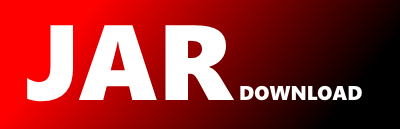
jmms.plugins.RedisApiReaderPlugin Maven / Gradle / Ivy
/*
* Copyright 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jmms.plugins;
import jmms.core.model.MetaApi;
import jmms.engine.Context;
import jmms.engine.Plugin;
import jmms.engine.PluginContext;
import jmms.modules.redis.RedisModule;
import jmms.sdk.Result;
import leap.core.annotation.ConfigProperty;
import leap.core.annotation.Configurable;
import leap.core.validation.annotations.Required;
import leap.lang.Strings;
import leap.lang.json.JSON;
import leap.lang.logging.Log;
import leap.lang.logging.LogFactory;
import redis.clients.jedis.JedisPubSub;
import redis.clients.jedis.exceptions.JedisConnectionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class RedisApiReaderPlugin implements Plugin {
private static final Log log = LogFactory.get(RedisApiReaderPlugin.class);
@Required
@Configurable.Nested
private RedisModule redis = new RedisModule();
private ExecutorService executors;
@Required
@ConfigProperty
private String key = "jmms.api";
@ConfigProperty
private boolean reload = true;
private boolean shutdown;
@Override
public void init(PluginContext context) {
redis.init();
if(reload) {
this.executors = Executors.newCachedThreadPool();
this.subscribe(context);
}
}
@Override
public void destroy(PluginContext context) {
try {
shutdown = true;
redis.destroy();
}finally {
if (null != executors) {
executors.shutdownNow();
executors = null;
}
}
}
@Override
public MetaApi readApi(Context readContext) {
return doReadApi();
}
protected void subscribe(PluginContext pluginContext) {
final RedisPubSub pubSub = new RedisPubSub(pluginContext);
executors.execute(() -> {
redis.directExecOnly((jedis) -> {
String listenedKey="__keyspace@"+jedis.getDB()+"__:" + key;
log.info("Listen redis key pattern: {} ...", listenedKey);
try {
jedis.psubscribe(pubSub, listenedKey);
}catch (JedisConnectionException e) {
log.info(e.getMessage(), e);
}
if(!shutdown) {
//listen again
subscribe(pluginContext);
}
});
});
}
private MetaApi doReadApi() {
Result result = redis.get(key).tryThrowError();
if(Strings.isEmpty(result.getValue())) {
return null;
}
return JSON.decode(result.getValue(), MetaApi.class);
}
protected class RedisPubSub extends JedisPubSub {
private final PluginContext pluginContext;
public RedisPubSub(PluginContext pluginContext) {
this.pluginContext = pluginContext;
}
@Override
public void onPMessage(String pattern, String channel, String message) {
final String command = message;
log.debug("On key command : {} {}", command, key);
switch (command) {
case "set" :
pluginContext.reload();
break;
case "del" :
//remove
break;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy