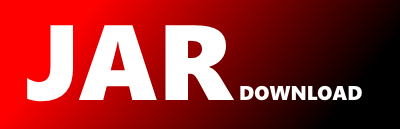
org.leandreck.endpoints.processor.model.EndpointNode Maven / Gradle / Ivy
The newest version!
/**
* Copyright © 2016 Mathias Kowalzik ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.leandreck.endpoints.processor.model;
import java.util.*;
import static java.util.stream.Collectors.toList;
/**
*/
public class EndpointNode {
private final String serviceName;
private final String serviceURL;
private final String template;
private final List methods;
private final List getMethods;
private final List headMethods;
private final List postMethods;
private final List putMethods;
private final List patchMethods;
private final List deleteMethods;
private final List optionsMethods;
private final List traceMethods;
private final Set types;
private final PrintConfiguration printConfiguration;
EndpointNode(final String serviceName, final String serviceURL, final String template, final List methods, final PrintConfiguration printConfiguration) {
this.serviceName = serviceName;
this.serviceURL = serviceURL;
this.template = template;
this.methods = methods;
this.printConfiguration = printConfiguration;
this.getMethods = this.getMethods().stream().filter(m -> m.getHttpMethods().contains("get")).collect(toList());
this.headMethods = this.getMethods().stream().filter(m -> m.getHttpMethods().contains("head")).collect(toList());
this.postMethods = this.getMethods().stream().filter(m -> m.getHttpMethods().contains("post")).collect(toList());
this.putMethods = this.getMethods().stream().filter(m -> m.getHttpMethods().contains("put")).collect(toList());
this.patchMethods = this.getMethods().stream().filter(m -> m.getHttpMethods().contains("patch")).collect(toList());
this.deleteMethods = this.getMethods().stream().filter(m -> m.getHttpMethods().contains("delete")).collect(toList());
this.optionsMethods = this.getMethods().stream().filter(m -> m.getHttpMethods().contains("options")).collect(toList());
this.traceMethods = this.getMethods().stream().filter(m -> m.getHttpMethods().contains("trace")).collect(toList());
this.types = collectTypes();
}
private Set collectTypes() {
final Map typeMap = new HashMap<>();
this.getMethods().stream()
.map(MethodNode::getTypes)
.flatMap(Collection::stream)
.filter(TypeNode::isDeclaredComplexType)
.forEach(type -> typeMap.put(type.getTypeName(), type));
return new HashSet<>(typeMap.values());
}
public String getServiceName() {
return serviceName;
}
public String getServiceURL() {
return serviceURL;
}
public List getMethods() {
return methods;
}
public String getTemplate() {
return template;
}
public Set getTypes() {
return types;
}
public List getGetMethods() {
return getMethods;
}
public List getHeadMethods() {
return headMethods;
}
public List getPostMethods() {
return postMethods;
}
public List getTraceMethods() {
return traceMethods;
}
public List getOptionsMethods() {
return optionsMethods;
}
public List getDeleteMethods() {
return deleteMethods;
}
public List getPatchMethods() {
return patchMethods;
}
public List getPutMethods() {
return putMethods;
}
/**
* Template Engine Configuration of this {@link EndpointNode} for customizing the generated output.
* @return printConfiguration
*/
public PrintConfiguration getPrintConfiguration() {
return printConfiguration;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy