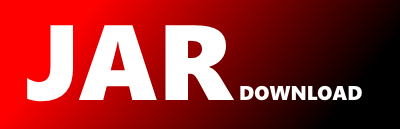
jmms.testing.server.TestController Maven / Gradle / Ivy
/*
* Copyright 2018 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package jmms.testing.server;
import jmms.core.model.MetaFakeCategory;
import jmms.core.model.MetaTestSuite;
import jmms.testing.TestAndSuite;
import jmms.testing.TestEngine;
import jmms.testing.TestResult;
import jmms.testing.TestingRuntime;
import jmms.testing.fake.Faker;
import leap.core.annotation.Inject;
import leap.core.security.annotation.AllowAnonymous;
import leap.core.validation.annotations.Required;
import leap.lang.New;
import leap.lang.Strings;
import leap.lang.util.ShortUUID;
import leap.web.annotation.Path;
import leap.web.annotation.http.DELETE;
import leap.web.annotation.http.GET;
import leap.web.annotation.http.POST;
import leap.web.api.mvc.ApiController;
import leap.web.api.mvc.ApiResponse;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
@Path(TestController.PATH)
@AllowAnonymous
public class TestController extends ApiController {
static final String PATH = "/$test";
private final TestEngine engine;
private final TestingRuntime runtime;
private final Map contexts = new ConcurrentHashMap<>();
private @Inject Faker faker;
public TestController(TestEngine engine, TestingRuntime runtime) {
this.engine = engine;
this.runtime = runtime;
}
@GET("/")
public ApiResponse meta(Boolean crud) {
MetaTestSuite meta;
if(null != crud && !crud) {
meta = runtime.getTestSuite().getMeta().filter((t) -> !t.isCrud());
}else {
meta = runtime.getTestSuite().getMeta();
}
return ApiResponse.of(meta);
}
@POST("/start")
public ApiResponse start() {
//todo: allow multi contexts.
contexts.clear();
String cid = ShortUUID.randomUUID();
contexts.put(cid, new RunContext());
Map map = New.hashMap("cid", cid);
return ApiResponse.of(map);
}
@POST("/run")
public ApiResponse run(String cid, @Required String tid) {
RunContext context;
if(!Strings.isEmpty(cid)) {
context = contexts.get(cid);
if (null == context) {
return ApiResponse.badRequest("Invalid context id '" + cid + "'");
}
}else {
context = new RunContext();
}
TestAndSuite test = runtime.getTestSuite().findTest(tid);
if(null == test) {
return ApiResponse.notFound("Test case '" + tid + "' not found");
}
if(context.running) {
return ApiResponse.badRequest("Concurrent running tests in the same context is not allowed!");
}
try {
context.running = true;
return ApiResponse.of(engine.runTestCase(test));
}finally{
context.running = false;
}
}
@POST("/reload")
public ApiResponse reload(boolean drop) {
if(drop) {
engine.dropDb(runtime.getApi(), false);
}
engine.reload(runtime.getApi());
return ApiResponse.ok();
}
@DELETE("/db/drop")
public ApiResponse dropDb() {
engine.dropDb(runtime.getApi(), true);
return ApiResponse.ok();
}
@DELETE("/db")
public ApiResponse cleanDb() {
return ApiResponse.of(engine.cleanDb(runtime.getApi()));
}
@POST("/db")
public ApiResponse genDb(int rows) {
if(rows == 0) {
rows = 1;
}
return ApiResponse.of(engine.genDb(runtime.getApi(), rows));
}
@GET("/fake")
public ApiResponse
© 2015 - 2025 Weber Informatics LLC | Privacy Policy