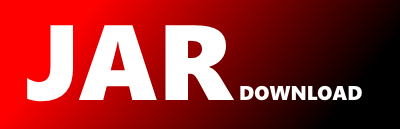
org.ldaptive.pool.PoolConfig Maven / Gradle / Ivy
/*
$Id: PoolConfig.java 3161 2016-10-05 20:40:19Z daniel_fisher $
Copyright (C) 2003-2014 Virginia Tech.
All rights reserved.
SEE LICENSE FOR MORE INFORMATION
Author: Middleware Services
Email: [email protected]
Version: $Revision: 3161 $
Updated: $Date: 2016-10-05 16:40:19 -0400 (Wed, 05 Oct 2016) $
*/
package org.ldaptive.pool;
import java.util.concurrent.TimeUnit;
import org.ldaptive.AbstractConfig;
import org.ldaptive.LdapUtils;
/**
* Contains all the configuration data that the pooling implementations need to
* control the pool.
*
* @author Middleware Services
* @version $Revision: 3161 $ $Date: 2016-10-05 16:40:19 -0400 (Wed, 05 Oct 2016) $
*/
public class PoolConfig extends AbstractConfig
{
/** Default min pool size, value is {@value}. */
public static final int DEFAULT_MIN_POOL_SIZE = 3;
/** Default max pool size, value is {@value}. */
public static final int DEFAULT_MAX_POOL_SIZE = 10;
/** Default validate on check in, value is {@value}. */
public static final boolean DEFAULT_VALIDATE_ON_CHECKIN = false;
/** Default validate on check out, value is {@value}. */
public static final boolean DEFAULT_VALIDATE_ON_CHECKOUT = false;
/** Default validate periodically, value is {@value}. */
public static final boolean DEFAULT_VALIDATE_PERIODICALLY = false;
/** Default validate period, value is {@value}. */
public static final long DEFAULT_VALIDATE_PERIOD = 1800;
/** Default per connection validate timeout, value is {@value}. */
public static final long DEFAULT_VALIDATE_TIMEOUT = 5000;
/** Minimum pool size. */
private int minPoolSize = DEFAULT_MIN_POOL_SIZE;
/** Maximum pool size. */
private int maxPoolSize = DEFAULT_MAX_POOL_SIZE;
/** Whether the ldap object should be validated when returned to the pool. */
private boolean validateOnCheckIn = DEFAULT_VALIDATE_ON_CHECKIN;
/** Whether the ldap object should be validated when given from the pool. */
private boolean validateOnCheckOut = DEFAULT_VALIDATE_ON_CHECKOUT;
/** Whether the pool should be validated periodically. */
private boolean validatePeriodically = DEFAULT_VALIDATE_PERIODICALLY;
/** Time in seconds that the validate pool should repeat. */
private long validatePeriod = DEFAULT_VALIDATE_PERIOD;
/** Maximum time in milliseconds a connection validation should block. */
private long validateTimeout = DEFAULT_VALIDATE_TIMEOUT;
/**
* Returns the min pool size. Default value is {@link #DEFAULT_MIN_POOL_SIZE}.
* This value represents the size of the pool after a prune has occurred.
*
* @return min pool size
*/
public int getMinPoolSize()
{
return minPoolSize;
}
/**
* Sets the min pool size.
*
* @param size min pool size
*/
public void setMinPoolSize(final int size)
{
checkImmutable();
if (size >= 0) {
logger.trace("setting minPoolSize: {}", size);
minPoolSize = size;
}
}
/**
* Returns the max pool size. Default value is {@link #DEFAULT_MAX_POOL_SIZE}.
* This value may or may not be strictly enforced depending on the pooling
* implementation.
*
* @return max pool size
*/
public int getMaxPoolSize()
{
return maxPoolSize;
}
/**
* Sets the max pool size.
*
* @param size max pool size
*/
public void setMaxPoolSize(final int size)
{
checkImmutable();
if (size >= 0) {
logger.trace("setting maxPoolSize: {}", size);
maxPoolSize = size;
}
}
/**
* Returns the validate on check in flag. Default value is {@link
* #DEFAULT_VALIDATE_ON_CHECKIN}.
*
* @return validate on check in
*/
public boolean isValidateOnCheckIn()
{
return validateOnCheckIn;
}
/**
* Sets the validate on check in flag.
*
* @param b validate on check in
*/
public void setValidateOnCheckIn(final boolean b)
{
checkImmutable();
logger.trace("setting validateOnCheckIn: {}", b);
validateOnCheckIn = b;
}
/**
* Returns the validate on check out flag. Default value is {@link
* #DEFAULT_VALIDATE_ON_CHECKOUT}.
*
* @return validate on check in
*/
public boolean isValidateOnCheckOut()
{
return validateOnCheckOut;
}
/**
* Sets the validate on check out flag.
*
* @param b validate on check out
*/
public void setValidateOnCheckOut(final boolean b)
{
checkImmutable();
logger.trace("setting validateOnCheckOut: {}", b);
validateOnCheckOut = b;
}
/**
* Returns the validate periodically flag. Default value is {@link
* #DEFAULT_VALIDATE_PERIODICALLY}.
*
* @return validate periodically
*/
public boolean isValidatePeriodically()
{
return validatePeriodically;
}
/**
* Sets the validate periodically flag.
*
* @param b validate periodically
*/
public void setValidatePeriodically(final boolean b)
{
checkImmutable();
logger.trace("setting validatePeriodically: {}", b);
validatePeriodically = b;
}
/**
* Returns the validate period. Default value is {@link
* #DEFAULT_VALIDATE_PERIOD}.
*
* @return validate period in seconds
*/
public long getValidatePeriod()
{
return validatePeriod;
}
/**
* Sets the period for which the pool will be validated.
*
* @param time in seconds
*/
public void setValidatePeriod(final long time)
{
checkImmutable();
if (time >= 0) {
logger.trace("setting validatePeriod: {}", time);
validatePeriod = time;
}
}
/**
* Returns the validate period as a duration.
*
* @return validate period in duration syntax
*/
public String getValidatePeriodDuration()
{
return LdapUtils.durationEncode(validatePeriod, TimeUnit.SECONDS);
}
/**
* Sets the period for which the pool will be validated.
*
* @param time in duration syntax or in seconds
*/
public void setValidatePeriodDuration(final String time)
{
checkImmutable();
if (LdapUtils.isDuration(time)) {
setValidatePeriod(LdapUtils.durationDecode(time, TimeUnit.SECONDS));
} else {
setValidatePeriod(Long.parseLong(time));
}
}
/**
* Returns the timeout in milliseconds imposed when validating a single
* connection.
*
* @return validate timeout
*/
public long getValidateTimeout()
{
return validateTimeout;
}
/**
* Sets the timeout imposed when validating a single connection.
*
* @param time in milliseconds for a connection validation
*/
public void setValidateTimeout(final long time)
{
checkImmutable();
if (time >= -1) {
logger.trace("setting validateTimeout: {}", time);
validateTimeout = time;
}
}
/**
* Returns the validate timeout as a duration.
*
* @return validate timeout in duration syntax
*/
public String getValidateTimeoutDuration()
{
return LdapUtils.durationEncode(validateTimeout, TimeUnit.MILLISECONDS);
}
/**
* Sets the timeout imposed when validating a single connection.
*
* @param time in duration syntax or in milliseconds
*/
public void setValidateTimeoutDuration(final String time)
{
checkImmutable();
if (LdapUtils.isDuration(time)) {
setValidateTimeout(LdapUtils.durationDecode(time, TimeUnit.MILLISECONDS));
} else {
setValidateTimeout(Long.parseLong(time));
}
}
/** {@inheritDoc} */
@Override
public String toString()
{
return
String.format(
"[%s@%d::minPoolSize=%s, maxPoolSize=%s, validateOnCheckIn=%s, " +
"validateOnCheckOut=%s, validatePeriodically=%s, validatePeriod=%s, " +
"validateTimeout=%s]",
getClass().getName(),
hashCode(),
minPoolSize,
maxPoolSize,
validateOnCheckIn,
validateOnCheckOut,
validatePeriodically,
validatePeriod,
validateTimeout);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy