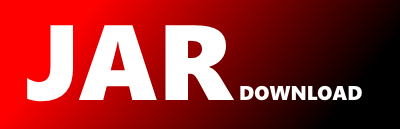
jmms.testing.TestContext Maven / Gradle / Ivy
/*
* Copyright 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jmms.testing;
import java.util.LinkedHashMap;
import java.util.Map;
public interface TestContext {
String EVAL_PREFIX = "eval:";
String FAKE_PREFIX = "fake:";
/**
* Returns current variables.
*/
Map getVariables();
/**
* Returns nested variables.
*/
default Map getVariables(String name) {
Map nested = (Map) getVariables().get(name);
if(null == nested) {
nested = new LinkedHashMap<>();
setVariable(name, nested);
}
return nested;
}
/**
* Clears the variables.
*/
void clearVariables();
/**
* Sets variables
*/
void setVariables(Map vars);
/**
* Set a variable.
*/
default void setVariable(String name, Object value) {
getVariables().put(name, value);
}
/**
* Set nested variable
*/
default void setVariable(String root, String name, Object value) {
getVariables(root).put(name, value);
}
/**
* Returns current running suite.
*/
TestSuite getCurrentSuite();
/**
* Sets current running suite.
*/
void setCurrentSuite(TestSuite suite);
/**
* Returns true if context running for test currently.
*/
default boolean isRunningTest() {
return null != getCurrentStep();
}
/**
* Returns current running test.
*/
TestCase getCurrentTest();
/**
* Sets current running test.
*/
void setCurrentTest(TestCase test);
/**
* Returns true if context running for step currently.
*/
default boolean isRunningStep() {
return null != getCurrentStep();
}
/**
* Returns previous step.
*/
TestStep getPreviousStep();
/**
* Sets previous step.
*/
void setPreviousStep(TestStep step);
/**
* Returns current running test step.
*/
TestStep getCurrentStep();
/**
* Sets current running step.
*/
void setCurrentStep(TestStep step);
/**
* Returns the last execution details.
*/
Object getExecDetails();
/**
* Sets the last execution details.
*/
void setExecDetails(Object details);
/**
* removes last execution details from context and returns it.
*/
default Object removeExecDetails() {
Object d = getExecDetails();
setExecDetails(null);
return d;
}
/**
* Resolves the value if the value is a string expr.
*
* @throws IllegalStateException if the resolved value is null.
*/
T resolve(Object value) throws IllegalStateException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy