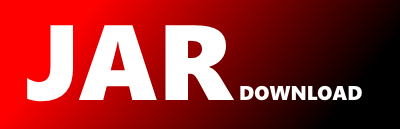
org.kurento.client.MediaObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kurento-client Show documentation
Show all versions of kurento-client Show documentation
Kurento Client
The Kurento Client project allows server applications to control media server resources.
/**
* This file is generated with Kurento-maven-plugin.
* Please don't edit.
*/
package org.kurento.client;
/**
*
* Base interface used to manage capabilities common to all Kurento elements. This includes both: {@link module:core/abstracts.MediaElement MediaElement} and {@link module:core.MediaPipeline MediaPipeline}
* Properties
*
* - id: unique identifier assigned to this
MediaObject
at instantiation time. {@link module:core.MediaPipeline MediaPipeline} IDs are generated with a GUID followed by suffix _kurento.MediaPipeline
. {@link module:core/abstracts.MediaElement MediaElement} IDs are also a GUID with suffix _kurento.elemenType
and prefixed by parent's ID.
*
*
* - MediaPipeline ID example
* 907cac3a-809a-4bbe-a93e-ae7e944c5cae_kurento.MediaPipeline
* - MediaElement ID example
907cac3a-809a-4bbe-a93e-ae7e944c5cae_kurento.MediaPipeline/403da25a-805b-4cf1-8c55-f190588e6c9b_kurento.WebRtcEndpoint
*
*
*
* - name: free text intended to provide a friendly name for this
MediaObject
. Its default value is the same as the ID.
* - tags: key-value pairs intended for applications to associate metadata to this
MediaObject
instance.
*
*
*
Events
*
* - `ErrorEvent`: reports asynchronous error events. It is recommended to always subscribe a listener to this event, as regular error from the pipeline will be notified through it, instead of through an exception when invoking a method.
*
*
*
**/
@org.kurento.client.internal.RemoteClass
public interface MediaObject extends KurentoObject {
/**
*
* Get {@link module:core.MediaPipeline MediaPipeline} to which this MediaObject
belongs. It returns itself when invoked for a pipeline object.
*
**/
org.kurento.client.MediaPipeline getMediaPipeline();
/**
*
* Get {@link module:core.MediaPipeline MediaPipeline} to which this MediaObject
belongs. It returns itself when invoked for a pipeline object.
*
**/
void getMediaPipeline(Continuation cont);
/**
*
* Get {@link module:core.MediaPipeline MediaPipeline} to which this MediaObject
belongs. It returns itself when invoked for a pipeline object.
*
**/
TFuture getMediaPipeline(Transaction tx);
/**
*
* Get parent of this MediaObject
. The parent of a {@link module:core/abstracts.Hub Hub} or a {@link module:core/abstracts.MediaElement MediaElement} is its {@link module:core.MediaPipeline MediaPipeline}. A {@link module:core.MediaPipeline MediaPipeline} has no parent, so this property will be null.
*
**/
org.kurento.client.MediaObject getParent();
/**
*
* Get parent of this MediaObject
. The parent of a {@link module:core/abstracts.Hub Hub} or a {@link module:core/abstracts.MediaElement MediaElement} is its {@link module:core.MediaPipeline MediaPipeline}. A {@link module:core.MediaPipeline MediaPipeline} has no parent, so this property will be null.
*
**/
void getParent(Continuation cont);
/**
*
* Get parent of this MediaObject
. The parent of a {@link module:core/abstracts.Hub Hub} or a {@link module:core/abstracts.MediaElement MediaElement} is its {@link module:core.MediaPipeline MediaPipeline}. A {@link module:core.MediaPipeline MediaPipeline} has no parent, so this property will be null.
*
**/
TFuture getParent(Transaction tx);
/**
*
* Get unique identifier of this MediaObject
. It's a synthetic identifier composed by a GUID and MediaObject
type. The ID is prefixed with the parent ID when the object has parent: ID_parent/ID_media-object.
*
**/
String getId();
/**
*
* Get unique identifier of this MediaObject
. It's a synthetic identifier composed by a GUID and MediaObject
type. The ID is prefixed with the parent ID when the object has parent: ID_parent/ID_media-object.
*
**/
void getId(Continuation cont);
/**
*
* Get unique identifier of this MediaObject
. It's a synthetic identifier composed by a GUID and MediaObject
type. The ID is prefixed with the parent ID when the object has parent: ID_parent/ID_media-object.
*
**/
TFuture getId(Transaction tx);
/**
*
* @deprecated
* Get (Use children instead) children of this MediaObject
.
*
**/
java.util.List getChilds();
/**
*
* @deprecated
* Get (Use children instead) children of this MediaObject
.
*
**/
void getChilds(Continuation> cont);
/**
*
* @deprecated
* Get (Use children instead) children of this MediaObject
.
*
**/
TFuture> getChilds(Transaction tx);
/**
*
* Get children of this MediaObject
.
*
**/
java.util.List getChildren();
/**
*
* Get children of this MediaObject
.
*
**/
void getChildren(Continuation> cont);
/**
*
* Get children of this MediaObject
.
*
**/
TFuture> getChildren(Transaction tx);
/**
*
* Get this MediaObject
's name. This is just a comodity to simplify developers' life debugging, it is not used internally for indexing nor idenfiying the objects. By default, it's the object's ID.
*
**/
String getName();
/**
*
* Get this MediaObject
's name. This is just a comodity to simplify developers' life debugging, it is not used internally for indexing nor idenfiying the objects. By default, it's the object's ID.
*
**/
void getName(Continuation cont);
/**
*
* Get this MediaObject
's name. This is just a comodity to simplify developers' life debugging, it is not used internally for indexing nor idenfiying the objects. By default, it's the object's ID.
*
**/
TFuture getName(Transaction tx);
/**
*
* Set this MediaObject
's name. This is just a comodity to simplify developers' life debugging, it is not used internally for indexing nor idenfiying the objects. By default, it's the object's ID.
*
**/
void setName(@org.kurento.client.internal.server.Param("name") String name);
/**
*
* Set this MediaObject
's name. This is just a comodity to simplify developers' life debugging, it is not used internally for indexing nor idenfiying the objects. By default, it's the object's ID.
*
**/
void setName(@org.kurento.client.internal.server.Param("name") String name, Continuation cont);
/**
*
* Set this MediaObject
's name. This is just a comodity to simplify developers' life debugging, it is not used internally for indexing nor idenfiying the objects. By default, it's the object's ID.
*
**/
void setName(@org.kurento.client.internal.server.Param("name") String name, Transaction tx);
/**
*
* Get flag activating or deactivating sending the element's tags in fired events.
*
**/
boolean getSendTagsInEvents();
/**
*
* Get flag activating or deactivating sending the element's tags in fired events.
*
**/
void getSendTagsInEvents(Continuation cont);
/**
*
* Get flag activating or deactivating sending the element's tags in fired events.
*
**/
TFuture getSendTagsInEvents(Transaction tx);
/**
*
* Set flag activating or deactivating sending the element's tags in fired events.
*
**/
void setSendTagsInEvents(@org.kurento.client.internal.server.Param("sendTagsInEvents") boolean sendTagsInEvents);
/**
*
* Set flag activating or deactivating sending the element's tags in fired events.
*
**/
void setSendTagsInEvents(@org.kurento.client.internal.server.Param("sendTagsInEvents") boolean sendTagsInEvents, Continuation cont);
/**
*
* Set flag activating or deactivating sending the element's tags in fired events.
*
**/
void setSendTagsInEvents(@org.kurento.client.internal.server.Param("sendTagsInEvents") boolean sendTagsInEvents, Transaction tx);
/**
*
* Get MediaObject
creation time in seconds since Epoch.
*
**/
int getCreationTime();
/**
*
* Get MediaObject
creation time in seconds since Epoch.
*
**/
void getCreationTime(Continuation cont);
/**
*
* Get MediaObject
creation time in seconds since Epoch.
*
**/
TFuture getCreationTime(Transaction tx);
/**
*
* Adds a new tag to this MediaObject
. If the tag is already present, it changes the value.
*
* @param key
* Tag name.
* @param value
* Value associated to this tag.
*
**/
void addTag(@org.kurento.client.internal.server.Param("key") String key, @org.kurento.client.internal.server.Param("value") String value);
/**
*
* Asynchronous version of addTag:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see MediaObject#addTag
*
* @param key
* Tag name.
* @param value
* Value associated to this tag.
*
**/
void addTag(@org.kurento.client.internal.server.Param("key") String key, @org.kurento.client.internal.server.Param("value") String value, Continuation cont);
/**
*
* Adds a new tag to this MediaObject
. If the tag is already present, it changes the value.
*
* @param key
* Tag name.
* @param value
* Value associated to this tag.
*
**/
void addTag(Transaction tx, @org.kurento.client.internal.server.Param("key") String key, @org.kurento.client.internal.server.Param("value") String value);
/**
*
* Removes an existing tag. Exists silently with no error if tag is not defined.
*
* @param key
* Tag name to be removed
*
**/
void removeTag(@org.kurento.client.internal.server.Param("key") String key);
/**
*
* Asynchronous version of removeTag:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see MediaObject#removeTag
*
* @param key
* Tag name to be removed
*
**/
void removeTag(@org.kurento.client.internal.server.Param("key") String key, Continuation cont);
/**
*
* Removes an existing tag. Exists silently with no error if tag is not defined.
*
* @param key
* Tag name to be removed
*
**/
void removeTag(Transaction tx, @org.kurento.client.internal.server.Param("key") String key);
/**
*
* Returns the value of given tag, or MEDIA_OBJECT_TAG_KEY_NOT_FOUND if tag is not defined.
*
* @param key
* Tag key.
* @return The value associated to the given key. *
**/
String getTag(@org.kurento.client.internal.server.Param("key") String key);
/**
*
* Asynchronous version of getTag:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see MediaObject#getTag
*
* @param key
* Tag key.
*
**/
void getTag(@org.kurento.client.internal.server.Param("key") String key, Continuation cont);
/**
*
* Returns the value of given tag, or MEDIA_OBJECT_TAG_KEY_NOT_FOUND if tag is not defined.
*
* @param key
* Tag key.
* @return The value associated to the given key. *
**/
TFuture getTag(Transaction tx, @org.kurento.client.internal.server.Param("key") String key);
/**
*
* Returns all tags attached to this MediaObject
.
* @return An array containing all key-value pairs associated with this MediaObject
. *
**/
java.util.List getTags();
/**
*
* Asynchronous version of getTags:
* {@link Continuation#onSuccess} is called when the action is
* done. If an error occurs, {@link Continuation#onError} is called.
* @see MediaObject#getTags
*
**/
void getTags(Continuation> cont);
/**
*
* Returns all tags attached to this MediaObject
.
* @return An array containing all key-value pairs associated with this MediaObject
. *
**/
TFuture> getTags(Transaction tx);
/**
* Add a {@link EventListener} for event {@link ErrorEvent}. Synchronous call.
*
* @param listener Listener to be called on ErrorEvent
* @return ListenerSubscription for the given Listener
*
**/
@org.kurento.client.internal.server.EventSubscription(ErrorEvent.class)
ListenerSubscription addErrorListener(EventListener listener);
/**
* Add a {@link EventListener} for event {@link ErrorEvent}. Asynchronous call.
* Calls Continuation<ListenerSubscription> when it has been added.
*
* @param listener Listener to be called on ErrorEvent
* @param cont Continuation to be called when the listener is registered
*
**/
@org.kurento.client.internal.server.EventSubscription(ErrorEvent.class)
void addErrorListener(EventListener listener, Continuation cont);
/**
* Remove a {@link ListenerSubscription} for event {@link ErrorEvent}. Synchronous call.
*
* @param listenerSubscription Listener subscription to be removed
*
**/
@org.kurento.client.internal.server.EventSubscription(ErrorEvent.class)
void removeErrorListener(ListenerSubscription listenerSubscription);
/**
* Remove a {@link ListenerSubscription} for event {@link ErrorEvent}. Asynchronous call.
* Calls Continuation<Void> when it has been removed.
*
* @param listenerSubscription Listener subscription to be removed
* @param cont Continuation to be called when the listener is removed
*
**/
@org.kurento.client.internal.server.EventSubscription(ErrorEvent.class)
void removeErrorListener(ListenerSubscription listenerSubscription, Continuation cont);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy