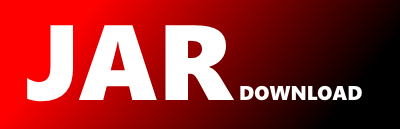
jp.go.nict.langrid.client.protobuf.proto.CommonProtos Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jp.go.nict.langrid.commons.protobufrpc Show documentation
Show all versions of jp.go.nict.langrid.commons.protobufrpc Show documentation
Common and utility library about ProtocolBuffers RPC for the Service Grid Server Software and java web services.
// Generated by the protocol buffer compiler. DO NOT EDIT!
package jp.go.nict.langrid.client.protobuf.proto;
public final class CommonProtos {
private CommonProtos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public static final class Header extends
com.google.protobuf.GeneratedMessage {
// Use Header.newBuilder() to construct.
private Header() {}
private static final Header defaultInstance = new Header();
public static Header getDefaultInstance() {
return defaultInstance;
}
public Header getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return jp.go.nict.langrid.client.protobuf.proto.CommonProtos.internal_static_Header_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return jp.go.nict.langrid.client.protobuf.proto.CommonProtos.internal_static_Header_fieldAccessorTable;
}
// required string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private boolean hasName;
private java.lang.String name_ = "";
public boolean hasName() { return hasName; }
public java.lang.String getName() { return name_; }
// required string value = 2;
public static final int VALUE_FIELD_NUMBER = 2;
private boolean hasValue;
private java.lang.String value_ = "";
public boolean hasValue() { return hasValue; }
public java.lang.String getValue() { return value_; }
public final boolean isInitialized() {
if (!hasName) return false;
if (!hasValue) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (hasName()) {
output.writeString(1, getName());
}
if (hasValue()) {
output.writeString(2, getValue());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasName()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getName());
}
if (hasValue()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getValue());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeDelimitedFrom(input).buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeDelimitedFrom(input, extensionRegistry)
.buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header result;
// Construct using jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header();
return builder;
}
protected jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header.getDescriptor();
}
public jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header getDefaultInstanceForType() {
return jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header) {
return mergeFrom((jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header other) {
if (other == jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header.getDefaultInstance()) return this;
if (other.hasName()) {
setName(other.getName());
}
if (other.hasValue()) {
setValue(other.getValue());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setName(input.readString());
break;
}
case 18: {
setValue(input.readString());
break;
}
}
}
}
// required string name = 1;
public boolean hasName() {
return result.hasName();
}
public java.lang.String getName() {
return result.getName();
}
public Builder setName(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasName = true;
result.name_ = value;
return this;
}
public Builder clearName() {
result.hasName = false;
result.name_ = getDefaultInstance().getName();
return this;
}
// required string value = 2;
public boolean hasValue() {
return result.hasValue();
}
public java.lang.String getValue() {
return result.getValue();
}
public Builder setValue(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasValue = true;
result.value_ = value;
return this;
}
public Builder clearValue() {
result.hasValue = false;
result.value_ = getDefaultInstance().getValue();
return this;
}
}
static {
jp.go.nict.langrid.client.protobuf.proto.CommonProtos.getDescriptor();
}
static {
jp.go.nict.langrid.client.protobuf.proto.CommonProtos.internalForceInit();
}
}
public static final class Fault extends
com.google.protobuf.GeneratedMessage {
// Use Fault.newBuilder() to construct.
private Fault() {}
private static final Fault defaultInstance = new Fault();
public static Fault getDefaultInstance() {
return defaultInstance;
}
public Fault getDefaultInstanceForType() {
return defaultInstance;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return jp.go.nict.langrid.client.protobuf.proto.CommonProtos.internal_static_Fault_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return jp.go.nict.langrid.client.protobuf.proto.CommonProtos.internal_static_Fault_fieldAccessorTable;
}
// required string faultCode = 1;
public static final int FAULTCODE_FIELD_NUMBER = 1;
private boolean hasFaultCode;
private java.lang.String faultCode_ = "";
public boolean hasFaultCode() { return hasFaultCode; }
public java.lang.String getFaultCode() { return faultCode_; }
// required string faultString = 2;
public static final int FAULTSTRING_FIELD_NUMBER = 2;
private boolean hasFaultString;
private java.lang.String faultString_ = "";
public boolean hasFaultString() { return hasFaultString; }
public java.lang.String getFaultString() { return faultString_; }
// required string faultDetail = 3;
public static final int FAULTDETAIL_FIELD_NUMBER = 3;
private boolean hasFaultDetail;
private java.lang.String faultDetail_ = "";
public boolean hasFaultDetail() { return hasFaultDetail; }
public java.lang.String getFaultDetail() { return faultDetail_; }
public final boolean isInitialized() {
if (!hasFaultCode) return false;
if (!hasFaultString) return false;
if (!hasFaultDetail) return false;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (hasFaultCode()) {
output.writeString(1, getFaultCode());
}
if (hasFaultString()) {
output.writeString(2, getFaultString());
}
if (hasFaultDetail()) {
output.writeString(3, getFaultDetail());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (hasFaultCode()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(1, getFaultCode());
}
if (hasFaultString()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(2, getFaultString());
}
if (hasFaultDetail()) {
size += com.google.protobuf.CodedOutputStream
.computeStringSize(3, getFaultDetail());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data).buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return newBuilder().mergeFrom(data, extensionRegistry)
.buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault parseFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return newBuilder().mergeDelimitedFrom(input).buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeDelimitedFrom(input, extensionRegistry)
.buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return newBuilder().mergeFrom(input).buildParsed();
}
public static jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return newBuilder().mergeFrom(input, extensionRegistry)
.buildParsed();
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder {
private jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault result;
// Construct using jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault.newBuilder()
private Builder() {}
private static Builder create() {
Builder builder = new Builder();
builder.result = new jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault();
return builder;
}
protected jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault internalGetResult() {
return result;
}
public Builder clear() {
if (result == null) {
throw new IllegalStateException(
"Cannot call clear() after build().");
}
result = new jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault();
return this;
}
public Builder clone() {
return create().mergeFrom(result);
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault.getDescriptor();
}
public jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault getDefaultInstanceForType() {
return jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault.getDefaultInstance();
}
public boolean isInitialized() {
return result.isInitialized();
}
public jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault build() {
if (result != null && !isInitialized()) {
throw newUninitializedMessageException(result);
}
return buildPartial();
}
private jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault buildParsed()
throws com.google.protobuf.InvalidProtocolBufferException {
if (!isInitialized()) {
throw newUninitializedMessageException(
result).asInvalidProtocolBufferException();
}
return buildPartial();
}
public jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault buildPartial() {
if (result == null) {
throw new IllegalStateException(
"build() has already been called on this Builder.");
}
jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault returnMe = result;
result = null;
return returnMe;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault) {
return mergeFrom((jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault other) {
if (other == jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault.getDefaultInstance()) return this;
if (other.hasFaultCode()) {
setFaultCode(other.getFaultCode());
}
if (other.hasFaultString()) {
setFaultString(other.getFaultString());
}
if (other.hasFaultDetail()) {
setFaultDetail(other.getFaultDetail());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder(
this.getUnknownFields());
while (true) {
int tag = input.readTag();
switch (tag) {
case 0:
this.setUnknownFields(unknownFields.build());
return this;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
this.setUnknownFields(unknownFields.build());
return this;
}
break;
}
case 10: {
setFaultCode(input.readString());
break;
}
case 18: {
setFaultString(input.readString());
break;
}
case 26: {
setFaultDetail(input.readString());
break;
}
}
}
}
// required string faultCode = 1;
public boolean hasFaultCode() {
return result.hasFaultCode();
}
public java.lang.String getFaultCode() {
return result.getFaultCode();
}
public Builder setFaultCode(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasFaultCode = true;
result.faultCode_ = value;
return this;
}
public Builder clearFaultCode() {
result.hasFaultCode = false;
result.faultCode_ = getDefaultInstance().getFaultCode();
return this;
}
// required string faultString = 2;
public boolean hasFaultString() {
return result.hasFaultString();
}
public java.lang.String getFaultString() {
return result.getFaultString();
}
public Builder setFaultString(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasFaultString = true;
result.faultString_ = value;
return this;
}
public Builder clearFaultString() {
result.hasFaultString = false;
result.faultString_ = getDefaultInstance().getFaultString();
return this;
}
// required string faultDetail = 3;
public boolean hasFaultDetail() {
return result.hasFaultDetail();
}
public java.lang.String getFaultDetail() {
return result.getFaultDetail();
}
public Builder setFaultDetail(java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
result.hasFaultDetail = true;
result.faultDetail_ = value;
return this;
}
public Builder clearFaultDetail() {
result.hasFaultDetail = false;
result.faultDetail_ = getDefaultInstance().getFaultDetail();
return this;
}
}
static {
jp.go.nict.langrid.client.protobuf.proto.CommonProtos.getDescriptor();
}
static {
jp.go.nict.langrid.client.protobuf.proto.CommonProtos.internalForceInit();
}
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Header_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Header_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_Fault_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_Fault_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\014common.proto\"%\n\006Header\022\014\n\004name\030\001 \002(\t\022\r" +
"\n\005value\030\002 \002(\t\"D\n\005Fault\022\021\n\tfaultCode\030\001 \002(" +
"\t\022\023\n\013faultString\030\002 \002(\t\022\023\n\013faultDetail\030\003 " +
"\002(\tB8\n(jp.go.nict.langrid.client.protobu" +
"f.protoB\014CommonProtos"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_Header_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_Header_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Header_descriptor,
new java.lang.String[] { "Name", "Value", },
jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header.class,
jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Header.Builder.class);
internal_static_Fault_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_Fault_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_Fault_descriptor,
new java.lang.String[] { "FaultCode", "FaultString", "FaultDetail", },
jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault.class,
jp.go.nict.langrid.client.protobuf.proto.CommonProtos.Fault.Builder.class);
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
public static void internalForceInit() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy