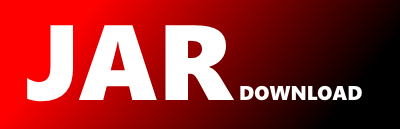
ucar.ma2.ArraySequenceNested Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 1998-2018 University Corporation for Atmospheric Research/Unidata
* See LICENSE for license information.
*/
package ucar.ma2;
/**
* Handles nested sequences: a 1D array of variable length 1D arrays of StructureData.
* Uses same technique as ArrayStructureMA for the inner fields; data storage is in member arrays.
* Used only by opendap internals.
*
* Example use:
*
ArraySequence aseq = new ArraySequence( members, outerLength);
for (int seq=0; seq < outerLength; seq++) {
aseq.setSequenceLength(seq, seqLength);
}
aseq.finish();
*
* @author caron
*/
public class ArraySequenceNested extends ArrayStructure {
private int[] sequenceLen;
private int[] sequenceOffset;
private int total = 0;
/**
* This is used for inner sequences, ie variable length structures nested inside of another structure.
* @param members the members of the STructure
* @param nseq the number of sequences, ie the length of the outer structure.
*/
public ArraySequenceNested(StructureMembers members, int nseq) {
super(members, new int[] {nseq});
sequenceLen = new int[nseq];
}
// not sure how this is used
protected StructureData makeStructureData( ArrayStructure as, int index) {
return new StructureDataA( as, index);
}
public StructureData getStructureData(int index) {
return new StructureDataA( this, index);
}
/**
* Set the length of one of the sequences.
* @param outerIndex which sequence?
* @param len what is its length?
*/
public void setSequenceLength( int outerIndex, int len) {
sequenceLen[outerIndex] = len;
}
/**
* Get the length of the ith sequence.
* @param outerIndex which sequence?
* @return its length
*/
public int getSequenceLength( int outerIndex) {
return sequenceLen[outerIndex];
}
/**
* Get the the starting index of the ith sequence.
* @param outerIndex which sequence?
* @return its starting index
*/
public int getSequenceOffset( int outerIndex) {
return sequenceOffset[outerIndex];
}
/**
* Call this when you have set all the sequence lengths.
*/
public void finish() {
sequenceOffset = new int[nelems];
total = 0;
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy