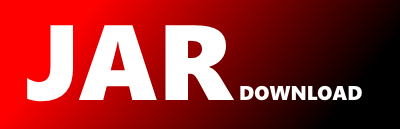
org.lealone.plugins.mysql.server.protocol.RowDataPacket Maven / Gradle / Ivy
/*
* Copyright 1999-2012 Alibaba Group.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.lealone.plugins.mysql.server.protocol;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.List;
import org.lealone.plugins.mysql.server.util.BufferUtil;
/**
* From server to client. One packet for each row in the result set.
*
*
* Bytes Name
* ----- ----
* n (Length Coded String) (column value)
* ...
*
* (column value): The data in the column, as a character string.
* If a column is defined as non-character, the
* server converts the value into a character
* before sending it. Since the value is a Length
* Coded String, a NULL can be represented with a
* single byte containing 251(see the description
* of Length Coded Strings in section "Elements" above).
*
* @see http://forge.mysql.com/wiki/MySQL_Internals_ClientServer_Protocol#Row_Data_Packet
*
*
* @author xianmao.hexm 2010-7-23 上午01:05:55
* @author zhh
*/
public class RowDataPacket extends ResponsePacket {
private static final byte NULL_MARK = (byte) 251;
public final int fieldCount;
public final List fieldValues;
public RowDataPacket(int fieldCount) {
this.fieldCount = fieldCount;
this.fieldValues = new ArrayList<>(fieldCount);
}
public void add(byte[] value) {
fieldValues.add(value);
}
@Override
public String getPacketInfo() {
return "MySQL RowData Packet";
}
@Override
public int calcPacketSize() {
int size = 0;
for (int i = 0; i < fieldCount; i++) {
byte[] v = fieldValues.get(i);
size += (v == null || v.length == 0) ? 1 : BufferUtil.getLength(v);
}
return size;
}
@Override
public void writeBody(ByteBuffer buffer, PacketOutput out) {
for (int i = 0; i < fieldCount; i++) {
byte[] fv = fieldValues.get(i);
if (fv == null || fv.length == 0) {
buffer.put(RowDataPacket.NULL_MARK);
} else {
BufferUtil.writeLength(buffer, fv.length);
buffer = out.writeToBuffer(fv, buffer);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy