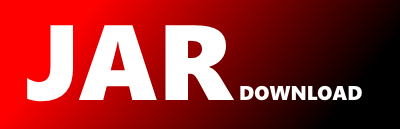
jmms.testing.asserts.Assert Maven / Gradle / Ivy
/*
* Copyright 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jmms.testing.asserts;
import leap.lang.Objects2;
import leap.lang.Strings;
import leap.lang.convert.Converts;
import java.util.*;
final class Assert {
public void equal(Object actual, Object expected) {
equals(actual, expected);
}
public void equals(Object actual, Object expected) {
if(!doEquals(actual, expected)) {
throw new AssertException("Expected [" + expected + "], Actual [" + actual + "]");
}
}
public void lt(Number toCheck, Number toBeAbove) {
if(toCheck.doubleValue() >= toBeAbove.doubleValue()) {
throw new AssertException("Number " + toCheck + " should < " + toBeAbove);
}
}
public void le(Number toCheck, Number toBeAbove) {
if(toCheck.doubleValue() > toBeAbove.doubleValue()) {
throw new AssertException("Number " + toCheck + " should <= " + toBeAbove);
}
}
public void gt(Number toCheck, Number toBeAbove) {
if(toCheck.doubleValue() <= toBeAbove.doubleValue()) {
throw new AssertException("Number " + toCheck + " should > " + toBeAbove);
}
}
public void ge(Number toCheck, Number toBeAtLeast) {
if(toCheck.doubleValue() < toBeAtLeast.doubleValue()) {
throw new AssertException("Number " + toCheck + " should >= " + toBeAtLeast);
}
}
/**
* Same as {@link #lt(Number, Number)}.
*/
public void isBelow(Number toCheck, Number toBeBelow){
lt(toCheck, toBeBelow);
}
/**
* Same as {@link #le(Number, Number)}.
*/
public void isAtMost(Number toCheck, Number toBeAtMost) {
le(toCheck, toBeAtMost);
}
/**
* Same as {@link #gt(Number, Number)}.
*/
public void isAbove(Number toCheck, Number toBeAbove) {
gt(toCheck, toBeAbove);
}
/**
* Same as {@link #ge(Number, Number)}.
*/
public void isAtLeast(Number toCheck, Number toBeAtLeast) {
ge(toCheck, toBeAtLeast);
}
public void isNotEmpty(Object value, String info) {
if(Strings.isEmpty(info)) {
info = "Value";
}
if(Objects2.isEmpty(value)) {
throw new AssertException(info + " should not be empty");
}
}
/**
* Asserts that a subset of properties in an object
*/
public void include(Map haystack, Map needle) {
include("", haystack, needle);
}
private void include(String prefix, Map haystack, Map needle) {
needle.forEach((name, expected) -> {
String fullName = prefix + name;
if(null == expected) {
return;
}
if(!haystack.containsKey(name)) {
throw new AssertException("Expected include property '" + fullName + "', Expected [" + expected + "], Actual not exists");
}
Object actual = haystack.get(name);
includeEquals(fullName, expected, actual);
});
}
private void includeEquals(String fullName, Object expected, Object actual) {
//Map
if(actual instanceof Map && expected instanceof Map) {
include(fullName + ".", (Map)actual, (Map)expected);
return;
}
//List
if(actual instanceof List && expected instanceof List) {
List expectedList = (List)expected;
List actualList = (List)actual;
if(expectedList.size() != actualList.size()) {
throw new AssertException("List '" + fullName + "' not equals, Expected size " + expectedList.size() + ", Actual " + actualList.size());
}
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy