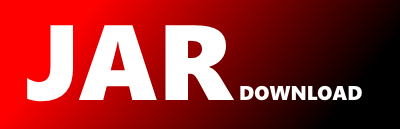
leap.lang.Predicates Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package leap.lang;
import java.io.Serializable;
import java.lang.annotation.Annotation;
import java.util.Map.Entry;
import java.util.function.Predicate;
import leap.lang.annotation.Nullable;
//some codes copy from google guava library 15.0-SNAPSHOT,under Apache License 2.0
public class Predicates {
/**
* Returns a predicate that evaluates to {@code true} if the object reference being tested is null.
*/
public static Predicate isNull() {
return ObjectPredicate.IS_NULL.withNarrowedType();
}
public static Predicate nameEquals(final String name){
return new Predicate() {
public boolean test(T object) {
return Strings.equals(object.getName(), name);
}
};
}
public static Predicate nameEqualsIgnoreCase(final String name){
return new Predicate() {
public boolean test(T object) {
return Strings.equalsIgnoreCase(object.getName(),name);
}
};
}
public static Predicate> entryKeyEquals(final K key){
return new Predicate>() {
public boolean test(Entry entry) {
return entry.getKey().equals(key);
}
};
}
public static Predicate> entryKeyEqualsIgnoreCase(final String key){
return new Predicate>() {
public boolean test(Entry entry) {
return entry.getKey().equalsIgnoreCase(key);
}
};
}
/**
* Returns a predicate that evaluates to {@code true} if the object being tested is an instance of the given class.
* If the object being tested is {@code null} this predicate evaluates to {@code false}.
*
*
* If you want to filter an {@code Iterable} to narrow its type, consider using
* {@link com.google.common.collect.Iterables#filter(Iterable, Class)} in preference.
*
*
* Warning: contrary to the typical assumptions about predicates (as documented at {@link Predicate#apply}),
* the returned predicate may not be consistent with equals. For example, {@code instanceOf(ArrayList.class)}
* will yield different results for the two equal instances {@code Lists.newArrayList(1)} and
* {@code Arrays.asList(1)}.
*/
public static Predicate
© 2015 - 2025 Weber Informatics LLC | Privacy Policy