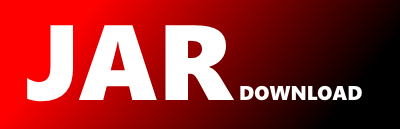
org.kurento.client.RecorderEndpoint Maven / Gradle / Ivy
Show all versions of kurento-client Show documentation
/**
* This file is generated with Kurento-maven-plugin.
* Please don't edit.
*/
package org.kurento.client;
/**
*
* Provides functionality to store media contents.
*
* RecorderEndpoint can store media into local files or send it to a remote
* network storage. When another {@link module:core/abstracts.MediaElement MediaElement} is connected to a
* RecorderEndpoint, the media coming from the former will be encapsulated into
* the selected recording format and stored in the designated location.
*
*
* These parameters must be provided to create a RecorderEndpoint, and they
* cannot be changed afterwards:
*
*
* -
* Destination URI, where media will be stored. These formats
* are supported:
*
* -
* File: A file path that will be written into the local file system.
* Example:
*
* file:///path/to/file
*
*
* -
* HTTP: A POST request will be used against a remote server. The server
* must support using the chunked encoding mode (HTTP header
*
Transfer-Encoding: chunked
). Examples:
*
* http(s)://{server-ip}/path/to/file
* -
*
* http(s)://{username}:{password}@{server-ip}:{server-port}/path/to/file
*
*
*
*
* -
* Relative URIs (with no schema) are supported. They are completed by
* prepending a default URI defined by property defaultPath. This
* property is defined in the configuration file
* /etc/kurento/modules/kurento/UriEndpoint.conf.ini, and the
* default value is
file:///var/lib/kurento/
*
* -
*
* NOTE (for current versions of Kurento 6.x): special characters are not
* supported in
{username}
or {password}
.
*
* This means that {username}
cannot contain colons
* (:
), and {password}
cannot contain 'at' signs
* (@
). This is a limitation of GStreamer 1.8 (the underlying
* media framework behind Kurento), and is already fixed in newer versions
* (which the upcoming Kurento 7.x will use).
*
* -
*
* NOTE (for upcoming Kurento 7.x): special characters in
*
{username}
or {password}
must be
* url-encoded.
*
* This means that colons (:
) should be replaced with
* %3A
, and 'at' signs (@
) should be replaced
* with %40
.
*
*
*
* -
* Media Profile ({@link #MediaProfileSpecType}), used for
* storage. This will determine the video and audio encoding. See below for
* more details about Media Profile.
*
* -
* EndOfStream (optional), a parameter that dictates if the
* recording should be automatically stopped once the EOS event is detected.
*
*
*
* Note that
*
* RecorderEndpoint requires write permissions to the destination
*
* ; otherwise, the media server won't be able to store any information, and an
* {@link ErrorEvent} will be fired. Make sure your application subscribes to this
* event, otherwise troubleshooting issues will be difficult.
*
*
* -
* To write local files (if you use
file://
), the system user that
* is owner of the media server process needs to have write permissions for the
* requested path. By default, this user is named 'kurento
'.
*
* -
* To record through HTTP, the remote server must be accessible through the
* network, and also have the correct write permissions for the destination
* path.
*
*
*
* The Media Profile is quite an important parameter, as it will
* determine whether the server needs to perform on-the-fly transcoding of the
* media. If the input stream codec if not compatible with the selected media
* profile, the media will be transcoded into a suitable format. This will result
* in a higher CPU load and will impact overall performance of the media server.
*
*
* For example: If your pipeline receives VP8-encoded video from WebRTC,
* and sends it to a RecorderEndpoint; depending on the format selected...
*
*
* -
* WEBM: The input codec is the same as the recording format, so no transcoding
* will take place.
*
* -
* MP4: The media server will have to transcode from VP8 to H264.
* This will raise the CPU load in the system.
*
* -
* MKV: Again, video must be transcoded from VP8 to H264, which
* means more CPU load.
*
*
*
* From this you can see how selecting the correct format for your application is
* a very important decision.
*
*
* Recording will start as soon as the user invokes the
* record
method. The recorder will then store, in the location
* indicated, the media that the source is sending to the endpoint. If no media
* is being received, or no endpoint has been connected, then the destination
* will be empty. The recorder starts storing information into the file as soon
* as it gets it.
*
*
* Recording must be stopped when no more data should be stored.
* This can be with the stopAndWait
method, which blocks and returns
* only after all the information was stored correctly.
*
*
*
* If your output file is empty, this means that the recorder is waiting for
* input media.
*
* When another endpoint is connected to the recorder, by default both AUDIO and
* VIDEO media types are expected, unless specified otherwise when invoking the
* connect
method. Failing to provide both types, will result in the
* RecorderEndpoint buffering the received media: it won't be written to the file
* until the recording is stopped. The recorder waits until all types of media
* start arriving, in order to synchronize them appropriately.
*
*
* The source endpoint can be hot-swapped while the recording is taking place.
* The recorded file will then contain different feeds. When switching video
* sources, if the new video has different size, the recorder will retain the
* size of the previous source. If the source is disconnected, the last frame
* recorded will be shown for the duration of the disconnection, or until the
* recording is stopped.
*
*
*
* It is recommended to start recording only after media arrives.
*
* For this, you may use the MediaFlowInStateChange
and
* MediaFlowOutStateChange
* events of your endpoints, and synchronize the recording with the moment media
* comes into the Recorder. For example:
*
*
* -
* When the remote video arrives to KMS, your WebRtcEndpoint will start
* generating packets into the Kurento Pipeline, and it will trigger a
*
MediaFlowOutStateChange
event.
*
* -
* When video packets arrive from the WebRtcEndpoint to the RecorderEndpoint,
* the RecorderEndpoint will raise a
MediaFlowInStateChange
event.
*
* -
* You should only start recording when RecorderEndpoint has notified a
*
MediaFlowInStateChange
for ALL streams (so, if you record
* AUDIO+VIDEO, your application must receive a
* MediaFlowInStateChange
event for audio, and another
* MediaFlowInStateChange
event for video).
*
*
*
*
**/
@org.kurento.client.internal.RemoteClass
public interface RecorderEndpoint extends UriEndpoint {
/**
*
* Starts storing media received through the sink pad.
*
**/
void record();
/**
*
* Asynchronous version of record:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see RecorderEndpoint#record
*
**/
void record(Continuation cont);
/**
*
* Starts storing media received through the sink pad.
*
**/
void record(Transaction tx);
/**
*
* Stops recording and does not return until all the content has been written to the selected uri. This can cause timeouts on some clients if there is too much content to write, or the transport is slow
*
**/
void stopAndWait();
/**
*
* Asynchronous version of stopAndWait:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see RecorderEndpoint#stopAndWait
*
**/
void stopAndWait(Continuation cont);
/**
*
* Stops recording and does not return until all the content has been written to the selected uri. This can cause timeouts on some clients if there is too much content to write, or the transport is slow
*
**/
void stopAndWait(Transaction tx);
/**
* Add a {@link EventListener} for event {@link RecordingEvent}. Synchronous call.
*
* @param listener Listener to be called on RecordingEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(RecordingEvent.class)
ListenerSubscription addRecordingListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link RecordingEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on RecordingEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(RecordingEvent.class)
void addRecordingListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link RecordingEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(RecordingEvent.class)
void removeRecordingListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link RecordingEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(RecordingEvent.class)
void removeRecordingListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link PausedEvent}. Synchronous call.
*
* @param listener Listener to be called on PausedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(PausedEvent.class)
ListenerSubscription addPausedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link PausedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on PausedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(PausedEvent.class)
void addPausedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link PausedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(PausedEvent.class)
void removePausedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link PausedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(PausedEvent.class)
void removePausedListener(ListenerSubscription listenerSubscription, Continuation cont);
/**
* Add a {@link EventListener} for event {@link StoppedEvent}. Synchronous call.
*
* @param listener Listener to be called on StoppedEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(StoppedEvent.class)
ListenerSubscription addStoppedListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link StoppedEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on StoppedEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(StoppedEvent.class)
void addStoppedListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link StoppedEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(StoppedEvent.class)
void removeStoppedListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link StoppedEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(StoppedEvent.class)
void removeStoppedListener(ListenerSubscription listenerSubscription, Continuation cont);
public class Builder extends AbstractBuilder {
/**
*
* Creates a Builder for RecorderEndpoint
*
**/
public Builder(org.kurento.client.MediaPipeline mediaPipeline, String uri){
super(RecorderEndpoint.class,mediaPipeline);
props.add("mediaPipeline",mediaPipeline);
props.add("uri",uri);
}
public Builder withProperties(Properties properties) {
return (Builder)super.withProperties(properties);
}
public Builder with(String name, Object value) {
return (Builder)super.with(name, value);
}
/**
*
* Sets a value for mediaProfile in Builder for RecorderEndpoint.
*
* @param mediaProfile
* Sets the media profile used for recording. If the profile is different than the one being received at the sink pad, media will be transcoded, resulting in a higher CPU load. For instance, when recording a VP8 encoded video from a WebRTC endpoint in MP4, the load is higher that when recording to WEBM.
*
**/
public Builder withMediaProfile(org.kurento.client.MediaProfileSpecType mediaProfile){
props.add("mediaProfile",mediaProfile);
return this;
}
/**
*
* Forces the recorder endpoint to finish processing data when an EOS is detected in the stream
*
**/
public Builder stopOnEndOfStream(){
props.add("stopOnEndOfStream",Boolean.TRUE);
return this;
}
}
}