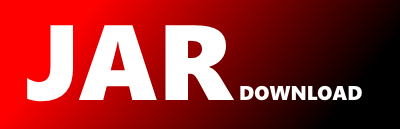
jmms.testing.SimpleTestSuite Maven / Gradle / Ivy
/*
* Copyright 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jmms.testing;
import jmms.core.Api;
import jmms.core.model.MetaTestSuite;
import leap.lang.Buildable;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
import java.util.function.Predicate;
public class SimpleTestSuite implements TestSuite {
private final Api api;
private final MetaTestSuite meta;
private final TestAction[] before;
private final TestCase[] tests;
private final TestSuite[] suites;
private final TestAction[] after;
public SimpleTestSuite(Api api, MetaTestSuite meta, TestAction[] before, TestCase[] tests, TestSuite[] suites, TestAction[] after) {
this.api = api;
this.meta = meta;
this.before = before;
this.tests = tests;
this.suites = suites;
this.after = after;
}
@Override
public Api getApi() {
return api;
}
@Override
public MetaTestSuite getMeta() {
return meta;
}
@Override
public TestAction[] getBefore() {
return before;
}
@Override
public TestCase[] getTests() {
return tests;
}
@Override
public TestSuite[] getSuites() {
return suites;
}
@Override
public TestAction[] getAfter() {
return after;
}
@Override
public TestAndSuite findTest(String id) {
return findTest(t -> id.equals(t.getMeta().getId()));
}
@Override
public TestAndSuite findTest(Predicate condition) {
return findTest(this, condition);
}
protected TestAndSuite findTest(TestSuite suite, Predicate condition) {
for(TestCase test : suite.getTests()) {
if(condition.test(test)) {
return new TestAndSuite(test, suite);
}
}
for(TestSuite child : suite.getSuites()) {
TestAndSuite test = findTest(child, condition);
if(null != test) {
return test;
}
}
return null;
}
@Override
public void iterate(Consumer func) {
iterate(this, func);
}
protected void iterate(TestSuite suite, Consumer func) {
for(TestCase test : suite.getTests()) {
func.accept(test);
}
for(TestSuite child : suite.getSuites()) {
iterate(child, func);
}
}
public static final class Builder implements Buildable {
private final Api api;
private final MetaTestSuite meta;
private final List before = new ArrayList<>();
private final List tests = new ArrayList<>();
private final List suites = new ArrayList<>();
private final List after = new ArrayList<>();
public Builder(TestTarget target, MetaTestSuite meta) {
this.api = target.getApi();
this.meta = meta;
}
public List getTests() {
return tests;
}
public List getSuites() {
return suites;
}
public void addTest(TestCase test) {
tests.add(test);
}
public void addSuite(TestSuite suite) {
suites.add(suite);
}
public void addBefore(TestAction action) {
before.add(action);
}
public void addAfter(TestAction action) {
after.add(action);
}
@Override
public SimpleTestSuite build() {
return new SimpleTestSuite(api, meta,
before.toArray(new TestAction[0]),
tests.toArray(new TestCase[0]), suites.toArray(new TestSuite[0]),
after.toArray(new TestAction[0]));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy