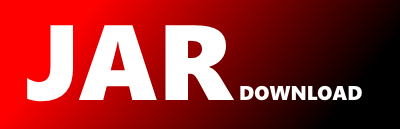
ucar.ma2.ArrayObject Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 1998-2018 University Corporation for Atmospheric Research/Unidata
* See LICENSE for license information.
*/
package ucar.ma2;
/**
* Concrete implementation of Array specialized for Objects.
* Data storage is with 1D java array of Objects.
*
* @see Array
* @author caron
*/
public class ArrayObject extends Array {
/** package private. use Array.factory() */
static ArrayObject factory(DataType dtype, Class elemType, boolean isVlen, Index index) {
return ArrayObject.factory(dtype, elemType, isVlen, index, null);
}
/* Create new ArrayObject with given indexImpl and backing store.
* Should be private.
* @param index use this Index
* @param stor. use this storage. if null, allocate.
* @return. new ArrayObject.D or ArrayObject object.
*/
static ArrayObject factory(DataType dtype, Class elemType, boolean isVlen, Index index, Object[] storage) {
if (index instanceof Index0D) {
return new ArrayObject.D0(dtype, elemType, isVlen, index, storage);
} else if (index instanceof Index1D) {
return new ArrayObject.D1(dtype, elemType, isVlen, index, storage);
} else if (index instanceof Index2D) {
return new ArrayObject.D2(dtype, elemType, isVlen, index, storage);
} else if (index instanceof Index3D) {
return new ArrayObject.D3(dtype, elemType, isVlen, index, storage);
} else if (index instanceof Index4D) {
return new ArrayObject.D4(dtype, elemType, isVlen, index, storage);
} else if (index instanceof Index5D) {
return new ArrayObject.D5(dtype, elemType, isVlen, index, storage);
} else if (index instanceof Index6D) {
return new ArrayObject.D6(dtype, elemType, isVlen, index, storage);
} else if (index instanceof Index7D) {
return new ArrayObject.D7(dtype, elemType, isVlen, index, storage);
} else {
return new ArrayObject(dtype, elemType, isVlen, index, storage);
}
}
///////////////////////////////////////////////////////////////////////////////
protected final boolean isVlen;
protected final Class elementType;
protected final Object[] storage;
/**
* Create a new Array of type Object and the given shape.
* dimensions.length determines the rank of the new Array.
* @param elementType the type of element, eg String
* @param shape the shape of the Array.
*/
public ArrayObject(DataType dtype, Class elementType, boolean isVlen, int[] shape) {
super(dtype, shape);
this.elementType = elementType;
storage = new Object[(int) indexCalc.getSize()];
this.isVlen = isVlen;
}
/**
* Create a new Array of type Object and the given shape and storage.
* dimensions.length determines the rank of the new Array.
* @param elementType the type of element, eg String
* @param shape the shape of the Array.
*/
public ArrayObject(DataType dtype, Class elementType, boolean isVlen, int [] shape, Object[] storage) {
super(dtype, shape);
this.elementType = elementType;
this.storage = storage;
this.isVlen = isVlen;
}
/**
* Create a new Array using the given IndexArray and backing store.
* used for sections, and factory. Trusted package private.
* @param elementType the type of element, eg String
* @param ima use this IndexArray as the index
* @param data use this as the backing store. if null, allocate
*/
ArrayObject(DataType dtype, Class elementType, boolean isVlen, Index ima, Object[] data) {
super(dtype, ima);
this.elementType = elementType;
this.isVlen = isVlen;
if (data != null) {
storage = data;
} else {
storage = new Object[(int) indexCalc.getSize()];
}
}
/** create new Array with given indexImpl and the same backing store */
protected Array createView( Index index) {
return ArrayObject.factory( dataType, elementType, isVlen, index, storage);
}
@Override
public Array copy() {
Array newA = factory(getDataType(), getElementType(), isVlen(), Index.factory(getShape()));
MAMath.copy(newA, this);
return newA;
}
/** Get underlying primitive array storage. CAUTION! You may invalidate your warrentee! */
public Object getStorage() { return storage; }
// copy from javaArray to storage using the iterator: used by factory( Object);
protected void copyFrom1DJavaArray(IndexIterator iter, Object javaArray) {
Object[] ja = (Object []) javaArray;
for (Object aJa : ja) iter.setObjectNext(aJa);
}
// copy to javaArray from storage using the iterator: used by copyToNDJavaArray;
protected void copyTo1DJavaArray(IndexIterator iter, Object javaArray) {
Object[] ja = (Object []) javaArray;
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy