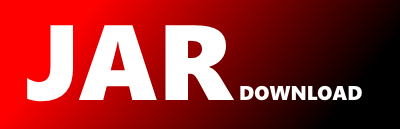
jmms.plugins.sfs.SfsConfig Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2019 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jmms.plugins.sfs;
import leap.lang.Strings;
import leap.lang.logging.Log;
import leap.lang.logging.LogFactory;
import leap.lang.path.Paths;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.boot.context.properties.ConfigurationProperties;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Path;
@ConfigurationProperties("sfs")
public class SfsConfig implements InitializingBean {
private static final Log log = LogFactory.get(SfsConfig.class);
private static final String DEFAULT_SIGN_SECRET = "__sfs_sign_secret_need_to_change__";
protected String dir;
protected int maxWaitingCommits = 10240;
protected int waitingCommitExpires = 30;
protected int signExpires = 30;
protected boolean fileLastAccessEnabled = true;
protected String signSecret = DEFAULT_SIGN_SECRET;
private Path dirPath;
public Path getDirPath() {
return dirPath;
}
public String getDir() {
return dir;
}
public void setDir(String dir) {
this.dir = dir;
}
public int getMaxWaitingCommits() {
return maxWaitingCommits;
}
public void setMaxWaitingCommits(int maxWaitingCommits) {
this.maxWaitingCommits = maxWaitingCommits;
}
public int getWaitingCommitExpires() {
return waitingCommitExpires;
}
public void setWaitingCommitExpires(int waitingCommitExpires) {
this.waitingCommitExpires = waitingCommitExpires;
}
public int getSignExpires() {
return signExpires;
}
public void setSignExpires(int signExpires) {
this.signExpires = signExpires;
}
public boolean isFileLastAccessEnabled() {
return fileLastAccessEnabled;
}
public void setFileLastAccessEnabled(boolean fileLastAccessEnabled) {
this.fileLastAccessEnabled = fileLastAccessEnabled;
}
public String getSignSecret() {
return signSecret;
}
public void setSignSecret(String signSecret) {
this.signSecret = signSecret;
}
@Override
public void afterPropertiesSet() throws Exception {
if (Strings.isEmpty(dir)) {
if (new File("./target").isDirectory() && new File("./src/").isDirectory()) {
dir = "./target/upload";
} else {
dir = "./upload/";
}
}
File file = new File(dir);
if (!file.exists()) {
Files.createDirectories(file.toPath());
}
if (!file.isDirectory()) {
throw new IllegalStateException("Path '" + dir + "' must be a directory");
}
if (!file.canWrite()) {
throw new IllegalStateException("Path '" + dir + "' must be writable");
}
dir = Paths.suffixWithoutSlash(dir);
dirPath = java.nio.file.Paths.get(dir).toAbsolutePath().normalize();
if(Strings.isEmpty(signSecret)) {
throw new IllegalStateException("The property 'sfs.signSecret' must be configured");
}
if(signSecret.equals(DEFAULT_SIGN_SECRET)) {
log.error("\n\n" +
"!!!WARNING!!!\n" +
"*********************************************************************************\n" +
" Don't use default sign secret, change it by config property 'sfs.signSecret'\n" +
"*********************************************************************************\n");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy