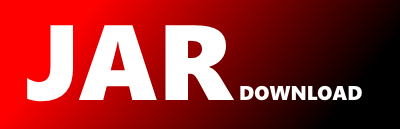
org.ladsn.jdbc.plugin.service.DataSourceService Maven / Gradle / Ivy
The newest version!
package org.ladsn.jdbc.plugin.service;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.HashMap;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import org.apache.commons.lang3.StringUtils;
import org.ladsn.jdbc.config.DynamicDataSourceContextHolder;
import org.ladsn.jdbc.dao.BaseDao;
import org.ladsn.jdbc.plugin.domain.DataSourceInfo;
import org.ladsn.jdbc.support.DataSourcePropertyBinder;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.annotation.Order;
import org.springframework.stereotype.Service;
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.serializer.SerializerFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import lombok.extern.slf4j.Slf4j;
@Slf4j
@Order(4)
@Service
public class DataSourceService {
@Autowired
private BaseDao baseDao;
/**
* 添加数据源
*
* @param dataSourceInfo
*/
public void addDataSource(DataSourceInfo dataSourceInfo) {
Map dataSourceConfig = convertDataSourceConfig(dataSourceInfo);
// 增加数据源
DataSourcePropertyBinder.addDataSource(dataSourceConfig);
log.info("数据源【{}】加载成功.", dataSourceInfo.getName());
}
/**
* 转换实体对象为数据源配置
*
* @param dataSourceInfo
* @return
*/
public Map convertDataSourceConfig(DataSourceInfo dataSourceInfo) {
Map map = null;
ObjectMapper mapper = new ObjectMapper();
try {
// 转换实体为数据源配置对象
String json = mapper.writeValueAsString(dataSourceInfo);
map = JSON.parseObject(json);
// 获取连接池其他配置
Map poolProperties = getPoolProperties(dataSourceInfo.getPoolProperties());
map.putAll(poolProperties);
log.debug(JSON.toJSONString(map, SerializerFeature.WriteNullStringAsEmpty));
} catch (Exception e) {
e.printStackTrace();
}
return map;
}
/**
* 获取字段中的属性
*
* @param config
* @return
*/
public Map getPoolProperties(String config) {
Map map = new HashMap();
if (StringUtils.isNotBlank(config)) {
Properties properties = new Properties();
try {
InputStream is = new ByteArrayInputStream(config.getBytes());
properties.load(is);
Set> entrySet = properties.entrySet();
for (Map.Entry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy