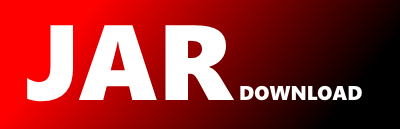
jmms.testing.fake.FakeMethods Maven / Gradle / Ivy
/*
* Copyright 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jmms.testing.fake;
import leap.lang.json.JSON;
import leap.lang.reflect.Reflection;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Map;
class FakeMethods {
private final Map map = new HashMap<>();
FakeMethods(Object faker) {
add(faker);
}
void add(Object faker) {
for(Method method : faker.getClass().getMethods()) {
if(null != method.getReturnType() && method.getParameters().length == 1) {
Class> type = method.getParameterTypes()[0];
if(FakeOptions.class.isAssignableFrom(type)) {
String name = method.getName();
if(name.startsWith("_")) {
name = name.substring(1);
}
map.put(name, new FakeMethod(faker, method, type));
}
}
}
}
FakeMethod get(String name) {
return map.get(name);
}
static class FakeMethod {
private final Object faker;
private final Method method;
private final Class> optsType;
private final FakeOptions defaultOpts;
public FakeMethod(Object faker, Method method, Class> optsType) {
this.faker = faker;
this.method = method;
this.optsType = optsType;
this.defaultOpts = optsType.equals(FakeOptions.class) ? FakeOptions.NO_OPTS : (FakeOptions)Reflection.newInstance(optsType);
}
Class> getOptionsType() {
return optsType;
}
Object eval(FakeOptions opts) {
if(null == opts) {
opts = defaultOpts;
}
if(!opts.getClass().equals(optsType)) {
opts = (FakeOptions)JSON.decode(JSON.encode(opts), optsType);
}
try {
Object v = method.invoke(faker, opts);
if(null == v || !(v instanceof String)) {
return v;
}
String s = (String) method.invoke(faker, opts);
if(opts.isLower()) {
s = s.toLowerCase();
}else if(opts.isUpper()) {
s = s.toUpperCase();
}
return s;
}catch (Exception e) {
throw new IllegalStateException(e.getMessage(), e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy