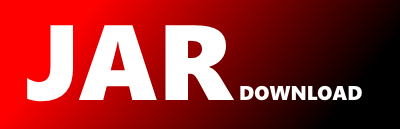
jmms.core.MetaResource Maven / Gradle / Ivy
/*
* Copyright 2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jmms.core;
import leap.lang.Strings;
import leap.lang.net.Urls;
import leap.lang.path.Paths;
import leap.lang.resource.Resource;
/**
* The resource of metadata.
*/
public interface MetaResource {
static String pathByUrl(Resource root, Resource child) {
String rootUrl = root.getURLString();
String childUrl = child.getURLString();
String relativeUri = Strings.removeStart(childUrl, rootUrl);
if (relativeUri.equals(childUrl)) {
throw new IllegalStateException("Invalid child '" + childUrl + "' of root '" + rootUrl + "'");
}
return Paths.prefixWithoutSlash(Paths.normalize(relativeUri));
}
static String pathByClass(String root, Resource child) {
root = Paths.prefixWithoutAndSuffixWithSlash(root);
String cp = Paths.prefixWithoutSlash(child.getClasspath());
String relativeUri = Strings.removeStart(cp, root);
if (relativeUri.equals(cp)) {
throw new IllegalStateException("Invalid child '" + cp + "' of root '" + root + "'");
}
return relativeUri;
}
static String pathByFile(String root, Resource file) {
String childPath = Urls.decode(Paths.normalize(file.getFilepath()));
root = Paths.prefixAndSuffixWithSlash(root);
int index = childPath.indexOf(root);
if (index <= 0) {
throw new IllegalStateException("Invalid root '" + root + "' at '" + file.getURLString() + "'");
}
String relativePath =
Paths.prefixWithAndSuffixWithoutSlash(childPath.substring(index + root.length()));
return relativePath.replace('\\', '/');
}
/**
* Is the resource exists?
*/
default boolean exists() {
return null != getReal() && getReal().exists();
}
/**
* Returns the real resource.
*/
Resource getReal();
/**
* The path relative to the root.
*/
String getPath();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy