, even if a lower value is
* set here.
*
*
*
*
**/
void setMinVideoRecvBandwidth(@org.kurento.client.internal.server.Param("minVideoRecvBandwidth") int minVideoRecvBandwidth, Transaction tx);
/**
*
* Get REMB override of minimum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unlimited: the video bitrate will drop as needed, even to the lowest
* possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
int getMinVideoSendBandwidth();
/**
*
* Get REMB override of minimum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unlimited: the video bitrate will drop as needed, even to the lowest
* possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
void getMinVideoSendBandwidth(Continuation cont);
/**
*
* Get REMB override of minimum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unlimited: the video bitrate will drop as needed, even to the lowest
* possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
TFuture getMinVideoSendBandwidth(Transaction tx);
/**
*
* Set REMB override of minimum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unlimited: the video bitrate will drop as needed, even to the lowest
* possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
void setMinVideoSendBandwidth(@org.kurento.client.internal.server.Param("minVideoSendBandwidth") int minVideoSendBandwidth);
/**
*
* Set REMB override of minimum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unlimited: the video bitrate will drop as needed, even to the lowest
* possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
void setMinVideoSendBandwidth(@org.kurento.client.internal.server.Param("minVideoSendBandwidth") int minVideoSendBandwidth, Continuation cont);
/**
*
* Set REMB override of minimum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the minimum video quality that will be
* sent when reacting to bad network conditions. Setting this parameter to a low
* value permits the video quality to drop when the network conditions get worse.
*
*
* This parameter provides a way to override the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or greater than
* this parameter, even if the remote peer requests even lower bitrates.
*
*
* Note that if you set this parameter too high (trying to avoid bad video
* quality altogether), you would be limiting the adaptation ability of the
* congestion control algorithm, and your stream might be unable to ever recover
* from adverse network conditions.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 100.
* -
* 0 = unlimited: the video bitrate will drop as needed, even to the lowest
* possible quality, which might make the video completely blurry and
* pixelated.
*
*
*
*
**/
void setMinVideoSendBandwidth(@org.kurento.client.internal.server.Param("minVideoSendBandwidth") int minVideoSendBandwidth, Transaction tx);
/**
*
* Get REMB override of maximum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than this
* parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this to higher values such as 2000 kbps (2
* mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unlimited: the video bitrate will grow until all the available network
* bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
int getMaxVideoSendBandwidth();
/**
*
* Get REMB override of maximum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than this
* parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this to higher values such as 2000 kbps (2
* mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unlimited: the video bitrate will grow until all the available network
* bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
void getMaxVideoSendBandwidth(Continuation cont);
/**
*
* Get REMB override of maximum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than this
* parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this to higher values such as 2000 kbps (2
* mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unlimited: the video bitrate will grow until all the available network
* bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
TFuture getMaxVideoSendBandwidth(Transaction tx);
/**
*
* Set REMB override of maximum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than this
* parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this to higher values such as 2000 kbps (2
* mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unlimited: the video bitrate will grow until all the available network
* bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
void setMaxVideoSendBandwidth(@org.kurento.client.internal.server.Param("maxVideoSendBandwidth") int maxVideoSendBandwidth);
/**
*
* Set REMB override of maximum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than this
* parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this to higher values such as 2000 kbps (2
* mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unlimited: the video bitrate will grow until all the available network
* bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
void setMaxVideoSendBandwidth(@org.kurento.client.internal.server.Param("maxVideoSendBandwidth") int maxVideoSendBandwidth, Continuation cont);
/**
*
* Set REMB override of maximum bitrate sent to WebRTC receivers.
*
* With this parameter you can control the maximum video quality that will be
* sent when reacting to good network conditions. Setting this parameter to a
* high value permits the video quality to raise when the network conditions get
* better.
*
*
* This parameter provides a way to limit the bitrate requested by remote REMB
* bandwidth estimations: the bitrate sent will be always equal or less than this
* parameter, even if the remote peer requests higher bitrates.
*
*
* Note that the default value of 500 kbps is a VERY
* conservative one, and leads to a low maximum video quality. Most applications
* will probably want to increase this to higher values such as 2000 kbps (2
* mbps).
*
*
* The REMB congestion control algorithm works by gradually increasing the output
* video bitrate, until the available bandwidth is fully used or the maximum send
* bitrate has been reached. This is a slow, progressive change, which starts at
* 300 kbps by default. You can change the default starting point of REMB
* estimations, by setting RembParams.rembOnConnect
.
*
*
* - Unit: kbps (kilobits per second).
* - Default: 500.
* -
* 0 = unlimited: the video bitrate will grow until all the available network
* bandwidth is used by the stream.
* Note that this might have a bad effect if more than one stream is running
* (as all of them would try to raise the video bitrate indefinitely, until the
* network gets saturated).
*
*
*
*
**/
void setMaxVideoSendBandwidth(@org.kurento.client.internal.server.Param("maxVideoSendBandwidth") int maxVideoSendBandwidth, Transaction tx);
/**
*
* Get Media flow state.
*
* - CONNECTED: There is an RTCP flow.
* - DISCONNECTED: No RTCP packets have been received for at least 5 sec.
*
*
*
**/
org.kurento.client.MediaState getMediaState();
/**
*
* Get Media flow state.
*
* - CONNECTED: There is an RTCP flow.
* - DISCONNECTED: No RTCP packets have been received for at least 5 sec.
*
*
*
**/
void getMediaState(Continuation cont);
/**
*
* Get Media flow state.
*
* - CONNECTED: There is an RTCP flow.
* - DISCONNECTED: No RTCP packets have been received for at least 5 sec.
*
*
*
**/
TFuture getMediaState(Transaction tx);
/**
*
* Get Connection state.
*
* - CONNECTED
* - DISCONNECTED
*
*
*
**/
org.kurento.client.ConnectionState getConnectionState();
/**
*
* Get Connection state.
*
* - CONNECTED
* - DISCONNECTED
*
*
*
**/
void getConnectionState(Continuation cont);
/**
*
* Get Connection state.
*
* - CONNECTED
* - DISCONNECTED
*
*
*
**/
TFuture getConnectionState(Transaction tx);
/**
*
* Get Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
int getMtu();
/**
*
* Get Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
void getMtu(Continuation cont);
/**
*
* Get Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
TFuture getMtu(Transaction tx);
/**
*
* Set Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
void setMtu(@org.kurento.client.internal.server.Param("mtu") int mtu);
/**
*
* Set Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
void setMtu(@org.kurento.client.internal.server.Param("mtu") int mtu, Continuation cont);
/**
*
* Set Maximum Transmission Unit (MTU) used for RTP.
*
* This setting affects the maximum size that will be used by RTP payloads. You
* can change it from the default, if you think that a different value would be
* beneficial for the typical network settings of your application.
*
*
* The default value is 1200 Bytes. This is the same as in libwebrtc (from
* webrtc.org), as used by
* Firefox
* or
* Chrome
* . You can read more about this value in
* Why RTP max packet size is 1200 in WebRTC?
* .
*
*
* WARNING: Change this value ONLY if you really know what you are doing
* and you have strong reasons to do so. Do NOT change this parameter just
* because it seems to work better for some reduced scope tests. The
* default value is a consensus chosen by people who have deep knowledge about
* network optimization.
*
*
* - Unit: Bytes.
* - Default: 1200.
*
*
*
**/
void setMtu(@org.kurento.client.internal.server.Param("mtu") int mtu, Transaction tx);
/**
*
* Get Advanced parameters to configure the congestion control algorithm.
*
**/
org.kurento.client.RembParams getRembParams();
/**
*
* Get Advanced parameters to configure the congestion control algorithm.
*
**/
void getRembParams(Continuation cont);
/**
*
* Get Advanced parameters to configure the congestion control algorithm.
*
**/
TFuture getRembParams(Transaction tx);
/**
*
* Set Advanced parameters to configure the congestion control algorithm.
*
**/
void setRembParams(@org.kurento.client.internal.server.Param("rembParams") org.kurento.client.RembParams rembParams);
/**
*
* Set Advanced parameters to configure the congestion control algorithm.
*
**/
void setRembParams(@org.kurento.client.internal.server.Param("rembParams") org.kurento.client.RembParams rembParams, Continuation cont);
/**
*
* Set Advanced parameters to configure the congestion control algorithm.
*
**/
void setRembParams(@org.kurento.client.internal.server.Param("rembParams") org.kurento.client.RembParams rembParams, Transaction tx);
/**
* Add a {@link EventListener} for event {@link MediaStateChangedEvent}. Synchronous call.
*
* @param listener Listener to be called on MediaStateChangedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(MediaStateChangedEvent.class)
ListenerSubscription addMediaStateChangedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link MediaStateChangedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on MediaStateChangedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(MediaStateChangedEvent.class)
void addMediaStateChangedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link MediaStateChangedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(MediaStateChangedEvent.class)
void removeMediaStateChangedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link MediaStateChangedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(MediaStateChangedEvent.class)
void removeMediaStateChangedListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link ConnectionStateChangedEvent}. Synchronous call.
*
* @param listener Listener to be called on ConnectionStateChangedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(ConnectionStateChangedEvent.class)
ListenerSubscription addConnectionStateChangedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link ConnectionStateChangedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on ConnectionStateChangedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(ConnectionStateChangedEvent.class)
void addConnectionStateChangedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link ConnectionStateChangedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(ConnectionStateChangedEvent.class)
void removeConnectionStateChangedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link ConnectionStateChangedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(ConnectionStateChangedEvent.class)
void removeConnectionStateChangedListener(ListenerSubscription listenerSubscription, Continuation cont);
}