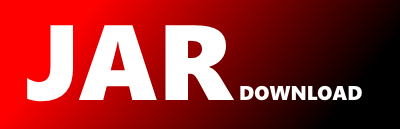
lt.lang.implicit.collection.RichIterable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of latte-compiler Show documentation
Show all versions of latte-compiler Show documentation
The latte-lang compiler project, which contains compiler and runtime required library.
The newest version!
package lt.lang.implicit.collection;
import lt.lang.function.Function1;
import java.util.LinkedList;
import java.util.List;
/**
* rich iterator
*/
public class RichIterable {
private final Iterable iterable;
public RichIterable(Iterable iterable) {
this.iterable = iterable;
}
public void forEach(Function1 f) throws Exception {
for (T t : iterable) {
f.apply(t);
}
}
public List filter(Function1 f) throws Exception {
List list = new LinkedList();
for (T t : iterable) {
if (f.apply(t)) {
list.add(t);
}
}
return list;
}
public List map(Function1 extends U, ? super T> f) throws Exception {
List list = new LinkedList();
for (T t : iterable) {
list.add(f.apply(t));
}
return list;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy