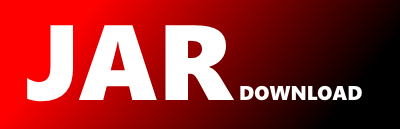
ucar.ma2.ArrayBoolean Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 1998-2018 University Corporation for Atmospheric Research/Unidata
* See LICENSE for license information.
*/
package ucar.ma2;
/**
* Concrete implementation of Array specialized for booleans.
* Data storage is with 1D java array of booleans.
*
* issues: what should we do if a conversion loses accuracy? nothing ? Exception ?
*
* @see Array
* @author caron
*/
public class ArrayBoolean extends Array {
// package private. use Array.factory() */
static ArrayBoolean factory(Index index) {
return ArrayBoolean.factory(index, null);
}
/* create new ArrayBoolean with given indexImpl and backing store.
* Should be private.
* @param index use this Index
* @param stor. use this storage. if null, allocate.
* @return. new ArrayDouble.D or ArrayDouble object.
*/
static ArrayBoolean factory( Index index, boolean [] storage) {
if (index instanceof Index0D) {
return new ArrayBoolean.D0(index, storage);
} else if (index instanceof Index1D) {
return new ArrayBoolean.D1(index, storage);
} else if (index instanceof Index2D) {
return new ArrayBoolean.D2(index, storage);
} else if (index instanceof Index3D) {
return new ArrayBoolean.D3(index, storage);
} else if (index instanceof Index4D) {
return new ArrayBoolean.D4(index, storage);
} else if (index instanceof Index5D) {
return new ArrayBoolean.D5(index, storage);
} else if (index instanceof Index6D) {
return new ArrayBoolean.D6(index, storage);
} else if (index instanceof Index7D) {
return new ArrayBoolean.D7(index, storage);
} else {
return new ArrayBoolean(index, storage);
}
}
///////////////////////////////////////////////////////////////////////////////
protected boolean[] storage;
/**
* Create a new Array of type boolean and the given shape.
* dimensions.length determines the rank of the new Array.
* @param dimensions the shape of the Array.
*/
public ArrayBoolean(int [] dimensions) {
super(DataType.BOOLEAN, dimensions);
storage = new boolean[(int)indexCalc.getSize()];
}
/**
* Create a new Array using the given IndexArray and backing store.
* used for sections. Trusted package private.
* @param ima use this IndexArray as the index
* @param data use this as the backing store
*/
ArrayBoolean(Index ima, boolean [] data) {
super(DataType.BOOLEAN, ima);
/* replace by something better
if (ima.getSize() != data.length)
throw new IllegalArgumentException("bad data length"); */
if (data != null)
storage = data;
else
storage = new boolean[(int)ima.getSize()];
}
/** create new Array with given indexImpl and same backing store */
protected Array createView( Index index) {
return ArrayBoolean.factory( index, storage);
}
/* Get underlying primitive array storage. CAUTION! You may invalidate your warrentee! */
public Object getStorage() { return storage; }
// copy from javaArray to storage using the iterator: used by factory( Object);
protected void copyFrom1DJavaArray(IndexIterator iter, Object javaArray) {
boolean[] ja = (boolean []) javaArray;
for (boolean aJa : ja) iter.setBooleanNext(aJa);
}
// copy to javaArray from storage using the iterator: used by copyToNDJavaArray;
protected void copyTo1DJavaArray(IndexIterator iter, Object javaArray) {
boolean[] ja = (boolean []) javaArray;
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy