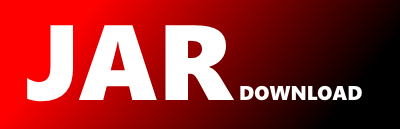
jmms.plugins.SwaggerDoc Maven / Gradle / Ivy
/*
* Copyright 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jmms.plugins;
import leap.lang.Strings;
import leap.lang.Try;
import leap.lang.io.*;
import leap.lang.json.JsonObject;
import leap.lang.logging.Log;
import leap.lang.logging.LogFactory;
import leap.lang.resource.Resource;
import leap.lang.resource.ResourceSet;
import leap.lang.resource.Resources;
import java.io.File;
import java.nio.file.*;
import java.nio.file.Files;
import java.nio.file.Paths;
public class SwaggerDoc {
private static final Log log = LogFactory.get(SwaggerDoc.class);
private FileChangeMonitor monitor;
private volatile JsonObject swagger;
private final String dir;
public SwaggerDoc() {
this("./doc/");
}
public SwaggerDoc(String dir) {
this.dir = leap.lang.path.Paths.suffixWithSlash(dir);
}
public void init() {
File dir1 = new File(dir + "_i18n/");
File dir2 = new File(dir + "_templates/");
if(dir1.exists() || dir2.exists()) {
if(null == monitor) {
monitor = new FileChangeMonitor(2000);
}
FileChangeListener listener = new FileChangeListenerAdaptor2() {
@Override
protected void onChanged(FileChangeObserver observer, FileChangeEvent event, File fileOrDirectory) {
log.info("Doc changed, re-generate it");
gen();
}
};
if(dir1.exists()) {
monitor.addObserver(new FileChangeObserver(dir1, listener));
}
if(dir2.exists()) {
monitor.addObserver(new FileChangeObserver(dir2, listener));
}
Try.throwUnchecked(() -> monitor.start());
log.info("Watching doc changes...");
}
Path doc = Paths.get(dir);
String root = Templates.ROOT_CLASS_PATH + "gitbook/";
String loc = "classpath:" + root + "**/*.*";
ResourceSet rs = Resources.scan(loc);
log.info("Scan {} gitbook files from '{}'", rs.size(), loc);
for(Resource r : rs) {
if(r.exists()) {
Path path = doc.resolve(Strings.removeStart(r.getClasspath(), root));
File file = path.toFile();
if(!file.exists()) {
Try.throwUnchecked(() -> Files.createDirectories(path.getParent()));
log.info("Create gitbook file : {}", path.normalize());
IO.writeString(path.toFile(), r.getContent());
}
}
}
}
public void close() {
if(null != monitor) {
Try.catchAll(() -> monitor.stop());
}
}
public void gen(JsonObject swagger) {
this.swagger = swagger;
gen();
}
private void gen() {
SwaggerDocGen gen = new SwaggerDocGen(dir, "zh");
gen.execute(swagger);
log.info("Swagger doc generated");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy