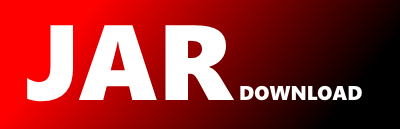
jmms.testing.SimpleTestContext Maven / Gradle / Ivy
/*
* Copyright 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jmms.testing;
import jmms.core.Api;
import jmms.engine.js.JsEngine;
import jmms.testing.fake.FakeExpr;
import jmms.testing.fake.FakeOptions;
import jmms.testing.fake.Faker;
import leap.lang.Args;
import leap.lang.Strings;
import leap.lang.json.JSON;
import leap.orm.mapping.Mappings;
import javax.script.ScriptException;
import java.util.*;
import java.util.function.BiFunction;
public class SimpleTestContext implements TestContext {
protected final Api api;
protected final JsEngine engine;
protected final Faker faker;
protected Map variables = new HashMap<>();
protected TestSuite currentSuite;
protected TestCase currentTest;
protected TestStep previousStep;
protected TestStep currentStep;
protected Object execDetails;
public SimpleTestContext(Api api, JsEngine engine, Faker faker) {
this.api = api;
this.engine = engine;
this.faker = faker;
}
@Override
public Map getVariables() {
return variables;
}
@Override
public void clearVariables() {
if(null != variables) {
variables.clear();
}
}
@Override
public void setVariables(Map variables) {
Args.notNull(variables);
this.variables = variables;
}
@Override
public TestSuite getCurrentSuite() {
return currentSuite;
}
@Override
public void setCurrentSuite(TestSuite suite) {
currentSuite = suite;
}
@Override
public TestCase getCurrentTest() {
return currentTest;
}
@Override
public void setCurrentTest(TestCase test) {
currentTest = test;
}
@Override
public TestStep getPreviousStep() {
return previousStep;
}
@Override
public void setPreviousStep(TestStep previousStep) {
this.previousStep = previousStep;
}
@Override
public TestStep getCurrentStep() {
return currentStep;
}
@Override
public void setCurrentStep(TestStep step) {
currentStep = step;
}
@Override
public Object getExecDetails() {
return execDetails;
}
@Override
public void setExecDetails(Object details) {
this.execDetails = details;
}
@Override
public T resolve(Object value) {
if(null == value) {
return null;
}
if(value instanceof Map) {
return (T)resolveMap((Map)value);
}
if(value instanceof List) {
return (T)resolveList((List)value);
}
if(value instanceof Object[]) {
return (T)resolveArray((Object[])value);
}
if(!(value instanceof String)) {
return (T)value;
}
String s = (String)value;
if(Strings.startsWithIgnoreCase(s, EVAL_PREFIX)) {
String expr = s.substring(EVAL_PREFIX.length());
try {
Object resolved = engine.eval(expr, getJavascriptVars());
if(null == resolved) {
throw new IllegalStateException("Can't resolve value '" + s + "'");
}
return (T)resolved;
}catch (ScriptException e) {
throw new IllegalStateException("Error eval \"" + expr + "\", " + e.getMessage(), e);
}
}
if(Strings.startsWithIgnoreCase(s, FAKE_PREFIX)) {
String expr = s.substring(FAKE_PREFIX.length());
Object sample;
if(FakeExpr.isExpr(expr)) {
sample = faker.eval(expr);
}else {
int left = expr.indexOf('(');
if(left > 0) {
String key = expr.substring(0, left);
String json = expr.substring(left + 1, expr.length() - 1);
if(!(json.startsWith("{") && json.endsWith("}"))) {
throw new IllegalStateException("Invalid fake expr [" + expr + "]");
}
try {
FakeOptions opts = JSON.decode(json, FakeOptions.class);
sample = faker.fake(key, opts);
}catch (Exception e) {
throw new IllegalStateException("Invalid fake expr [" + expr + "], " + e.getMessage(), e);
}
}else {
sample = faker.fake(expr);
}
}
return (T)sample;
}
return (T)s;
}
protected Map getJavascriptVars() {
Map vars = new HashMap<>(variables);
vars.put("$id", new IdFunc());
return vars;
}
protected Map resolveMap(Map map) {
Map resolved = new LinkedHashMap<>();
map.forEach((name,value) -> {
resolved.put(name, resolve(value));
});
return resolved;
}
protected List resolveList(List list) {
List resolved = new ArrayList();
list.forEach(item -> {
resolved.add(resolve(item));
});
return resolved;
}
protected Object[] resolveArray(Object[] a) {
Object[] resolved = new Object[a.length];
for(int i=0;i {
@Override
public Object apply(String name, Map map) {
Object id = map.get("$id");
if(null != id) {
return id;
}
return Mappings.getId(api.getOrmContext().getMetadata().getEntityMapping(name), map);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy